Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Programming Create the following classes according the descriptions provided: Bug: The Superclass Bug has the following private attributes: String species, which is the name
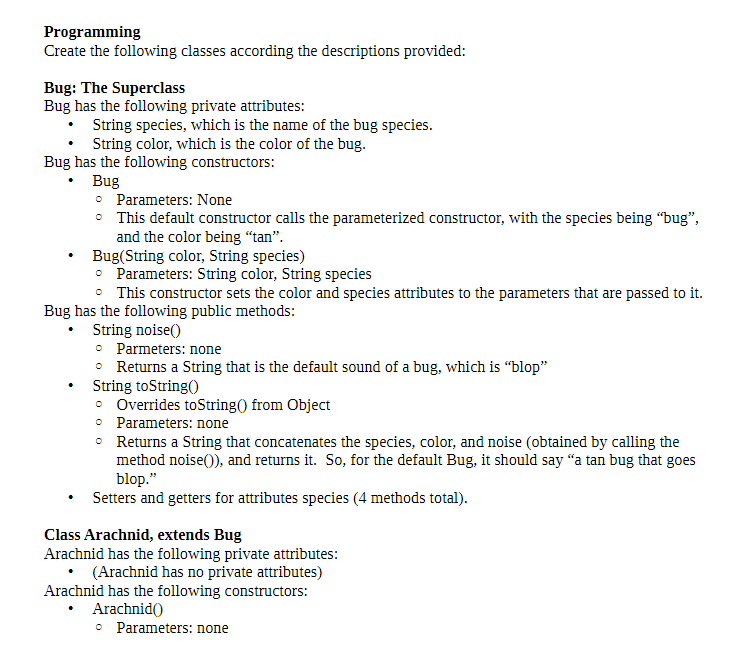
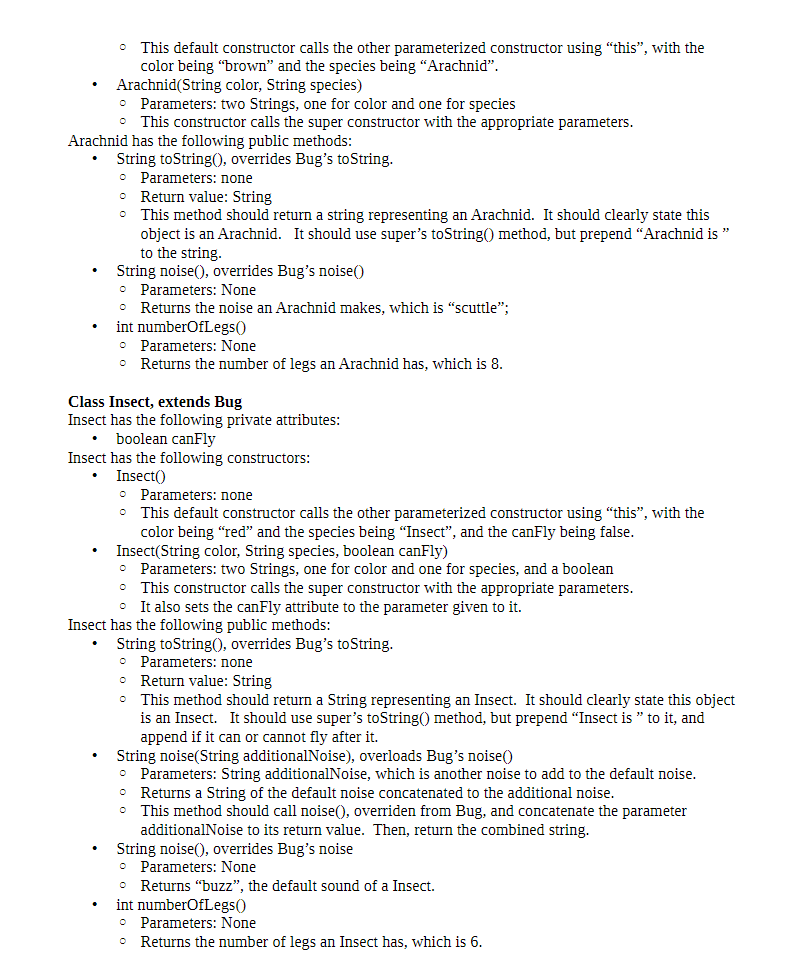
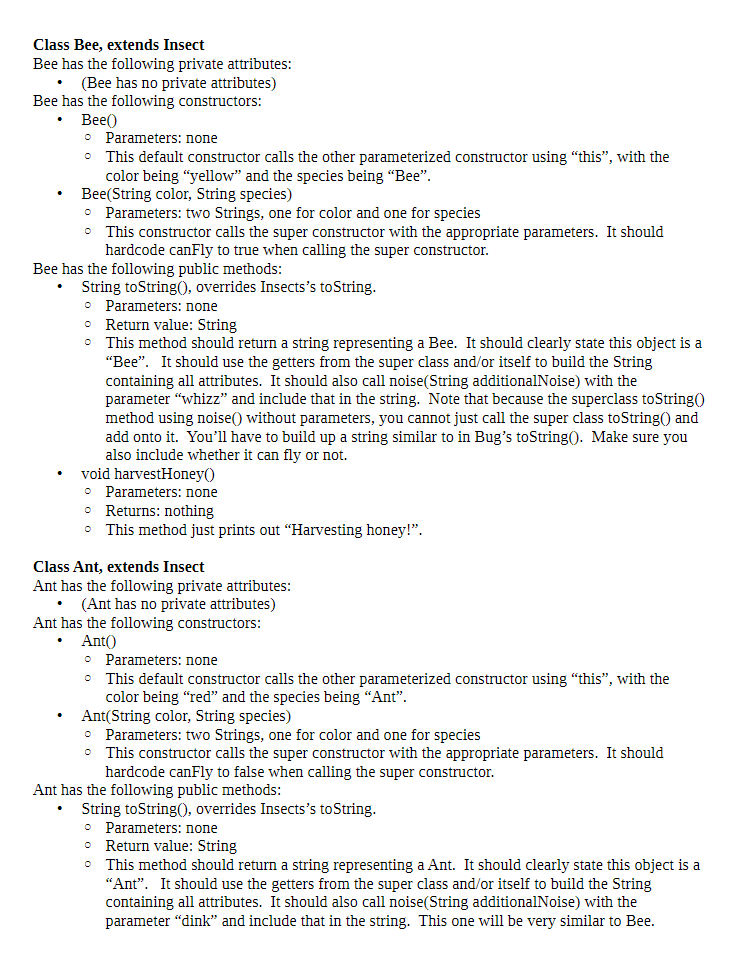
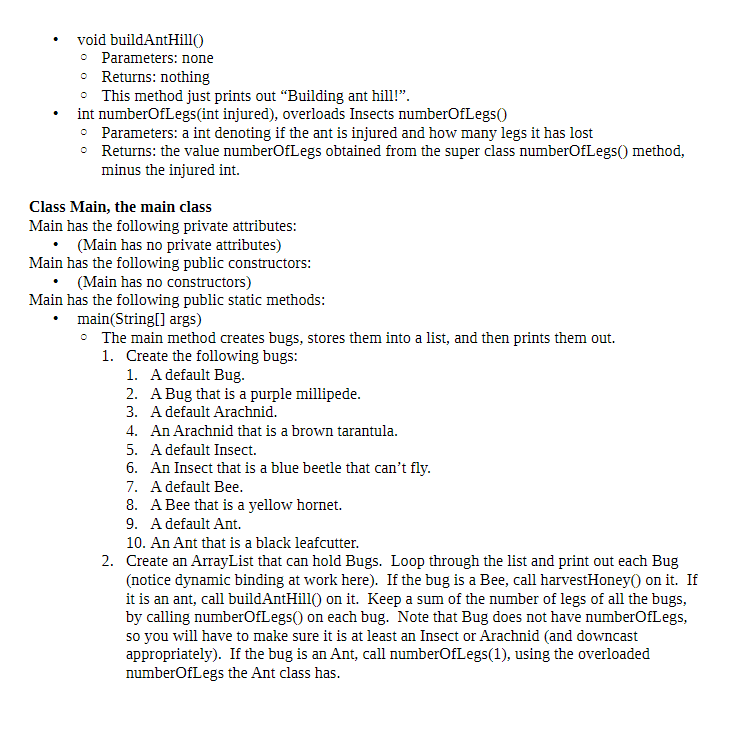
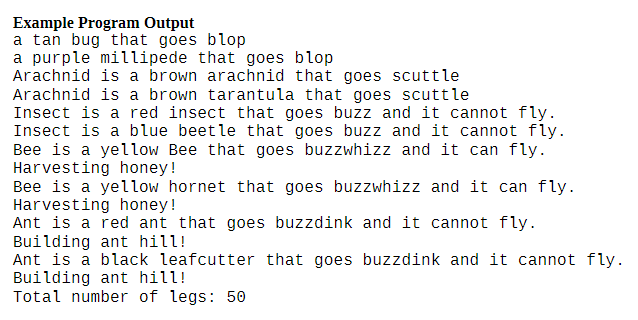
Programming Create the following classes according the descriptions provided: Bug: The Superclass Bug has the following private attributes: String species, which is the name of the bug species. String color, which is the color of the bug. Bug has the following constructors: Bug Parameters: None This default constructor calls the parameterized constructor, with the species being "bug", and the color being "tan". Bug(String color, String species) Parameters: String color, String species This constructor sets the color and species attributes to the parameters that are passed to it. Bug has the following public methods: String noise() Parmeters: none Returns a String that is the default sound of a bug, which is "blop" String to String() Overrides toString() from Object Parameters: none Returns a String that concatenates the species, color, and noise (obtained by calling the method noise()), and returns it. So, for the default Bug, it should say "a tan bug that goes blop." Setters and getters for attributes species (4 methods total). Class Arachnid, extends Bug Arachnid has the following private attributes: (Arachnid has no private attributes) Arachnid has the following constructors: Arachnid() Parameters: none This default constructor calls the other parameterized constructor using "this", with the color being "brown" and the species being "Arachnid". Arachnid(String color, String species) Parameters: two Strings, one for color and one for species 0 This constructor calls the super constructor with the appropriate parameters. Arachnid has the following public methods: String to String(), overrides Bug's to String. Parameters: none C Return value: String This method should return a string representing an Arachnid. It should clearly state this object is an Arachnid. It should use super's toString() method, but prepend "Arachnid is " to the string. String noise(), overrides Bug's noise() Parameters: None Returns the noise an Arachnid makes, which is "scuttle"; int numberOfLegs()) Parameters: None 0 Returns the number of legs an Arachnid has, which is 8. Class Insect, extends Bug Insect has the following private attributes: boolean canFly Insect has the following constructors: Insect() Parameters: none This default constructor calls the other parameterized constructor using "this", with the color being "red" and the species being "Insect", and the canFly being false. Insect(String color, String species, boolean canFly) Parameters: two Strings, one for color and one for species, and a boolean 0 This constructor calls the super constructor with the appropriate parameters. It also sets the canFly attribute to the parameter given to it. Insect has the following public methods: String toString(), overrides Bug's to String. Parameters: none Return value: String This method should return a String representing an Insect. It should clearly state this object is an Insect. It should use super's to String() method, but prepend "Insect is "to it, and