Answered step by step
Verified Expert Solution
Question
00
1 Approved Answer
program in java please keep it simple and follow all the steps include exceptions when possible does not specify what kinda of collection is being
program in java please keep it simple and follow all the steps include exceptions when possible
does not specify what kinda of collection is being implemented use best judgment
Part 1: Implement your "Thing" class Start by choosing what sort of list you would like to keep. For example: To-do list Vacation and travel information Pets Real estate properties pretty much any sort of list You can be creative about your list. It is prohibited to use books, points, lines, circles, grocery items, students, persons, bank accounts, cars or songs. Please note that you may reuse your "Thing" class across more than one programming assignment, so choose something that interests you. Your "Thing" class must have the following characteristics: a meaningful name (not "Thing") has at least 3 instance variables such that: o one instance variable must be integer (i.e., int) and this variable will be used to find aggregate information about "Thing"s that are stored in a collection class as will be explained later. o one instance variable must be String and this variable will be used as a search key. o the third variable can be of any type based on the semantics of your "Thing". All instance variables must be private. Implement getters and setters for all the attributes of your "Thing". (Note that Eclipse will automagically create them for you with Source Generate Getters and Setters.) Implement a toString() method that returns a String representation of your "Thing" class where all the instance variables are in one line and separated by tabs. Implement the equals() method for your "Thing" where two things are considered equal when they have the same search key. Note that, the equality of String attributes should be case insensitive. For example, "MATH","math" and "Math" match each other. In order to compare strings in Java use the String's equalsIgnoreCase () method. For example, the following code should print true: String strl = "Hello"; String str2 = "hello"; System.out.println(stri.equalsIgnoreCase(str2)); Implement the Comparable interface and the compareTo() method for your "Thing". compareTo() returns o o o a negative number when the invoking object's search key is less than the parameter's search key O when two search keys are equal a positive number when the invoking object's search key is greater than the parameter's search key For example, "Ant".compareTo("bat") will return a negative number. Note that the comparison of String attributes should be case insensitive. For example, "MATH", "math" and "Math" match each other. Part 2: Implement a Collection class Implement a collection class of "Things" using an array. a. You may NOT use the Java library classes ArrayList or Vector or any other java collection class. b. You must use simple java Arrays in the same way as discussed in class. The name of your collection class should include the name of your "Thing". For example, if your "Thing" is called Country, then your collection class should be called CountryArrayBag. Implement a constructor for your collection class that takes one input parameter that represents the maximum number of elements that can be stored in your collection. Implement the following methods in your collection class: 1.insert(): a method that takes three input parameters and uses these inputs to instantiate an object of type "Thing", initialize its instance variables, and then inserts it in the collection class IN ASCENDING ORDER according to the search key instance variable. Write a try/catch block to handle any exception that might occur (such as attempting to insert into a full list). 2. size(): a method that returns the number of objects in the collection. toString(): a method that returns a String representation of the collection that includes all elements that are stored in the collection. The output string must be nicely formatted in a tabular format, one "Thing" per line. For example, a list of students in order by name) could be displayed as follows: Name Age Major Eric 25 CIT Math Hanna John Reem 20 CS CS 4. find(): this method depends on the search key attribute of the "Thing" class. The method takes as input a String value and returns the first object with a search key that matches the input search value. Use the compareTel) method from the Thing class. 5. countOccurrences.(): this method depends on the search key attribute of the "Thing" class. The method takes as input a string value and returns as output the number of objects in the collection that have a search key attribute that matches the input search value. Use the compare Tol) method from the Thing class. 6. contains(): this method takes one input parameter of type "Thing". The method rfalse based on whether the collection contains at least one "Thing" that is equal to the input parameter. Use the equals() method in your "Thing" class to determine if two objects are equal. 7. total(): this method uses the int instance variable of the Thing class. The method calculates and returns the sum of the int instance variable of all objects stored in the collection. For example, in the student collection, this method finds the sum of age of all students in the list. This sum could be used, for example, along with the output of the size() method to find the average student age. countRange(): this method depends on the int instance variable of the "Thing" class. The method takes as input two int values and counts how many objects in the list have a value that lies in the given range. For example, in the student collection class that is displayed above, countRange (25, 30) returns 3 because there are 3 students with age values that fall in the range between 25 and 30 inclusive. Note that if the first input parameter is greater than the second input parameter then the method always returns 0 (i.e., countRange (30,25) returns 0). 9. delete(): similar to the contains method, this method takes one input parameter of type "Thing". The method then searches the collection for the first object that equals the input object and deletes its occurrence if found. After the item has been deleted, the list must still be maintained IN ORDER. The method also returns true if an object is deleted and false otherwise. 10. Write an iterator for your collection class that iterates through your collection in reverse order. Such an iterator could be used to display your objects in reverse alphabetical order. For example, Name Age Major Reem John Hanna Eric CS Math CIT Part 3: Implement your Driver class Implement a Driver class that works as follows: Create a collection class with capacity 10. Use the insert.