Question
problem- I need help breaking down this specific program it's a banking code and I need to explain the below steps as mentioned in a
problem- I need help breaking down this specific program it's a banking code and I need to explain the below steps as mentioned in a 2 pg or so summary I just need help in the right direction please and thank you. link to original code is also provided as well as code below http://www.cppforschool.com/project/banking-system-project.html
1. Problem description (Should explain what you are trying to do and its importance)
2. Introduction (Should introduce your topic and applications, advantages / disadvantages)
3. Your analysis (Results)
4. Conclusion
Make sure to include and identify the OOP concepts in your project.
#include#include #include #include using namespace std; //*************************************************************** // CLASS USED IN PROJECT //**************************************************************** class account { int acno; char name[50]; int deposit; char type; public: void create_account(); //function to get data from user void show_account() const; //function to show data on screen void modify(); //function to add new data void dep(int); //function to accept amount and add to balance amount void draw(int); //function to accept amount and subtract from balance amount void report() const; //function to show data in tabular format int retacno() const; //function to return account number int retdeposit() const; //function to return balance amount char rettype() const; //function to return type of account }; //class ends here void account::create_account() { cout<<" Enter The account No. :"; cin>>acno; cout<<" Enter The Name of The account Holder : "; cin.ignore(); cin.getline(name,50); cout<<" Enter Type of The account (C/S) : "; cin>>type; type=toupper(type); cout<<" Enter The Initial amount(>=500 for Saving and >=1000 for current ) : "; cin>>deposit; cout<<" Account Created.."; } void account::show_account() const { cout<<" Account No. : "< >type; type=toupper(type); cout<<" Modify Balance amount : "; cin>>deposit; } void account::dep(int x) { deposit+=x; } void account::draw(int x) { deposit-=x; } void account::report() const { cout< //*************************************************************** // function declaration //**************************************************************** void write_account(); //function to write record in binary file void display_sp(int); //function to display account details given by user void modify_account(int); //function to modify record of file void delete_account(int); //function to delete record of file void display_all(); //function to display all account details void deposit_withdraw(int, int); // function to desposit/withdraw amount for given account void intro(); //introductory screen function //*************************************************************** // THE MAIN FUNCTION OF PROGRAM //**************************************************************** int main() { char ch; int num; intro(); do { system("cls"); cout<<" \tMAIN MENU"; cout<<" \t01. NEW ACCOUNT"; cout<<" \t02. DEPOSIT AMOUNT"; cout<<" \t03. WITHDRAW AMOUNT"; cout<<" \t04. BALANCE ENQUIRY"; cout<<" \t05. ALL ACCOUNT HOLDER LIST"; cout<<" \t06. CLOSE AN ACCOUNT"; cout<<" \t07. MODIFY AN ACCOUNT"; cout<<" \t08. EXIT"; cout<<" \tSelect Your Option (1-8) "; cin>>ch; system("cls"); switch(ch) { case '1': write_account(); break; case '2': cout<<" \tEnter The account No. : "; cin>>num; deposit_withdraw(num, 1); break; case '3': cout<<" \tEnter The account No. : "; cin>>num; deposit_withdraw(num, 2); break; case '4': cout<<" \tEnter The account No. : "; cin>>num; display_sp(num); break; case '5': display_all(); break; case '6': cout<<" \tEnter The account No. : "; cin>>num; delete_account(num); break; case '7': cout<<" \tEnter The account No. : "; cin>>num; modify_account(num); break; case '8': cout<<" \tThanks for using bank managemnt system"; break; default :cout<<"\a"; } cin.ignore(); cin.get(); }while(ch!