append if it can or cannot fly after it. String noise(String additional Noise), overloads Bug's noise () Parameters: String additional Noise, which is another noise to add to the default noise. Returns a String of the default noise concatenated to the additional noise. This method should call noise(), overriden from Bug, and concatenate the parameter additional Noise to its return value. Then, return the combined string. String noise(), overrides Bug's noise Parameters: None Returns "buzz", the default sound of a Insect. int numberOfLegs()) Parameters: None Returns the number of legs an Insect has, which is 6. Class Bee, extends Insect Bee has the following private attributes: (Bee has no private attributes) Bee has the following constructors: Bee()) Parameters: none This default constructor calls the other parameterized constructor using "this", with the color being "yellow" and the species being "Bee". Bee(String color, String species) Parameters: two Strings, one for color and one for species This constructor calls the super constructor with the appropriate parameters. It should hardcode canFly to true when calling the super constructor. Bee has the following public methods: String to String(), overrides Insects's to String. Parameters: none Return value: String This method should return a string representing a Bee. It should clearly state this object is a "Bee". It should use the getters from the super class and/or itself to build the String containing all attributes. It should also call noise(String additional Noise) with the parameter "whizz" and include that in the string. Note that because the superclass toString() method using noise() without parameters, you cannot just call the super class toString() and add onto it. You'll have to build up a string similar to in Bug's toString(). Make sure you also include whether it can fly or not. void harvestHoney() Parameters: none Returns: nothing This method just prints out "Harvesting honey!". Class Ant, extends Insect Ant has the following private attributes: (Ant has no private attributes) Ant has the following constructors: Ant() Parameters: none This default constructor calls the other parameterized constructor using "this", with the color being "red" and the species being "Ant". Ant(String color, String species) Parameters: two Strings, one for color and one for species This constructor calls the super constructor with the appropriate parameters. It should hardcode canFly to false when calling the super constructor. Ant has the following public methods: String to String(), overrides Insects's to String. Parameters: none Return value: String This method should return a string representing a Ant. It should clearly state this object is a "Ant". It should use the getters from the super class and/or itself to build the String containing all attributes. It should also call noise(String additionalNoise) with the parameter "dink" and include that in the string. This one will be very similar to Bee. void buildAntHill() Parameters: none Returns: nothing This method just prints out "Building ant hill!". int numberOfLegs (int injured), overloads Insects numberOfLegs() Parameters: a int denoting if the ant is injured and how many legs it has lost Returns: the value numberOfLegs obtained from the super class numberOfLegs() method, minus the injured int. Class Main, the main class Main has the following private attributes: (Main has no private attributes) Main has the following public constructors: (Main has no constructors) Main has the following public static methods: main(String[] args) The main method creates bugs, stores them into a list, and then prints them out. 1. Create the following bugs: 1. A default Bug. 2. A Bug that is a purple millipede. 