Question
Programming in C. A simple bubble sort algorithm is attached for your reference. Remember in your code, you have to open 2 files: an input
Programming in C. A simple bubble sort algorithm is attached for your reference. Remember in your code, you have to open 2 files: an input file to read "Lab3.dat" data, and an output file to write your sorted array. The Lab3.dat file contains exactly 100 elements in random order, your task is to sort them in ascending order - smallest to greatest. Write a program to open and read from a file (attached Lab3.dat), sort the data in ascending order (smallest to largest), and write the sorted data into an output file. Attached simple bubble sort functions. /* C program for implementation of Bubble sort */ #include void swap(int *xp, int *yp) { int temp = *xp; *xp = *yp; *yp = temp; } /* A function to implement bubble sort */ void bubbleSort(int arr[], int n) { int i, j; for (i = 0; i < n-1; i++) /* Last i elements are already in place */ for (j = 0; j < n-i-1; j++) if (arr[j] > arr[j+1]) swap(&arr[j], &arr[j+1]); } /* Function to print an array */ void printArray(int arr[], int size) { int i; for (i=0; i < size; i++) printf("%d ", arr[i]); printf("n"); } /* Main program to test above functions */ int main(void) { int arr[] = {64, 34, 25, 12, 22, 11, 90}; int n = sizeof(arr)/sizeof(arr[0]); bubbleSort(arr, n); printf("Sorted array: "); printArray(arr, n); return 0; }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
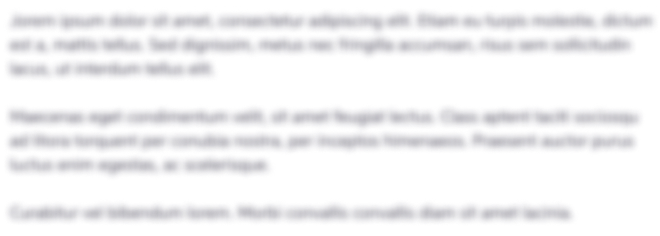
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started