='8'); return 0; } //*************************************************************** // function to write in file //**************************************************************** void write_account() { account ac; ofstream outFile; outFile.open("account.dat",ios::binary|ios::app); ac.create_account(); outFile.write(reinterpret_cast (&ac), sizeof(account)); outFile.close(); } //*************************************************************** // function to read specific record from file //**************************************************************** void display_sp(int n) { account ac; bool flag=false; ifstream inFile; inFile.open("account.dat",ios::binary); if(!inFile) { cout<<"File could not be open !! Press any Key..."; return; } cout<<" BALANCE DETAILS "; while(inFile.read(reinterpret_cast (&ac), sizeof(account))) { if(ac.retacno()==n) { ac.show_account(); flag=true; } } inFile.close(); if(flag==false) cout<<" Account number does not exist"; } //*************************************************************** // function to modify record of file //**************************************************************** void modify_account(int n) { bool found=false; account ac; fstream File; File.open("account.dat",ios::binary|ios::in|ios::out); if(!File) { cout<<"File could not be open !! Press any Key..."; return; } while(!File.eof() && found==false) { File.read(reinterpret_cast (&ac), sizeof(account)); if(ac.retacno()==n) { ac.show_account(); cout<<" Enter The New Details of account"< (sizeof(account)); File.seekp(pos,ios::cur); File.write(reinterpret_cast (&ac), sizeof(account)); cout<<" \t Record Updated"; found=true; } } File.close(); if(found==false) cout<<" Record Not Found "; } //*************************************************************** // function to delete record of file //**************************************************************** void delete_account(int n) { account ac; ifstream inFile; ofstream outFile; inFile.open("account.dat",ios::binary); if(!inFile) { cout<<"File could not be open !! Press any Key..."; return; } outFile.open("Temp.dat",ios::binary); inFile.seekg(0,ios::beg); while(inFile.read(reinterpret_cast (&ac), sizeof(account))) { if(ac.retacno()!=n) { outFile.write(reinterpret_cast (&ac), sizeof(account)); } } inFile.close(); outFile.close(); remove("account.dat"); rename("Temp.dat","account.dat"); cout<<" \tRecord Deleted .."; } //*************************************************************** // function to display all accounts deposit list //**************************************************************** void display_all() { account ac; ifstream inFile; inFile.open("account.dat",ios::binary); if(!inFile) { cout<<"File could not be open !! Press any Key..."; return; } cout<<" \t\tACCOUNT HOLDER LIST "; cout<<"==================================================== "; cout<<"A/c no. NAME Type Balance "; cout<<"==================================================== "; while(inFile.read(reinterpret_cast (&ac), sizeof(account))) { ac.report(); } inFile.close(); } //*************************************************************** // function to deposit and withdraw amounts //**************************************************************** void deposit_withdraw(int n, int option) { int amt; bool found=false; account ac; fstream File; File.open("account.dat", ios::binary|ios::in|ios::out); if(!File) { cout<<"File could not be open !! Press any Key..."; return; } while(!File.eof() && found==false) { File.read(reinterpret_cast (&ac), sizeof(account)); if(ac.retacno()==n) { ac.show_account(); if(option==1) { cout<<" \tTO DEPOSITE AMOUNT "; cout<<" Enter The amount to be deposited"; cin>>amt; ac.dep(amt); } if(option==2) { cout<<" \tTO WITHDRAW AMOUNT "; cout<<" Enter The amount to be withdraw"; cin>>amt; int bal=ac.retdeposit()-amt; if((bal<500 && ac.rettype()=='S') || (bal<1000 cout<<"insufficience balance"; else ac.draw(amt); } int pos =(-1)*static_cast (sizeof(ac)); File.seekp(pos,ios::cur); File.write(reinterpret_cast (&ac), sizeof(account)); cout<<" \t Record Updated"; found=true; } } File.close(); if(found==false) cout<<" Record Not Found "; } //*************************************************************** // INTRODUCTION FUNCTION //**************************************************************** void intro() { cout<<" \t BANK"; cout<<" \tMANAGEMENT"; cout<<" \t SYSTEM"; cout<<" MADE BY : your name"; cout<<" SCHOOL : your school name"; cin.get(); }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
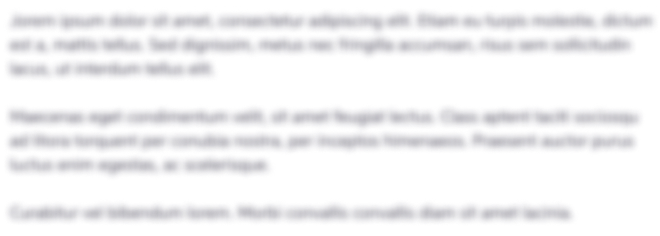
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started