Question
Program Assignment 2 : #1. Fence-post and Random numbers 1. Write an interactive program that prompts for a desired sum, then repeatedly rolls two six-sided
Program Assignment 2 :
#1. Fence-post and Random numbers
1. Write an interactive program that prompts for a desired sum, then repeatedly rolls two six-sided dice until their sum is the desired sum. You are supposed to generate random numbers to simulate two dice. Here is the expected dialogue with the user: (4-credit)
Desired dice sum: 9
4 and 3 = 7
3 and 5 = 8
5 and 6 = 11
5 and 6 = 11
1 and 5 = 6
6 and 3 = 9
Finish the following code file:
import java.util.*;
public class TestDiceRoll {
public static void main(String[] args) {
Scanner console = new Scanner(System.in);
System.out.print("Desired dice sum: ");
int sum = console.nextInt();
// your code here
}
}
2. Write a code that prints a random number of lines between 2 and 8 lines inclusive, where each line contains a random number of '$' signs between 5 to 30 inclusive (4-credit). For example:
$$$$
$$$$$$$$$$$$$$$
$$$$$$$
$$$$$$$$$$$$$$$$$$$$$$$$$$$$$$
$$$$$$$$$$
$$$$$$$$$$$$$$$
$$$$$$
3. Write a method randomWalk that performs a random one-dimensional walk, reporting each position reached and the maximum position reached during the walk. The random walk should begin at position 0. On each step, you should either increase or decrease the position by 1 (with equal probability). The walk stops when 3 or -3 is hit. The output should look like this: (4-credit)
position = 0
position = 1
position = 0
position = -1
position = -2
position = -1
position = -2
position = -3
max position = 1
4. Write a static method named gcd that accepts two integers as parameters and returns the greatest common divisor (GCD) of the two numbers. The greatest common divisor of two integers a and b is the largest integer that is a factor of both a and b. The GCD of any number and 1 is 1, and the GCD of any number and 0 is that number. One efficient way to compute the GCDis to use Euclid's algorithm, which states the following: (3-credit)
GCD(a, b) = GCD(b, a % b)
GCD(a, 0) = Absolute value of a
For example, gcd(24, 84) returns 12, gcd(105, 45) returns 15, and gcd(0, 8) returns 8.
Test your code with the following code file.
public class TestGCD {
public static void main(String[] args) {
System.out.println("GCD of 27 and 6 is " + gcd(27, 6)); // 3
System.out.println("GCD of 24 and 84 is " + gcd(24, 84)); // 12
System.out.println("GCD of 38 and 7 is " + gcd(38, 7)); // 1
System.out.println("GCD of 45 and 105 is " + gcd(45, 105)); // 15
System.out.println("GCD of 1 and 25 is " + gcd(1, 25)); // 1
System.out.println("GCD of 25 and 1 is " + gcd(25, 1)); // 1
System.out.println("GCD of 0 and 14 is " + gcd(0, 14)); // 14
System.out.println("GCD of 14 and 0 is " + gcd(14, 0)); // 14
}
public static int gcd(int a, int b) {
// your code goes here
}
}
Submission:
please archive the source codes and documents describing program structure and execution steps and program results (screen capture, etc.) into one zip file and upload it to the BlackBoard as the attachment.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
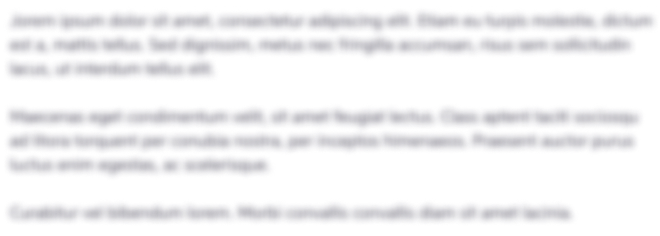
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started