Question
Programming language: C++ Scenario: Image processing is an interesting field of computer engineering and signal processing that analyses and summarizes video and image content. The
Programming language: C++
Scenario: Image processing is an interesting field of computer engineering and signal processing that analyses and summarizes video and image content. The field was initially led by NASA scientists to analyze imagery coming from space probes. That interest in space exploration still holds true for today. See: https://mars.nasa.gov/resources/25622/perseverance-navcams-360-degree-panorama/In this assignment we shall briefly explore this field. A grayscale image consists of pixels (intensity values) arranged in 2 dimensional grid format where each pixel can have a value between 0 and 255 where values closer to 0 are dark intensities and values closer to 255 are bright intensities. The sample text file, imageA3gives is an example of a 16 x 16 image. The actual image is shown to the right.
Unfortunately, in transmitting the original text file over the internet, intensity data was corrupted and represented as NaNs in the file. See attached text file imageA3.txt.
This is the contents of the text file:
160 160 NaN 160 128 96 64 32 0 0 0 0 0 0 NaN 0 160 192 192 192 160 128 96 64 32 0 0 0 0 0 0 0 NaN 192 224 224 NaN 160 128 96 NaN 32 0 0 0 NaN 0 0 160 NaN 224 256 224 192 160 128 96 64 32 0 0 0 0 0 128 160 NaN 224 256 224 NaN 160 128 96 64 32 0 0 NaN 0 96 128 160 192 224 256 224 192 160 128 NaN 64 32 0 0 0 NaN 96 128 NaN 192 224 256 224 192 160 128 96 64 32 0 0 32 64 96 128 160 192 NaN 256 NaN 192 160 128 96 64 32 0 0 32 64 96 128 160 NaN 224 256 224 192 160 128 96 64 32 0 0 NaN 64 NaN 128 160 192 224 256 NaN 192 NaN 128 96 64 0 0 0 32 64 96 128 160 192 224 256 224 NaN 160 128 NaN 0 0 0 0 32 64 96 128 160 192 224 256 224 192 160 128 0 0 0 0 0 32 64 96 NaN 160 192 224 256 224 192 160 0 NaN 0 0 0 0 32 64 96 128 160 192 224 256 224 192 0 0 0 0 0 0 0 32 64 96 128 NaN 192 NaN 256 224 0 0 0 0 0 0 0 0 NaN 64 96 128 160 192 224 255
Part 1: Signal Conditioning / Data Cleaning
Design (flow diagram) and implement a C++ program to (i) read in the corrupted file pixel by pixel, (ii) correct (clean) the corrupted pixel values and (iii) write the cleaned image to a new file. (Give the completed code for this section.) i. Write a function to read the pixel value as a string. Write a sub-function called isNaN to check if it is a NaN. ii. If the pixel intensity value is a NaN, write a sub-function called cleanImagetoclean the corrupted data to an appropriate value based on neighboring pixels values. Store the clean image data into a vector. There is no need to store data into a 2 dimensional array or vector for this assignment. iii. Unfortunately, the .txt format is not an image format. Image formats come with additional header information and cannot be opened with a text editor. Netpbm offers a simplistic alternative for transmitting image data. The portable pixmap format (PPM), the portable graymap format (PGM) and the portable bitmap format (PBM) are image file formats designed to be easily exchanged between platforms. You are required to write the clean image data into a new .pgm file. You would need to automatically determine the number of columns and rows of pixels (16 x 16 in this case) as well as the maximum pixel value (255 in this case). See: http:/etpbm.sourceforge.net/doc/pgm.htmlfor details of this format. Use: https://www.kylepaulsen.com/stuff/NetpbmViewer/to render / view your file.
Part 2: Statistics
In addition to conforming to the proper .pgm format you are required to find the median value as an additional statistic of this image and embed as a line of comment in the .pgm format. For example:# Median = 96. This question requires the data to be sorted to find the median. Design (flow diagram) and write a function to sort the data in ascending order. You cannot use the STL sort function.
Part 3: Histogram Based Thresholding
Thresholding is a simple and easy way to segment an image into separate objects. Using a histogram method, we can segment this image into several classes based on intensity (pixel) values. Pixel values lie within a range of 0 to 255. We can categorize these intensities into the following histogram ranges:
Class 1, Very Bright: 192 to 255
Class 2, Bright: 128 to 191
Class 3, Dark: 64 to 127
Class 4, Very Dark: 0 to 63
Write a function to relabel the image pixel values to one of the four classes [1,2,3,4] (integer values). Write this segmented image into a new .pgm file with the histogram frequency values embedded as lines of comment.
imageA3 - Notepad 32 0 @ @ @ 0 File Edit Format View Help 160 160 160 160 160 192 192 192 160 192 224 224 160 192 224 256 128 160 192 224 96 128 160 192 64 96 128 160 32 64 96 128 32 64 96 32 64 @ 32 @ 12B 160 192 224 256 224 192 160 128 96 64 32 96 128 160 192 224 256 224 192 160 128 96 64 32 64 96 128 160 192 224 256 224 192 160 96 128 160 192 224 256 224 192 160 128 96 64 32 32 64 96 128 16e 192 224 256 224 192 160 128 96 64 32 32 64 96 128 160 192 224 256 32 64 96 128 160 192 224 256 224 192 160 32 64 96 128 160 192 224 256 224 192 160 128 128 224 32 64 96 128 160 192 224 256 224 192 160 32 64 96 128 160 192 224 256 224 192 32 64 96 128 160 192 96 64 32 32 64 96 128 160 192 224 256 224 192 160 128 96 64 @ @ 128 224 96 255 imageA3 - Notepad 32 0 @ @ @ 0 File Edit Format View Help 160 160 160 160 160 192 192 192 160 192 224 224 160 192 224 256 128 160 192 224 96 128 160 192 64 96 128 160 32 64 96 128 32 64 96 32 64 @ 32 @ 12B 160 192 224 256 224 192 160 128 96 64 32 96 128 160 192 224 256 224 192 160 128 96 64 32 64 96 128 160 192 224 256 224 192 160 96 128 160 192 224 256 224 192 160 128 96 64 32 32 64 96 128 16e 192 224 256 224 192 160 128 96 64 32 32 64 96 128 160 192 224 256 32 64 96 128 160 192 224 256 224 192 160 32 64 96 128 160 192 224 256 224 192 160 128 128 224 32 64 96 128 160 192 224 256 224 192 160 32 64 96 128 160 192 224 256 224 192 32 64 96 128 160 192 96 64 32 32 64 96 128 160 192 224 256 224 192 160 128 96 64 @ @ 128 224 96 255Step by Step Solution
There are 3 Steps involved in it
Step: 1
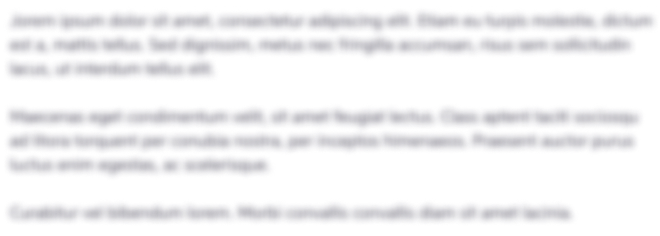
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started