Question
Programming Assignment (50 points) 1. You are given a partially completed program hw05q1.c. The structure of this homework is similar to previous homework. In this
Programming Assignment (50 points) 1. You are given a partially completed program hw05q1.c. The structure of this homework is similar to previous homework. In this homework, you should use linked list to do the same work in the previous homework. You should follow the instructions given in the program to complete the functions so that the program executes properly. You will be completing a program that creates a linked list of student records. It is a menu-driven program where the user is given the following options: a) Add a new student to the list. When adding a new student to the list, the user is prompted for student's name, major, school year of student and ID number of the student. The student should be added at sorted position. If the student already exists in the list, then you should not add to the list. The school year is enum type. The sorting should happen within the list. You should not create a new linked list of students having sorted students. b) Display the list of students. This should display each students details one after the other. c) Remove the student from the linked list. After removing the student, the linked list should not be broken. Also, make sure to free the associated memory after removing the student. Expected output of each menu option: (similar as previous homework)
addSort:
// READ BEFORE YOU START: // You are given a partially completed program that creates a linked list of patient records. // Each record(struct) has this information: student's name, major, school year of student, ID number. // The struct 'studentRecord' holds information of one student. School year is enum type. // A linked list called 'list' is made to hold the list of students. // To begin, you should trace through the given code and understand how it works. // Please read the instructions above each required function and follow the directions carefully. // You should not modify any of the given code, the return types, or the parameters, you risk getting compile error. // You are not allowed to modify main (). // You can use string library functions. // ***** WRITE COMMENTS FOR IMPORANT STEPS OF YOUR CODE. ***** // ***** GIVE MEANINGFUL NAMES TO VARIABLES. *****
#include
#pragma warning(disable: 4996) // for Visual Studio Only
#define MAX_NAME_LENGTH 25
typedef enum { freshman = 0, sophomore, junior, senior } schoolYearType; // enum type
struct studentRecord { char studentName[MAX_NAME_LENGTH]; char major[MAX_NAME_LENGTH]; schoolYearType schoolYear; unsigned int IDNumber; struct studentRecord* next; // pointer to next node } *list = NULL; // Declare linked list 'list'
// forward declaration of functions (already implmented) void flushStdIn(); void executeAction(char);
// functions that need implementation: int addSort(char* studentName_input, char* major_input, char* schoolYear_input, unsigned int IDNumber_input); // 20 points void displayList(); // 10 points int countNodes(); // 5 points int deleteNode(char* studentName_input); // 10 points void swapNodes(struct studentRecord* node1, struct studentRecord* node2); // 5 points
int main() { char selection = 'i'; // initialized to a dummy value printf(" CSE240 HW6 "); do { printf(" Currently %d student(s) on the list.", countNodes()); // NOTE: countNodes() called here printf(" Enter your selection: "); printf("\t a: add a new student "); printf("\t d: display student list "); printf("\t r: remove a student from the list "); printf("\t q: quit "); selection = getchar(); flushStdIn(); executeAction(selection); } while (selection != 'q');
return 0; }
// flush out leftover ' ' characters void flushStdIn() { char c; do c = getchar(); while (c != ' ' && c != EOF); }
// Ask for details from user for the given selection and perform that action // Read the code in the function, case by case void executeAction(char c) { char studentName_input[MAX_NAME_LENGTH], major_input[MAX_NAME_LENGTH]; unsigned int IDNumber_input, result = 0; char schoolYear_input[20]; switch (c) { case 'a': // add student // input student details from user printf(" Enter student name: "); fgets(studentName_input, sizeof(studentName_input), stdin); studentName_input[strlen(studentName_input) - 1] = '\0'; // discard the trailing ' ' char printf("Enter major: "); fgets(major_input, sizeof(major_input), stdin); major_input[strlen(major_input) - 1] = '\0'; // discard the trailing ' ' char
printf("Enter whether student is 'freshman' or 'sophomore' or 'junior' or 'senior': "); fgets(schoolYear_input, sizeof(schoolYear_input), stdin); schoolYear_input[strlen(schoolYear_input) - 1] = '\0'; // discard the trailing ' ' char printf("Please enter ID number: "); scanf("%d", &IDNumber_input); flushStdIn();
// add the student to the list result = addSort(studentName_input, major_input, schoolYear_input, IDNumber_input); if (result == 0) printf(" Student is already on the list! "); else if (result == 1) printf(" Student successfully added to the list! "); break;
