Question
Problem: Fill in the missing code in Problem4.c (in the designated areas). All the files are located below. Will rate positively if complete. Take screenshot
Problem: Fill in the missing code in Problem4.c (in the designated areas). All the files are located below. Will rate positively if complete.
Take screenshot of compiled output once complete. (which should look similar to the sample one provided)
This problem requires flipping a biased, 2-sided coin where bias [ 0.0,1.0 ] is the fraction of the time the result of a coin-flip is a HEAD and the(1.0-bias) fraction of the time the result is a TAIL. Model the two possible results of a coin-flip as follows
typedef enum { HEAD=1,TAIL=2 } FLIP;
Let the user specify the bias, a stack capacity, and a number of trials at run-time. Using those three values, run one trial trials times, accumulating the total of all flips (flipsTrials) and the maximum of the maxSize over all trials (maxSizeTrials). When all trials are completed, output the following simple statistics
Average flips = XXXXXXXX.X, maximum size = XXXXXXXXX
The steps for running one trial are (1) construct the coin-flips stack using the user-specified capacity; (2) push one HEAD onto the coin-flips stack; (3) push one TAIL onto the coin-flips stack; (4) initialize flips and maxSize appropriately; (5) repeat the simple algorithm below; then (6) destruct the coin-flips stack. Keep track of the maximum value of GetSizeSTACK() (maxSize) and the number of times the simple algorithm flips a coin (flips) during the trial.
DO
flip := CoinFlip(bias)
flips := flips+1
IF ( flip == top-of-stack (TOS) element ) THEN
pop TOS from coin-flips stack
ELSE
push flip onto coin-flips stack
IF ( stack-size > maxSize ) THEN
maxSize = stack-size
END
END
WHILE ( NOT stack-is-empty )
//--------------------------------------------------------------
// STACK ADT Problem #4
// Problem4.c
//--------------------------------------------------------------
#include
#include
#include
#include "..\Random.h"
#include ".\STACK2.h"
typedef enum { HEAD=1,TAIL=2 } FLIP;
//--------------------------------------------------------------
int main()
//--------------------------------------------------------------
{
void RunOneTrial(const double bias,const int capacity,int *flips,int *maxSize);
double bias;
SetRandomSeed();
printf("bias? ");
while ( scanf("%lf",&bias) != EOF )
{
int trials,capacity;
int flipsTrials,maxSizeTrials;
int i;
printf("capacity? "); scanf("%d",&capacity);
Student provides missing code to run all the trials for the bias-capacity-trials instance
of the problem and accumulate and display the required statistics according to the following
format
Average flips = XXXXXXXX.X, maximum size = XXXXXXXXX
printf(" bias? ");
}
}
//--------------------------------------------------------------
void RunOneTrial(const double bias,const int capacity,int *flips,int *maxSize)
//--------------------------------------------------------------
{
FLIP CoinFlip(const double bias);
STACK coinflips;
ConstructSTACK(&coinflips,capacity);
Student provides missing code to "run one trial" (see requirements)
DestructSTACK(&coinflips);
}
//--------------------------------------------------------------
FLIP CoinFlip(const double bias)
//--------------------------------------------------------------
{
return( ((double) RandomInteger(1,100)/100
}
//----------------------------------------------------
// STACK2.h
//----------------------------------------------------
#ifndef STACK2_H
#define STACK2_H
#include
//==============================================================
// Data model definitions
//==============================================================
typedef struct STACK
{
int size;
int capacity;
int *elements;
} STACK;
//==============================================================
// Public member function prototypes
//==============================================================
void ConstructSTACK(STACK *stack,const int capacity);
void DestructSTACK(STACK *stack);
void PushSTACK(STACK *stack,const int element);
void PopSTACK(STACK *stack);
int PeekSTACK(const STACK *stack,const int offset);
int GetSizeSTACK(const STACK *stack);
int GetCapacitySTACK(const STACK *stack);
bool IsFullSTACK(const STACK *stack);
bool IsEmptySTACK(const STACK *stack);
int PeekSTACK2(const STACK *stack,const int offset,bool *exception);
int PeekSTACK3(const STACK *stack,const int offset);
//==============================================================
// Private utility member function prototypes
//==============================================================
// (none)
#endif
//--------------------------------------------------
// STACK2.c
//--------------------------------------------------
#include
#include
#include
#include ".\Stack2.h"
#include "..\ADTExceptions.h"
//--------------------------------------------------
void ConstructSTACK(STACK *stack,const int capacity)
//--------------------------------------------------
{
if ( capacity
stack->size = 0;
stack->capacity = capacity;
stack->elements = (int *) malloc(sizeof(int)*capacity);
if ( stack->elements == NULL ) RaiseADTException(MALLOC_ERROR);
}
//--------------------------------------------------
void DestructSTACK(STACK *stack)
//--------------------------------------------------
{
free(stack->elements);
}
//--------------------------------------------------
