Question
Problem Statement We encounter projectile motion whenever we attempt to catch a fly ball, hit a long drive, or shoot a free throw. The laws
Problem Statement
We encounter projectile motion whenever we attempt to catch a fly ball, hit a long drive, or shoot a free throw. The laws of physics, which describe projectile motion, are well known. They were first studied by Napoleons generals. To simplify the problem, we will assume that we can
- consider the surface of the Earth to be a plane
- ignore the effect of the drag from air friction
Under the simplifying conditions above, the following formulas hold for a projectile with an initial velocity of v feet/sec and a launch angle of r (note that these expressions are not written according to the syntax of the Java language).
-
Time span of the flight: T = 2 v sin r / g
-
Maximum height: ymax = (v sin r) t - 12 g t2, where t = T/2
-
Range (distance traveled by the projectile): xmax = (v cos r) T
-
Here g is the gravitational acceleration g = 32 ft/sec2 (in English units) and r is measured in radians. This project simulates the process of repeated attempts to hit a target with a projectile. The goal is to shoot the projectile within a 1-foot distance from the target, since such a short miss is accepted as a hit. You will construct a Java program that
-
can check if the initial attempt overshoots the target with more than 1 foot; if so the process is terminated and then restarted with an increased initial velocity (note that for any initial velocity the longest range is attained if the launch angle is of 45 degrees)
-
can determines the error of a shot (error = projectile range distance to target)
-
can check if the error is less than 1 foot in absolute value; if so, the user is notified about
the result and the process terminates
-
can offer the user four chances to modify the launch angle and try to hit the target
-
can keep track of the smallest error produced by the subsequent attempts; the best result is reported to the user
Analysis and requirements
The analysis describes the logical structure of the problem in a way which helps us to plan (design) a solution.
Input
Initial input values are (i) initial velocity (feet/sec)
(ii) distance to the desired target (feet) (iii) the gravitational acceleration (a constant)
Additional input values are the launch angles for the repeated attempts if applicable. The angle must always be in the range of 0.0 45.0 degrees. Distance, velocity and launch angle are solicited from the user on JOptionPane input windows.
Output
Output messages are displayed both on JOptionPane windows and on the console. Every time a launch has been executed by the program, a report providing the details of the trajectory must be displayed
Each report must contain
(1) the initial velocity in feet/sec; (2) the current launch angle in degrees; (3) the flight time in seconds; (4) the maximum height attained; (5) the distance traveled by the projectile (range); (6) the error with which the projectile missed the target
Note that the error is a positive value when the projectile overshoots, and negative for short shots. All real numbers displayed must be rounded to the nearest hundredth.
The first report is based upon launch of 45 degrees (which provides the longest possible range) to see if the target is within reach at all for the given velocity. If the first attempt falls short of the target, another window as shown on Figure 5 displays the information and then the program exits.
If the first shot is long enough, the window of Figure 3 shall be used to input all subsequent launch angle modifications as chosen by the user. The corresponding reports of Figure 4 show the re-calculated trajectories. Naturally, the user will try to modify the angle so as to make the projectile land nearer and nearer to the target. After each unsuccessful attempt a warning is printed to the console, see a sample on Figure 6.
"Shot fell short of the target. Increase the launch angle!"
Figure 6
Figure 7 shows a sample output on the console after all four angle modifications failed to hit the target.
Shot went beyond the target. Decrease the launch angle!
Shot went beyond the target. Decrease the launch angle!
Shot fell short of the target. Increase the launch angle!
Shot fell short of the target. Increase the launch angle!
Your best shot missed the target with 4.47 feet.
Figure 7
Note that it is necessary to keep track of the least absolute error occurred in the series of attempts, since it has to be reported as the last line in Figure 7 shows.
Figure 8 shows a successful launch. Such a report is followed by the message of Figure 9.
Relevant Formulas
The basic formulas 1, 2 and 3 in the Problem Statement will be used for all trajectory computations. The input value for a launch angle shall be solicited and given in degrees. The previous formulas shall be implemented by making use of the trig methods Math.sin(angle ) and Math.cos(angle ) from the Math class. These methods require the parameter angle in radians. Therefore an angle given in degrees must be converted to radians. The conversion formula runs as follows:
(angle in radians) = (angle in degrees) /180
Note that it can be used in either direction, from degrees to radians or vice versa. The value of (PI) is available as a named constant Math.PI from the Math class of the Java library.
Design
For this project you shall design a single class which contains all the necessary data and operations. A suggested class name is Projectile. You must decide upon the necessary import(s).
The Projectile class contains the main method, which in turn contains all the variable declarations. The main method should carry out the following tasks in the order given:
-
declares and assigns a named constant for the gravitational acceleration; the value is 32
-
solicits and stores the input values as explained in the Analysis section
-
computes all the trajectory data and saves those in variables
-
builds up and stores the output message in a single string variable
-
displays the output windows
-
numbers in the output are formatted to the nearest hundredth; for this purpose the String.format( ) and printf( ) methods are used
-
uses if and/or if-else logic to decide if additional launches are necessary and repeats the operations at most for times if needed
-
terminates the program when it is due
Step by Step Solution
There are 3 Steps involved in it
Step: 1
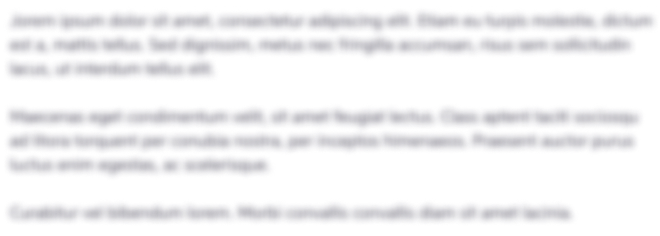
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started