3. A default Arachnid. 4. An Arachnid that is a brown tarantula. 5. A default Insect. 6. An Insect that is a blue beetle that can't fly. 7. A default Bee. 8. A Bee that is a yellow hornet. 9. A default Ant. 10. An Ant that is a black leafcutter. 2. Create an ArrayList that can hold Bugs. Loop through the list and print out each Bug (notice dynamic binding at work here). If the bug is a Bee, call harvestHoney) on it. If it is an ant, call buildAntHill() on it. Keep a sum of the number of legs of all the bugs, by calling numberOfLegs() on each bug. Note that Bug does not have numberOfLegs, so you will have to make sure it is at least an Insect or Arachnid (and downcast appropriately). If the bug is an Ant, call numberOfLegs (1), using the overloaded numberOfLegs the Ant class has. Example Program Output a tan bug that goes blop a purple millipede that goes blop Arachnid is a brown arachnid that goes scuttle Arachnid is a brown tarantula that goes scuttle Insect is a red insect that goes buzz and it cannot fly. Insect is a blue beetle that goes buzz and it cannot fly. Bee is a yellow Bee that goes buzzwhizz and it can fly. Harvesting honey! Bee is a yellow hornet that goes buzzwhizz and it can fly. Harvesting honey! Ant is a red ant that goes buzzdink and it cannot fly. Building ant hill! Ant is a black leafcutter that goes buzzdink and it cannot fly. Building ant hill! Total number of legs: 50 Programming Create the following classes according the descriptions provided: Bug: The Superclass Bug has the following private attributes: String species, which is the name of the bug species. String color, which is the color of the bug. Bug has the following constructors: Bug Parameters: None This default constructor calls the parameterized constructor, with the species being "bug", and the color being "tan". Bug(String color, String species) Parameters: String color, String species This constructor sets the color and species attributes to the parameters that are passed to it. Bug has the following public methods: String noise() Parmeters: none Returns a String that is the default sound of a bug, which is "blop" String toString() Overrides to String() from Object Parameters: none Returns a String that concatenates the species, color, and noise (obtained by calling the method noise()), and returns it. So, for the default Bug, it should say "a tan bug that goes blop." Setters and getters for attributes species (4 methods total). Class Arachnid, extends Bug Arachnid has the following private attributes: (Arachnid has no private attributes) Arachnid has the following constructors: Arachnid()) Parameters: none This default constructor calls the other parameterized constructor using "this", with the color being "brown" and the species being "Arachnid". Arachnid(String color, String species) Parameters: two Strings, one for color and one for species 0 This constructor calls the super constructor with the appropriate parameters. Arachnid has the following public methods: String toString(), overrides Bug's to String. Parameters: none