void PushSTACK(STACK *stack,const int element)
//--------------------------------------------------
{
if ( IsFullSTACK(stack) ) RaiseADTException(STACK_OVERFLOW);
stack->elements[stack->size] = element;
++stack->size;
}
//--------------------------------------------------
void PopSTACK(STACK *stack)
//--------------------------------------------------
{
if ( IsEmptySTACK(stack) ) RaiseADTException(STACK_UNDERFLOW);
stack->size--;
}
//--------------------------------------------------
int PeekSTACK(const STACK *stack,const int offset)
//--------------------------------------------------
{
if ( !((0
return( stack->elements[stack->size-(offset+1)] );
}
//--------------------------------------------------
int GetSizeSTACK(const STACK *stack)
//--------------------------------------------------
{
return( stack->size );
}
//--------------------------------------------------
int GetCapacitySTACK(const STACK *stack)
//--------------------------------------------------
{
return( stack->capacity );
}
//--------------------------------------------------
bool IsFullSTACK(const STACK *stack)
//--------------------------------------------------
{
return( (stack->size == stack->capacity) ? true : false );
}
//--------------------------------------------------
bool IsEmptySTACK(const STACK *stack)
//--------------------------------------------------
{
return( stack->size == 0 );
}
//--------------------------------------------------
int PeekSTACK2(const STACK *stack,const int offset,bool *exception)
//--------------------------------------------------
{
if ( !((0
{
*exception = true;
return( 0 );
}
*exception = false;
return( stack->elements[stack->size-(offset+1)] );
}
//--------------------------------------------------
int PeekSTACK3(const STACK *stack,const int offset)
//--------------------------------------------------
{
if ( !((0
{
fprintf(stderr,"\a Exception \"%s\" ",STACK_OFFSET_ERROR);
system("PAUSE");
exit( 1 );
}
return( stack->elements[stack->size-(offset+1)] );
}
//--------------------------------------------------------------
// ADTExceptions.h
//--------------------------------------------------------------
#ifndef ADTEXCEPTIONS_H
#define ADTEXCEPTIONS_H
// ADT exception definitions
#define MALLOC_ERROR "malloc() error"
#define DATE_ERROR "DATE error"
#define STACK_CAPACITY_ERROR "STACK capacity error"
#define STACK_UNDERFLOW "STACK underflow"
#define STACK_OVERFLOW "STACK overflow"
#define STACK_OFFSET_ERROR "STACK offset error"
// ADT exception-handler prototype
void RaiseADTException(char exception[]);
#endif
//--------------------------------------------------------------
// ADTExceptions.c
//--------------------------------------------------------------
#include
#include
#include
#include ".\ADTExceptions.h"
//--------------------------------------------------------------
void RaiseADTException(char exception[])
//--------------------------------------------------------------
{
fprintf(stderr,"\a Exception \"%s\" ",exception);
system("PAUSE");
exit( 1 );
}
//--------------------------------------------------
// Random.h
//--------------------------------------------------
#ifndef RANDOM_H
#define RANDOM_H
#include
// Initialize the "seed" used by the "rand()" function in
void SetRandomSeed(void);
// Return a uniformly and randomly chosen integer from [ LB,UB ].
int RandomInteger(int LB,int UB);
// Return a uniformly and randomly chosen real number chosen from [ 0.0,1.0 ).
double RandomReal(void);
// Return a uniformly and randomly chosen boolean from { false,true }.
bool RandomBoolean(void);
// Return a uniformly and randomly chosen character from characters[i] i in [ 0,(size-1) ].
char RandomCharacter(char characters[],int size);
#endif
//--------------------------------------------------
// Random.c
//--------------------------------------------------
#include
#include
#include
#include
#include ".\Random.h"
//--------------------------------------------------
void SetRandomSeed(void)
//--------------------------------------------------
{
srand(time(NULL));
}
//--------------------------------------------------
int RandomInteger(int LB,int UB)
//--------------------------------------------------
{
return( (int) (RandomReal()*(UB-LB+1)) + LB );
}
//--------------------------------------------------
double RandomReal()
//--------------------------------------------------
{
int R;
do
R = rand();
while ( R == RAND_MAX );
return( (double) R/RAND_MAX );
}
//--------------------------------------------------
bool RandomBoolean(void)
//--------------------------------------------------
{
return( RandomInteger(1,10000)
}
//--------------------------------------------------
char RandomCharacter(char characters[],int size)
//--------------------------------------------------
{
return( characters[RandomInteger(0,(size-1))] );
}
Sample Program Dialo EACOURSESICS13111Code\ADTs STACKProblem4.exe bias? .5 apacity? 100000 trials 1000 Average flips -184052.9. maximum size - 10047 bias? .5 apacity? 100000 trials? 1000 Average flips bias? .5 1216.7, maximum size 864 apacity? 100000 trials 1000 verage flips 15578.. maximum size - 5739 ias?.5 apacity? 100000 trials? 10000 Exception "STACK overflow Press any key to continue - . . _Step by Step Solution
There are 3 Steps involved in it
Step: 1
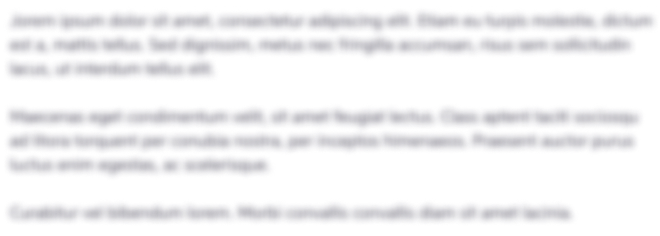
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started