Question
Problem Statement Create a to-do-list and associated operations using linked-lists in python. The To-Do list should have the capability of: Adding/Creating a task Assigning a
- Problem Statement
Create a to-do-list and associated operations using linked-lists in python.
The To-Do list should have the capability of:
- Adding/Creating a task
- Assigning a task-Id
- Removing a task
- Searching a task
- Marking a task completed
- Unmarking a task as completed
- List status showing all completed and incomplete tasks and associated details.
To solve this problem, create a linked-list of tasks and mark them as complete and incomplete.
Youll will be given few commands in the input file.
Operations:
- def initiateToDoList(read_input_file): This function reads the input file and creates a to-do
list and all associated data structures and calls the necessary functions as mentioned in the
input file.
Input: Input-File name with path.
Output: None
- def addTask(task_string=): This function is called by initiateToDoList and creates a task
along with a unique task-number and writes this task-number in the output file.
Input : Task string.
Output: ADDED:
- def removeTask(task_string=, task_number=): Description: This function is called by
initiateToDoList and removes a task along with a unique task-number and writes this task-
number in the output file.
Input : Task string. OR task_number.
Output: REMOVED:
- def searchTask(search_string=): This function is called by initiateToDoList and find a task
along with a unique task-number and writes this task-number in the output file.
Input : Task string. OR task_number.
Output: SEARCHED:
----------------------------------------
----------------------------------------
- def completeTask(task_string=, task_number=): This function is called by
initiateToDoList and marks a task along with a unique task-number as complete and writes
this task-number in the output file.
Input : Task string. OR task_number.
Output: COMPLETED:
- def incompleteTask(task_string=, task_number=): This function is called by
initiateToDoList and unmarks a task along with a unique task-number as complete and writes
this task-number in the output file.
Input : Task string. OR task_number.
Output: UNCOMPLETED:
- def statusTask(): This function is called by initiateToDoList and unmarks a task along with a
unique task-number as complete and writes this task-number in the output file.
Input :
Output: TASK STATUS:
--------------------------------------------------
--------------------------------------------------
Requirements:
- Implement the above problem statement using Python 3.7
- Read the input from a file(inputPS10.txt), which contains the list of tasks and associated
actions to be taken identified by relevant tags at the start of each line separated with a colon.
- You will output your answers to a file (outputPS10.txt) for each line.
- Perform an analysis for the features above and give the running time in terms of input size: n.
Sample Input:
Input will be taken from the file(inputPS10.txt).
Add a Task: Complete Assignment.
Add a Task: Return Library Books.
Add a Task: Book a ticket.
Add a Task: Wish Birthday
Remove Task: Wish Birthday
Mark Complete: Complete Assignment.
Task Status:
Mark InComplete: Complete Assignment.
Remove Task: Complete Assignment.
Add a Task: Call Home.
Add a Task: Read Algorithm book
Remove Task: Call Home. Search Task: book.
Add a Task: wear mask,
Sample Output:
Display the output in outputPS10.txt.
ADDED:TA3291-Complete Assignment.
ADDED:TA8920-Return Library Book.
ADDED:TA4836-Book a ticket.
COMPLETED:TA3291-Complete Assignment.
TASK-STATUS:
Task-Number Task-String Task-Status
----------------------------------------------------
TA3291 Complete Assignment. C
TA8920 Return Library Book. I
TA4836 Book a ticket. I
----------------------------------------------------
UNCOMPLETED:TA3291-Complete Assignment. REMOVED:TA3291-Complete Assignment.
ADDED:TA3111-Call Home.
ADDED:TA3916-Read Algorithm book
REMOVED:TA3111-Call Home. SEARCHED:book
--------------------------------
TA8920-Return Library Book. TA4836-Book a ticket.
---------------------------- ADDED:TA9374-wear mask,
Step by Step Solution
There are 3 Steps involved in it
Step: 1
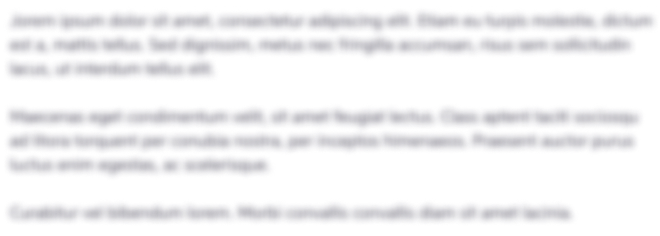
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started