Return value: String This method should return a string representing an Arachnid. It should clearly state this object is an Arachnid. It should use super's toString() method, but prepend "Arachnid is " to the string. C String noise(), overrides Bug's noise() Parameters: None Returns the noise an Arachnid makes, which is "scuttle"; int numberOfLegs()) Parameters: None 0 Returns the number of legs an Arachnid has, which is 8. Class Insect, extends Bug Insect has the following private attributes: boolean canFly Insect has the following constructors: Insect() Parameters: none This default constructor calls the other parameterized constructor using "this", with the color being "red" and the species being "Insect", and the canFly being false. Insect(String color, String species, boolean canFly) Parameters: two Strings, one for color and one for species, and a boolean 0 This constructor calls the super constructor with the appropriate parameters. It also sets the canFly attribute to the parameter given to it. Insect has the following public methods: String to String(), overrides Bug's to String. Parameters: none Return value: String This method should return a String representing an Insect. It should clearly state this object is an Insect. It should use super's toString() method, but prepend "Insect is "to it, and append if it can or cannot fly after it. String noise (String additional Noise), overloads Bug's noise () Parameters: String additional Noise, which is another noise to add to the default noise. Returns a String of the default noise concatenated to the additional noise. This method should call noise(), overriden from Bug, and concatenate the parameter additional Noise to its return value. Then, return the combined string. String noise(), overrides Bug's noise Parameters: None Returns "buzz", the default sound of a Insect. int numberOfLegs () Parameters: None Returns the number of legs an Insect has, which is 6. Class Bee, extends Insect Bee has the following private attributes: (Bee has no private attributes) Bee has the following constructors: Bee()) Parameters: none This default constructor calls the other parameterized constructor using "this", with the color being "yellow" and the species being "Bee". Bee(String color, String species) Parameters: two Strings, one for color and one for species This constructor calls the super constructor with the appropriate parameters. It should hardcode canFly to true when calling the super constructor. Bee has the following public methods: String to String(), overrides Insects's to String. Parameters: none Return value: String This method should return a string representing a Bee. It should clearly state this object is a "Bee". It should use the getters from the super class and/or itself to build the String containing all attributes. It should also call noise(String additional Noise) with the parameter "whizz" and include that in the string. Note that because the superclass toString() method using noise() without parameters, you cannot just call the super class toString() and add onto it. You'll have to build up a string similar to in Bug's toString(). Make sure you also include whether it can fly or not. void harvestHoney() Parameters: none Returns: nothing This method just prints out "Harvesting honey!". Class Ant, extends Insect Ant has the following private attributes: (Ant has no private attributes) Ant