case 'd': // display the list displayList(); break;
case 'r': // remove a student printf(" Please enter student name: "); fgets(studentName_input, sizeof(studentName_input), stdin); studentName_input[strlen(studentName_input) - 1] = '\0'; // discard the trailing ' ' char
// delete the node result = deleteNode(studentName_input); if (result == 0) printf(" Student does not exist! "); else if (result == 1) printf(" Student successfully removed from the list. "); else printf(" List is empty! "); break;
case 'q': // quit break; default: printf("%c is invalid input! ",c); } }
// Q1 : addSort // This function is used to insert a new student into the list. You can insert the new student to the end of the linked list. // Do not allow the student to be added to the list if that student already exists in the list. You can do that by checking the names of the students already in the list. // If the student already exists then return 0 without adding student to the list. If the student does not exist in the list then go on to add the student at the end of the list and return 1. // NOTE: You must convert the string 'schoolYear_input' to an enum type and store it in the list because the struct has enum type for school year. // Sorting should happen within the list. That is, you should not create a new linked list of students having sorted students. // Hint: One of the string library function can be useful to implement this function because the sorting needs to happen by student name which is a string. // Use swapNodes() to swap the nodes in the sorting logic
int addSort(char* studentName_input, char* major_input, char* schoolYear_input, unsigned int IDNumber_input) // 20 points { struct studentRecord* tempList = list; // work on a copy of 'list' // enter code here
return 0; // edit this line as needed }
// Q2 : displayList (10 points) // This function displays the linked list of students, with all details (struct elements). // Parse through the linked list and print the student details one after the other. See expected output screenshots in homework question file. // NOTE: School year is stored in the struct as enum type. You need to display a string for school year. void displayList() { struct studentRecord* tempList = list; // work on a copy of 'list' // enter code here
}
// Q3: countNodes (5 points) // This function counts the number of students on the linked list and returns the number. // You may use this function in other functions like deleteNode(), sortList(), addNode(), if needed. // It can helpful to call this function in other functions, but not necessary. // This function is called in main() to display number of students along with the user menu. int countNodes() {
return 0; // edit this line as needed }
// Q4 : deleteNode (10 points) // This function deletes the node specified by the student name. // Parse through the linked list and remove the node containing this student name. // NOTE: After you remove the node, make sure that your linked list is not broken. // (Hint: Visualize the list as: list......-> node1 -> node_to_remove -> node2 -> ...end. // After removing "node_to_remove", the list is broken into "list ....node1" and "node2.... end". Stitch these lists.)
int deleteNode(char* studentName_input) { struct studentRecord* tempList = list->next; // work on a copy of 'list'
return 0; // edit this line as needed }
// Q5 : swapNodes (5 points) // This function swaps the elements of parameters 'node1' and 'node2' (Here node1 does NOT mean 1st node of the list. 'node1' is just the name of the node) // This function should not alter the 'next' element of the nodes. Only then will the nodes be swapped. // Hint: The swap logic is similar to swapping two simple integer/string variables. // NOTE: This function can be used in the sorting logic in addSort()
void swapNodes(struct studentRecord* node1, struct studentRecord* node2) { }
Currently 2 student(s) on the list. Enter your selection: a: add a new student d: display student list q:removeastudentfronthelist quit Enter student name: David Luiz Enter major: cse Enter whether student is 'freshman' or "sophomore' or "ju Please enter iD number: 333 a: add a new student d: display student list r: remove a student from the list q: quit deleteNode: (see displayList() photo for list before deleting node) Currently 3 student(s) on the list. Enter your selection: a: add a new student d: display student list r : remove a student from the list q: quit a: add a new student d: display student list SchoolYear: freshman ID number: 333 Student name: William Borges Student major: CSE Schoolyear: senior ID number: 222 Currently 2 student(s) on the list. Enter your selection: a: add a new student d: display student list r : remove a student from the list q : quit
Step by Step Solution
There are 3 Steps involved in it
Step: 1
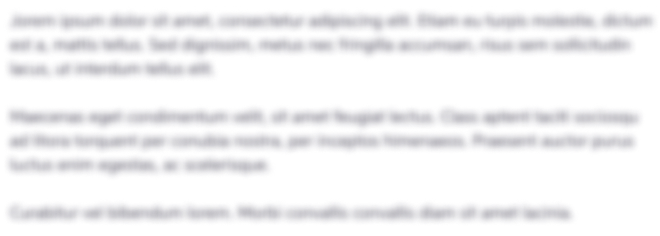
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started