Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Program #6 Binary Search Trees Total points: 100 Objectives Write and use a linked implementation of a binary search tree. Problem Description In this




Program #6 Binary Search Trees Total points: 100 Objectives Write and use a linked implementation of a binary search tree. Problem Description In this assignment, you will practice solving a problem using a binary search tree. You will implement a Java application that could be used in a restaurant. You are asked to implement four classes: Menultem, BSTNode, BST and Driver. Each of these classes is described below. Classes The method names, parameters and return types must match the specification exactly. You may add other methods if you wish. Menultem Class The Menultem class represents one item ordered from a menu. The Menultem has three instance variables: name (of type String), qty (of type int) and price (of type double). Implement the following methods for the Menultem class: Menultem (String, int, double): A constructor to initialize all instance variables. A value for each instance variable will be passed as a parameter to the constructor. The parameters must be in the order (name, qty and price). The instance variable name will be used as the search key and as the instance variable that will determine the order in which the items are stored in the binary search tree. Getters for all instance variables. Setter methods for each instance variable. equals(): Implement the equals() method for your Menultem where two Menultems are considered equal when they have the same search key (name). Other instance variables must be ignored. Use the standard signature for the equals() method. Note that, the equality of String attributes should be case insensitive. For example, "MATH", "math" and "Math" match each other. In order to compare strings in Java use the String's equalsignoreCase() method. For example, the following code should print true: String strl = "Hello"; String str2 = "hello"; System.out.println (strl.equals Ignore Case (str2)); toString(): a method that returns a nicely formatted string description of the item. All items should be separated by tabs (use "\t") and the entire Menultem should be displayed on one line with no labels. In addition to name, price and qty, calculate the total value of the Menultem (price* qty) and add it to the end of the string. BST Rice $1.50 3 $4.50 Use the Number Format or Decimal Format class to display prices with exactly two digits following the decimal place. Implement the Comparable interface and the compareTo() method for Menultem. compareTo() returns a negative number when the invoking object's search key (name) is less than the parameter's search key BSTNode Class Create a BSTNode class. You must create your own BSTNode class and you may not use any node classes from the Java library. The BSTNode instance variables consist of data and two links, left and right. The data instance variable must be of type Menultem. O when the two search keys (names) are equal a positive number when the invoking object's search key (name) is greater than the parameter's search key For example, "ant".compareTo("bat") will return a negative number. Note that the comparison of String attributes should be case insensitive. For example, "MATH", "math" and "Math" match each other. Base the comparison only on the search key. All other instance variables must be ignored. The instance variables must be private. BSTNode(data: Menultem, left: BSTNode, right: BSTNode): Implement a constructor with parameters data, left and right, in that order. Implement getters and setters for all instance variables. Your BST class must be separate from your BSTNode class. You may implement a generic BSTNode class if you like. The Menuitems data must be stored in a recursive binary search tree data structure. This means the data is stored in order according to the binary search tree principles. You must create your own binary search tree class (separate from the BSTNode class) and implement the recursive methods you need. You must create your own BST class and you may not use any tree classes from the Java library. Be sure to implement encapsulation by having a clean separation between the node and binary search tree classes. Do this by making the instance variables private in both classes and by putting methods that manipulate more than one node in the BST class. Use a linked implementation. The BST class will have one private instance variable, root, which will hold a reference to the topmost node in the tree. Implement a no-arguments constructor that instantiates an empty binary search tree. The BST class methods must include these recursive methods: o void insert(Menultem mi): a method that takes one input parameter, a Menultem, and adds it to the binary search tree in the proper spot, preserving the binary search tree property. If the Menultem is already in the tree, insert() will not add a new item to the tree but rather will increment the qty of the existing item by the new qty. If the Menultem is not already in the tree, the method inserts the Menultem in its correct position void preorder() - traverses the tree in pre-order (based on the search key) and prints the contents of each Node o void postorder() - traverses the tree in post-order (based on the search key) and prints the contents of each Node o void inorder() - traverses the tree in post-order (based on the search key) and prints the contents of each Node o size(): a method that returns the number of nodes in the tree oint depth(): returns the height (depth) of the tree. Note: An empty tree has a height of -1. A tree with one node has height of 0. o getTotal Qty(): an integer method that sums and returns the quantities of all Menultems in the tree o Menultem search(String name) - traverses the tree and returns a reference to a Menultem with a search key (name) that matches the parameter. Returns null if not found. o o getTotal Before Tax(): a method that calculates and returns the total value of all items in the tree. Remember to multiply the price by the quantity to get the total for each item. getTax(): a method with one parameter, taxRate (a double). getTax() calculates and returns a double representing the tax to be paid on the order. Not recursive. getTip(): a method with one parameter, percentage (a double). getTip() calculates and returns a double representing the tip to be paid on the order's total before tax. Not recursive. o otoString(): a method that returns a string representation of all the Menultems in the tree. The items will be displayed in alphabetical order. The String includes the following: a restaurant name and some header lines details of all the Menultems, one per line the total of all the items in the tree before tax and tip been added the amount of tax to be added (use 8% when calling getTax()) Item the amount of tip to be added (use 20% when calling getTip()) the total after tax and tip have been added Sample of String returned from toString(): Downtown Cafe Injera Rice Sambusa Sushi Total: Tax: Tip: Price $2.50 $1.50 $5.80 $8.25 $46.70 $3.74 $9.34 Qty Total 1 N3WH 4 2 $2.50 $4.50 $23.20 $16.50 Grand total: $59.78 You are required to use the approach outlined in class with a public method with no arguments (one argument in the case of insert(), search(), getTax() and getTip()) and a private helper method. You may not directly access the root of the tree from the driver. You may not* implement a public getRoot() method. With the exception of inorder(), preorder() and postorder(), the BST class should not contain any print statements. All printing occurs in the Driver. Driver Use the Driver class to demonstrate that the BST class and its methods work properly. Create one or two BST trees. Add 8 to 15 Menultems to the trees. Change the order of insertion to test different tree topologies. Do not add the items in alphabetical order or your tree will degenerate to a linked list. Call all the BST methods and display the results. Be sure to test the case where the Menultem is already in the tree (insert() causes the existing item's quantity to be changed). Print both orders in a nicely formatted output. Other Use NumberFormat or DecimalFormat to display money with a dollar sign, commas and exactly two digits after the decimal place (e.g., $1,234.56). With the exception of inorder(), preorder() and postorder(), do not print from any class except the Driver. All instance variables must be private. Additional Requirements Provide a UML class diagram for the BST class. Provide a UML structure diagram. Provide an invariant for the BST class. You are not required to provide any other comments for this program although you may find that adding comments helps you to understand the problem. Implement the classes as described above. Use good coding style throughout (see slide deck 01-D Objects and Chapter 1 of your text) including o correct private/public access modifiers o consistent indenting o meaningful variable names o normal capitalization conventions o other aspects of easy-to-read code You are free to adopt and adapt code from the textbook and class slides. However, document the source of any borrowed code.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
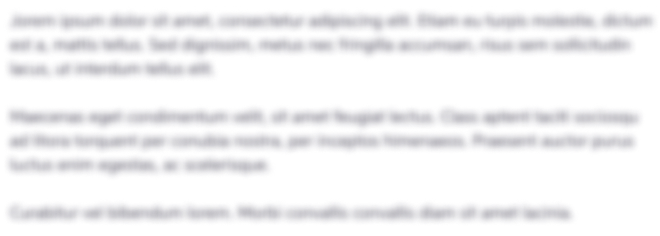
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started