has the following constructors: Ant() Parameters: none This default constructor calls the other parameterized constructor using "this", with the color being "red" and the species being "Ant". Ant(String color, String species) Parameters: two Strings, one for color and one for species This constructor calls the super constructor with the appropriate parameters. It should hardcode canFly to false when calling the super constructor. Ant has the following public methods: String to String(), overrides Insects's to String. Parameters: none Return value: String This method should return a string representing a Ant. It should clearly state this object is a "Ant". It should use the getters from the super class and/or itself to build the String containing all attributes. It should also call noise(String additionalNoise) with the parameter "dink" and include that in the string. This one will be very similar to Bee. void buildAntHill() Parameters: none Returns: nothing This method just prints out "Building ant hill!". int numberOfLegs (int injured), overloads Insects numberOfLegs () Parameters: a int denoting if the ant is injured and how many legs it has lost Returns: the value numberOf Legs obtained from the super class numberOfLegs() method, minus the injured int. Class Main, the main class Main has the following private attributes: (Main has no private attributes) Main has the following public constructors: (Main has no constructors) Main has the following public static methods: main (String[] args) The main method creates bugs, stores them into a list, and then prints them out. 1. Create the following bugs: 1. A default Bug. 2. A Bug that is a purple millipede. 3. A default Arachnid. 4. An Arachnid that is a brown tarantula. 5. A default Insect. 6. An Insect that is a blue beetle that can't fly. 7. A default Bee. 8. A Bee that is a yellow hornet. 9. A default Ant. 10. An Ant that is a black leafcutter. 2. Create an ArrayList that can hold Bugs. Loop through the list and print out each Bug (notice dynamic binding at work here). If the bug is a Bee, call harvestHoney() on it. If it is an ant, call buildAntHill() on it. Keep a sum of the number of legs of all the bugs, by calling numberOfLegs() on each bug. Note that Bug does not have numberOfLegs, so you will have to make sure it is at least an Insect or Arachnid (and downcast appropriately). If the bug is an Ant, call numberOfLegs (1), using the overloaded numberOfLegs the Ant class has. Example Program Output a tan bug that goes blop a purple millipede that goes blop Arachnid is a brown arachnid that goes scuttle Arachnid is a brown tarantula that goes scuttle Insect is a red insect that goes buzz and it cannot fly. Insect is a blue beetle that goes buzz and it cannot fly. Bee is a yellow Bee that goes buzzwhizz and it can fly. Harvesting honey! Bee is a yellow hornet that goes buzzwhizz and it can fly. Harvesting honey! Ant is a red ant that goes buzzdink and it cannot fly. Building ant hill! Ant is a black leafcutter that goes buzzdink and it cannot fly. Building ant hill! Total number of legs: 50
Step by Step Solution
There are 3 Steps involved in it
Step: 1
import javautilArrayList class Bug private String species private String color public Bug thistan bug public BugString color String species thiscolor ...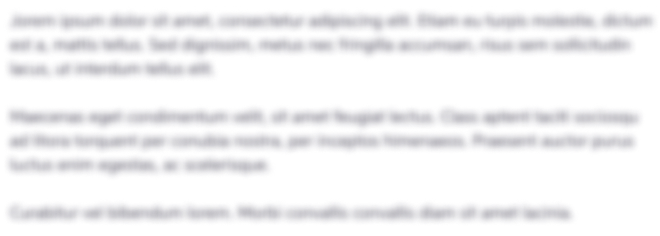
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started