Question
PROGRAMMING ASSIGNMENT #3 Write a C program that calculates and prints the average of two or more sales amounts entered by the user. The program
PROGRAMMING ASSIGNMENT #3 Write a C program that calculates and prints the average of two or more sales amounts entered by the user. The program should first prompt the user to enter the number of sales to process (make sure: at least 2 sales must be entered by user! Give an appropriate polite error message of your choice, and re-prompt) . It should then prompt the user for each sales amount. As each sales amount is entered, the program should ensure that the sales entered are greater zero. If the amount is not greater than zero, an error message should be displayed (as shown below), and the user should be re-prompted for a sales amount. Any bad sales entered should not be included in the average calculation. Once all values are entered, the program should calculate and print the average of all of the valid values entered. At the end display a message for the appropriate sales average. This program will feature the if statement as well as programming techniques for data validation. I call these trap loops. The loop traps the user into entering valid data (see online notes for details).
The dialog with the user will be as follows:
Welcome to the Sears Sales Analyzer.
This program calculates the average of as many
sales you wish to enter.
First, enter the number of sales to process: 4
Now enter the 4 sales to be processed.
Enter sales amount #1: 900.00
Enter sales amount #2: 800.00
Enter sales amount # 3: -200
*** Invalid entry. Sales amount must be positive. ***
Enter sales amount #3: 2500.01
Enter sales amount #4: 540.00
The Total of the 4 sales entered is $4740.01
The Average of the 4 sales entered is $1185.00
Your sales average is adequate.
Note that what the user types in is indicated by the blue area above. You should substitute your name in the welcome statement above. Notice the trap in sales #3. That would repeat continuously until the user enters a correct data value.
After the average is displayed, give the final statement based on the following:
If sales average < $1000 display Your sales average needs improvement.
Else If sales average < $2000 display Your sales average is adequate.
Else If sales average < $5000 display Congratulations you made the Silver Club.
Else If sales average >= $5000 display Congratulations you made the Elite Gold Club.
I did not provide an algorithm for this assignment, however I have included several hints and requirements below. They should be helpful. Other Hints/Requirements: * This program requires variables of type int and float. * Use printf and scanf statements to first prompt the user for the number of sales to be processed (dont forget the buffer clearing statement too!). * This program requires loop structures. (for, while or do -- your choice). * The loop requires some a printf and scanf statement to prompt user for a sales amounts. * The loop also requires a selection structure (if, or if else) statement to check for bad data values. * The validation of data requires a printf statement to output error message an improper amount is entered. * The program requires an accumulator to keep track of the sum of good sales entered. * Once all sales amounts are entered, determine average and then use printf statements for average, total and a message based on the average.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
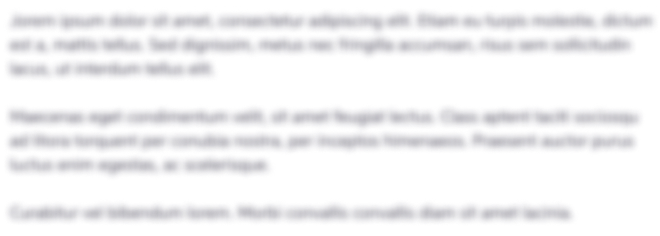
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started