Question
Problem 9: A simple interactive program 18 points total; individual-only This problem provides additional practice with writing static methods, and it also gives you a
Problem 9: A simple interactive program
18 points total; individual-only
This problem provides additional practice with writing static methods, and it also gives you a chance to write a simple program with repeated user interactions. In particular, the program will allow the user to perform a variety of operations on a set of three integers.
Getting started Begin by downloading the following file: SimpleStats.java
/* * Problem Set 1 * * A simple interactive program that performs operations * on a set of three integers. */
import java.util.*;
public class SimpleStats {
public static void printMenu() { System.out.println("(0) Enter new numbers"); System.out.println("(1) Find the largest"); System.out.println("(2) Compute the sum"); System.out.println("(3) Compute the range (largest - smallest)"); System.out.println("(4) Compute the average"); System.out.println("(5) Print the numbers in ascending order"); System.out.println("(6) Quit"); System.out.println(); } /*** PUT YOUR SEPARATE HELPER METHODS FOR OPTIONS 1-5 HERE ***/ public static void main(String[] args) { Scanner scan = new Scanner(System.in);
// the three integers int n1 = 2; int n2 = 4; int n3 = 6;
boolean more_input = true; do { System.out.print("The current numbers are: "); System.out.println(n1 + " " + n2 + " " + n3); printMenu(); System.out.print("Enter your choice: "); int choice = scan.nextInt(); /* * Expand this conditional statement to process choices 1-5. * Make sure to follow the guidelines in the assignment for * doing so. */ if (choice == 0) { System.out.print("Enter three new numbers: "); n1 = scan.nextInt(); n2 = scan.nextInt(); n3 = scan.nextInt(); } else if (choice == 6) { more_input = false; } else { System.out.println("Invalid choice. Please try again."); } System.out.println(); } while (more_input); System.out.println("Have a nice day!"); } }
It provides a skeleton for your program.
In particular, weve given you the beginnings of the main() method, including the user-interaction loop. main() also declares the variables n1, n2, and n3 for the three integers that the program will process, and it provides initial values for those variables.
Compile and run the program, and you should see the following output:
The current numbers are: 2 4 6 (0) Enter new numbers (1) Find the largest (2) Compute the sum (3) Compute the range (largest - smallest) (4) Compute the average (5) Print the numbers in ascending order (6) Quit Enter your choice:
At the moment, the only supported options are options 0 and 6, so feel free to try them out!
Your tasks Start by reading over the starter code that weve given you. Make sure that you understand it.
Then add support for menu options 1-5 so that they perform the specified operations on the three numbers.
Important notes:
-
You must implement a separate helper method for each option. In addition, you should add appropriate code to main to handle each option calling the appropriate helper method, and taking whatever additional steps are needed.
-
Each helper method must take the three numbers as parameters. The names of the methods are up to you, but you should of course pick names that describe what the methods are supposed to do.
-
The helper methods for options 1-4 must not do any printing. Rather, they should return the appropriate result, and the printing of the result should be done in main. You should select the appropriate return type for each method, based on the value it is supposed to compute. Options 1-3 should produce an integer, while option 4 should produce a floating-point number.
-
Your helper method for option 5 should do its own printing printing the numbers in ascending order, separated by spaces. This helper method should not return a value.
-
There are one or more cases in which one of the helper methods can be simplified by having it call one of the other helper methods to obtain a partial result. For full credit, you should do this whenever possible to avoid duplicating code unnecessarily.
-
You may not use methods from any libraries other than the built-in Math library, although using Math is not required. Refer to the Math API for details.
A sample run can be found here. Please match the formatting of the results in that file as closely as possible.
Sample output:
The current numbers are: 2 4 6 (0) Enter new numbers (1) Find the largest (2) Compute the sum (3) Compute the range (largest - smallest) (4) Compute the average (5) Print the numbers in ascending order (6) Quit Enter your choice: 3 The range of the numbers is 4 The current numbers are: 2 4 6 (0) Enter new numbers (1) Find the largest (2) Compute the sum (3) Compute the range (largest - smallest) (4) Compute the average (5) Print the numbers in ascending order (6) Quit Enter your choice: 4 The average of the numbers is 4.0 The current numbers are: 2 4 6 (0) Enter new numbers (1) Find the largest (2) Compute the sum (3) Compute the range (largest - smallest) (4) Compute the average (5) Print the numbers in ascending order (6) Quit Enter your choice: 0 Enter three new numbers: 15 7 21 The current numbers are: 15 7 21 (0) Enter new numbers (1) Find the largest (2) Compute the sum (3) Compute the range (largest - smallest) (4) Compute the average (5) Print the numbers in ascending order (6) Quit Enter your choice: 1 The largest of the numbers is 21 The current numbers are: 15 7 21 (0) Enter new numbers (1) Find the largest (2) Compute the sum (3) Compute the range (largest - smallest) (4) Compute the average (5) Print the numbers in ascending order (6) Quit Enter your choice: 2 The sum of the numbers is 43 The current numbers are: 15 7 21 (0) Enter new numbers (1) Find the largest (2) Compute the sum (3) Compute the range (largest - smallest) (4) Compute the average (5) Print the numbers in ascending order (6) Quit Enter your choice: 3 The range of the numbers is 14 The current numbers are: 15 7 21 (0) Enter new numbers (1) Find the largest (2) Compute the sum (3) Compute the range (largest - smallest) (4) Compute the average (5) Print the numbers in ascending order (6) Quit Enter your choice: 4 The average of the numbers is 14.333333333333334 The current numbers are: 15 7 21 (0) Enter new numbers (1) Find the largest (2) Compute the sum (3) Compute the range (largest - smallest) (4) Compute the average (5) Print the numbers in ascending order (6) Quit Enter your choice: 5 In order, the numbers are: 7 15 21 The current numbers are: 15 7 21 (0) Enter new numbers (1) Find the largest (2) Compute the sum (3) Compute the range (largest - smallest) (4) Compute the average (5) Print the numbers in ascending order (6) Quit Enter your choice: 6 Have a nice day!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
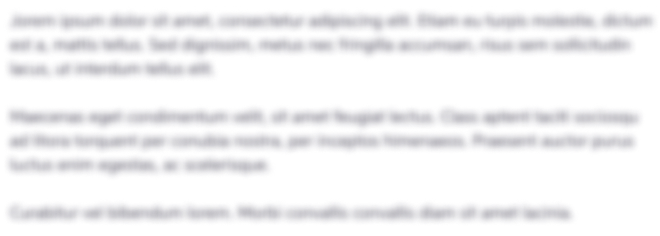
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started