Question
Problem: Write a program that prints the calendar for a given year or a given month. The user will be given the option of printing
Problem:
Write a program that prints the calendar for a given year or a given month. The user will be given the option of printing the calendar for the whole year or just a month. If the calendar for the whole year is being printed then the data for thanksgiving must be specified. You are required to create multiple methods. Also you need to consider if the year is a leap year or not.
How to proceed to solving this problem:
You need to find out how many days has passed since Jan 1800 up to the given year. You must check if the year is a leap year or not to know the number of the days in February.
You need to find out the first day of the month for each given month which is going to be Sunday, Monday, Tuesday, Wednesday, Thursday, Friday or Saturday.
You need to find out the Thanksgiving Day for the given year.
Now you are ready to print the calendar.
Refer to the provided shell for more details.
Requirements:
Must implement all the provided methods in the shell
Your program must work for a leap year as well
Your program must output a description indicating what the program does
Naming conventions
Indentation
Proper way of calling methods
Use of conditional statements
You are allowed to be creative by adding more functionality to your program
You can deviate from the provided shell and create your own solution. However you must use ample number of methods.
This is the Shell for the java:
import java.util.Scanner;
public class PrintCalendar
{
/*The main method has only one line of code and it calls the process
method
You do not need to change anything in this method*/
public static void main(String[] args)
{
process();
}
/*This method ask the required info and calls the appropriate methods
based on the user's input to either print
the calendar for the month or for the year. You can declare your
Scanner object in this method since it
will only be used here */
public static void process()
{
}
//this method prints the body of the calender for the given month by
calling the the methods printMonthTable and printMonthBody
public static void printMonth(int year, int month)
{
printMonthTitle(year, month);
printMonthBody(year, month);
}
//this method prints the title of the days in each week(sunday Mon Tues
Wed Thur Fir Sat)
/*This method prints the following
month name year
----------------------------
Sun Mon Tue Wed Thu Fri Sat
this method gets the month as an integer and you need to call the
appropriate method to get the name of the month*/
public static void printMonthTitle(int year, int month)
{
}
/*this method calls the method getStartday to find out the first day of
the month(sunday, monday, tuesday, wednesday, thursday or friday
then it calls the method print by passing the startday, year and
month*/
public static void printMonthBody(int year, int month)
{
}
/*This method prints the days in the month, you will need to call the
getNumberOfDaysInMonth method
to get the appropriate number of days and you will also need to print
out the day that Thanksgiving
is on if the month is November (using the thanks method)*/
public static void print(int startDay, int year, int month)
{
}
/*This method finds out the day of thanksgiving
you must use switch statements . Thanksgiving is the last Thursday of
November therefore we need to add a value to 21.
for example if the first of the month is sunday then from sunday to
thurday is 5 days, then we add 5 to 21 and that would be the thanksgiving
day.
The first day of November 2018 is Thursday and so thanksgiving will be
on 1 + 3 * 7 = 22*/
public static int thanks(int startDay)
{
return 0;
}
/*This method gets the number of the month and returns the name of
the month for example the month is 2 then this method must return
Febuary*/
public static String getMonthName(int month)
{
return "";
}
/*this method returns the first day of the month which is a number,
Sunday - saturday is assosiated with 0 - 6.
you need to know how many days has passed since 1800, therefore we call
the method getTotalNumberOfDays.
Jan 1st 1800 was on a wednesday.
After you have the total number of the days you can find out the number
of the weeks has passed since Jan 1800
the leftover (mod by 7) will be the start day for the given month and
the number of the days left
*/
public static int getStartDay(int year, int month)
{
return 0;
}
/*use a for loop to calculate the total number of the days has passed
since 1800 to the (year -1)
use another for loop to add the number of the days in each month up to
the month entered by the user.
also remember to check if the year is a leap year or not.
this method needs to call getNumberOfDaysInMonth method*/
public static int getTotalNumberOfDays(int year, int month)
{
return 0;
}
/*This method returns the number of the days in the given month, if the
month is Feb and a leapyear returns 29 else it returns 28*/
public static int getNumberOfDaysInMonth(int year, int month)
{
return 0;
}
/*returns true if a year is a leap year otherwise it will return
false*/
public static boolean isLeapYear(int year)
{
return true;
}
This is the output:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
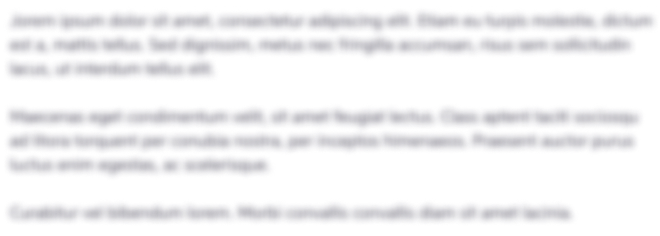
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started