() method to insert 5-10 items in the collection. You may use any arbitrary values in the objects to be inserted. The driver then calls the methods 2 through 10 in order. Display the results of each operation after it is performed. Label your output clearly. Do not use a Scanner to read any inputs from the user. Instead, use hard coded values for the different methods' inputs. Part 1: Implement your "Thing" class Start by choosing what sort of list you would like to keep. For example: To-do list Vacation and travel information Pets Real estate properties pretty much any sort of list You can be creative about your list. It is prohibited to use books, points, lines, circles, grocery items, students, persons, bank accounts, cars or songs. Please note that you may reuse your "Thing" class across more than one programming assignment, so choose something that interests you. Your "Thing" class must have the following characteristics: a meaningful name (not "Thing") has at least 3 instance variables such that: o one instance variable must be integer (i.e., int) and this variable will be used to find aggregate information about "Thing"s that are stored in a collection class as will be explained later. o one instance variable must be String and this variable will be used as a search key. o the third variable can be of any type based on the semantics of your "Thing". All instance variables must be private. Implement getters and setters for all the attributes of your "Thing". (Note that Eclipse will automagically create them for you with Source Generate Getters and Setters.) Implement a toString() method that returns a String representation of your "Thing" class where all the instance variables are in one line and separated by tabs. Implement the equals() method for your "Thing" where two things are considered equal when they have the same search key. Note that, the equality of String attributes should be case insensitive. For example, "MATH","math" and "Math" match each other. In order to compare strings in Java use the String's equalsIgnoreCase () method. For example, the following code should print true: String strl = "Hello"; String str2 = "hello"; System.out.println(stri.equalsIgnoreCase(str2)); Implement the Comparable interface and the compareTo() method for your "Thing". compareTo() returns o o o a negative number when the invoking object's search key is less than the parameter's search key O when two search keys are equal a positive number when the invoking object's search key is greater than the parameter's search key For example, "Ant".compareTo("bat") will return a negative number. Note that the comparison of String attributes should be case insensitive. For example, "MATH", "math" and "Math" match each other. Part 2: Implement a Collection class Implement a collection class of "Things" using an array. a. You may NOT use the Java library classes ArrayList or Vector or any other java collection class. b. You must use simple java Arrays in the same way as discussed in class. The name of your collection class should include the name of your "Thing". For example, if your "Thing" is called Country, then your collection class should be called CountryArrayBag. Implement a constructor for your collection class that takes one input parameter that represents the maximum number of elements that can be stored in your collection. Implement the following methods in your collection class: 1.insert(): a method that takes three input parameters and uses these inputs to instantiate an object of type "Thing", initialize its instance variables, and then inserts it in the collection class IN ASCENDING ORDER according to the search key instance variable. Write a try/catch block to handle any exception that might occur (such as attempting to insert into a full list). 2. size(): a method that returns the number of objects in the collection. toString(): a method that returns a String representation of the collection that includes all elements that are stored in the collection. The output string must be nicely formatted in a tabular format, one "Thing" per line. For example, a list of students in order by name) could be displayed as follows: Name Age Major Eric 25 CIT Math Hanna John Reem 20 CS CS 4. find(): this method depends on the search key attribute of the "Thing" class. The method takes as input a String value and returns the first object with a search key that matches the input search value. Use the compareTel) method from the Thing class. 5. countOccurrences.(): this method depends on the search key attribute of the "Thing" class. The method takes as input a string value and returns as output the number of objects in the collection that have a search key attribute that matches the input search value. Use the compare Tol) method from the Thing class. 6. contains(): this method takes one input parameter of type "Thing". The method rfalse based on whether the collection contains at least one "Thing" that is equal to the input parameter. Use the equals() method in your "Thing" class to determine if two objects are equal. 7. total(): this method uses the int instance variable of the Thing class. The method calculates and returns the sum of the int instance variable of all objects stored in the collection. For example, in the student collection, this method finds the sum of age of all students in the list. This sum could be used, for example, along with the output of the size() method to find the average student age. countRange(): this method depends on the int instance variable of the "Thing" class. The method takes as input two int values and counts how many objects in the list have a value that lies in the given range. For example, in the student collection class that is displayed above, countRange (25, 30) returns 3 because there are 3 students with age values that fall in the range between 25 and 30 inclusive. Note that if the first input parameter is greater than the second input parameter then the method always returns 0 (i.e., countRange (30,25) returns 0). 9. delete(): similar to the contains method, this method takes one input parameter of type "Thing". The method then searches the collection for the first object that equals the input object and deletes its occurrence if found. After the item has been deleted, the list must still be maintained IN ORDER. The method also returns true if an object is deleted and false otherwise. 10. Write an iterator for your collection class that iterates through your collection in reverse order. Such an iterator could be used to display your objects in reverse alphabetical order. For example, Name Age Major Reem John Hanna Eric CS Math CIT Part 3: Implement your Driver class Implement a Driver class that works as follows: Create a collection class with capacity 10. Use the insert.() method to insert 5-10 items in the collection. You may use any arbitrary values in the objects to be inserted. The driver then calls the methods 2 through 10 in order. Display the results of each operation after it is performed. Label your output clearly. Do not use a Scanner to read any inputs from the user. Instead, use hard coded values for the different methods' inputsStep by Step Solution
There are 3 Steps involved in it
Step: 1
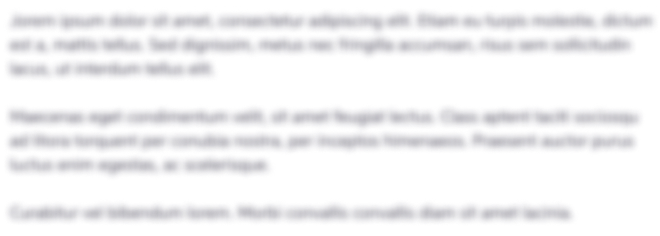
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started