Question
Problem Description: A program is needed that will simulate a game of chance, where the player bets on the outcome of a Snail Race. The
Problem Description: A program is needed that will simulate a game of chance, where the player bets on the outcome of a Snail Race. The player will be allowed to repeatedly select one of the following choices from the menu as long as the player does not choose to quit the game or the player has not lost all of his/her money through choosing snails that did not win a race. Choose a snail upon which to place a bet Choose to just watch a race, but not place a bet on a snail Choose to leave the races (quit the game) When the player selects a snail, the game action will proceed as follows: provide the player the ability to enter an amount of money that he/she wants to wager on the snail winning the race simulate the racing of the snails through the use of the random number generator to determine which of the snails wins the race and display which snail won the race determine whether the players chosen snail won the race and either add to the players money by 2 times the bet if the players chosen snail won or subtract from the players money the amount of the bet if the players chosen snail did not win the race display the players current amount of remaining money re-display the game menu to the player and allow the game to begin again with the remaining amount of players money Should the player select the option to Just Watch, the game action will proceed as follows: simulate the racing of the snails through the use of the random number generator to determine which of the snails wins the race display the players current amount of remaining money re-display the game menu to the player and allow the game to begin again with the remaining amount of players money Should the player select the option to Leave the races or the player has lost all of his money in the game, the game action will proceed as follows: drop out of the game loop following the game loop determine whether the player has any money left and output one of the following messages before ending the program: o if player has no money left output a message that wishes the player better luck next time and asks him/her to come play again soon o if player has more money left than starting amount output a message that congratulations the player on the winnings and asks him/her to come play again soon. Page 2 of 5 o if player has some money left output a message that thanks the player for playing and asks him/her to come play again soon Instructions: Using the knowledge that you have gained about methods and the use of parameters and returned values, you are to code a simple game program called SnailRaces. The logic design of this program requires that several methods be used to perform the game actions of allowing the user to select a snail, handling a bet entry and simulating the race along with determining the winner of a race and calculating the monetary winnings or losses. To assist you in visualizing the major tasks in this game, the following hierarchical chart has been provided. The functional description of each method is given below. Method: main() The main method will operate as a main controller or driver of each of these game actions. It will contain the master game loop. Method: selectSnail() This value-returning method will display the games menu. This method will insure that the player selects only a valid choice from the menu. (HINT: Input validation required.) The method will return the players validated menu choice back to the main() method. This method does not require any parameter values to do its work. Method: placeBet() This value-returning method will need two pieces of data. This first data item will be the players choice of a snail (the menu choice). The second data item will be the players amount of remaining money with which he/she will be able to place a bet. The method may make some comment about the snail that was chosen by the player, but it must output a prompt for the player to enter the amount of money that he/she wants to bet on the particular snail number that was chosen from the menu. The prompt might be something like How much money do you want to place on snails name, where snails name is replaced with one of the actual snail names that youve chosen. selectSnail method get users snail choice placeBet method place bet on the race runRace method run the race and determine amount won or lost main method game loop coded here Page 3 of 5 The method should accept keyboard input for the amount of the bet. It should also validate that the entered amount is not zero or less and that it is not more than the amount of money that remains for the player (HINT: Data validation required). After a valid amount is entered for the bet, then the bet amount is returned to the main() method. Method: runRace() This method will simulate a race of the three snails and determine the winner of the race by creating a Random object and using an appropriate method of that class to generate a number that serves to identify the winning snail for the race. The returned random number will need to be limited to between 1 and n (the number of snails in the race). (Hint: review the nextInt() method of the Random class, see Chapter 4, Section 4.11, pgs. 233 234, and Chapter 4 powerpoint slides). To aid in simulating this race, the method will output an announcers call of the race something like: And the snails are off, look at them GO!!!! The winner is ______ Where the randomly generated number will be converted to the associated snails name. This method will need to receive via a parameter the players snail choice (menu choice). After the winning snail number has been produced, this method must determine whether the players selected snail was the winning snail (Hint: players snail number == winning snail number). This method will also need to receive via a parameter the players bet amount, because if the player chose the winning snail, then the method will need to calculate two (2) times the bet amount as the race winnings for the player. However, if the player did not choose the winning snail, then the method must calculate the race lost, which can be calculated as (negative 1) times the bet amount. This method will need to return the positive or negative race winnings amount back to the main method, where it can be used to adjust through addition of the returned amount the players remaining money. After completing the coding logic for this program, compile, execute and test the program to see if it will provide a functional game that can be played. Then play the game to see if you come home from the snail races as a winner or a loser.
Example Execution:
Welcome to the snail races!
You have 200.00 dollars.
Races Menu
1) Bet on Speedy
2) Bet on Zippy
3) Bet on Slick
4) Just watch, no bet
0) Leave the races
Select a snail: 1 Snail Speedy is a good choice!
How much would you like to bet on Speedy
50.00
And the snails are off Look at them GO!!!
The winner is Slick You lose -50.00 dollars.
You have 150.00 dollars.
Races Menu
1) Bet on Speedy
2) Bet on Zippy
3) Bet on Slick
4) Just watch, no bet
0) Leave the races
Select a snail:
4 And the snails are off Look at them GO!!!
The winner is Speedy Program input is highlighted in yellow.
NOTE: Not all possible paths through this game program are illustrated with this example execution. Page 5 of 5 You have 150.00 dollars. Races Menu 1) Bet on Speedy 2) Bet on Zippy 3) Bet on Slick 4) Just watch, no bet 0) Leave the races Select a snail: 2 Snail Zippy is a good choice! How much would you like to bet on Zippy 50.00 And the snails are off Look at them GO!!! The winner is Speedy You lose -50.00 dollars. You have 100.00 dollars. Races Menu 1) Bet on Speedy 2) Bet on Zippy 3) Bet on Slick 4) Just watch, no bet 0) Leave the races Select a snail: 0 Thanks for playing - come back again soon!!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
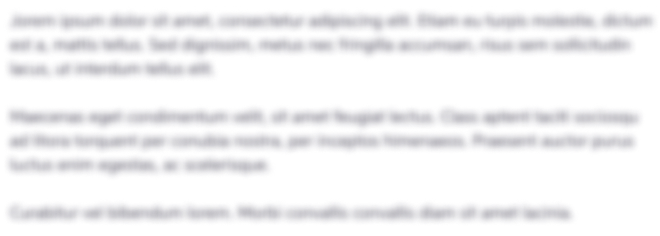
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started