Question
Programming Language is Java Implement a MaxHeap and use it to simulate an Emergency Room as discussed in class Th Feb 14 (week 5). Provide
Programming Language is Java
Implement a MaxHeap and use it to simulate an Emergency Room as discussed in class Th Feb 14 (week 5). Provide testing classes for each class.
Patient arrival is simulated with one input file containing records of patient arrival time, urgency (priority), and an estimated treatment time. Each patient is assigned an urgency number in 1~10 (priority level, 1 is lowest/least urgent) and an estimated treatment time in minutes (Like need 20 minutes). Patients are seen based on their urgency. Patients with a same urgency are seen following their arrival order (first come first serve). For simplicity, there is only one doctor. Assume no two patients arrive at the same time.
The HW zip file contains the following:
- ER.java: incomplete. Need to add code into run()
- Patient.java: incomplete. Need to add accessors, overload toString() (if you want to print a patient object for testing), and overload compareTo()
- patientRecords.text: one example data file
Unless to add package statement(s) and additional imports, you should not modify the given Java files in other unspecified ways. Check with the instructor when youre unsure.
Your MaxHeap class must be defined as a generic class:
public class MaxHeapextends Comparable> {
private T[] heap; // heap array
...
}
// ER.java (shell) // CS451 // ER class for ER simulation. Holds ER data and simulation code. // Simulates arrival and treatment of ER patients with one single doctor.
//java.io classes, used for file i/o import java.io.BufferedReader; import java.io.FileReader; import java.io.IOException;
import java.util.ArrayList; import java.util.Scanner; // I/O methods
public class ER { private ArrayList rawRecords; // hold raw patient records read from file private MaxHeap patientQueue; // patients that are queued
/** * Initialize the simulation by loading patient data from file * * @param patientFileName name of file holding patient data */ public ER(String patientFileName) { rawRecords = loadFromFile(patientFileName); if ( rawRecords.size()>0 ) { Patient[] recordsArr = new Patient[rawRecords.size()]; patientQueue = new MaxHeap(recordsArr, 0, rawRecords.size()); // an empty heap with size spots } }
/** * Load data from file * * @param fileName name of file holding patient data * @return an ArrayList of Patient objects read out of the file */ private ArrayList loadFromFile(String fileName) { ArrayList records = new ArrayList<>();
// open file Scanner fileIn = null; // scanner object to connect to file
try { // open input file fileIn = new Scanner(new BufferedReader(new FileReader(fileName)));
// skip first line (record headings) fileIn.nextLine(); // read one line, but do not use
// loop through multiple records while (fileIn.hasNext()) { // (col number starts from 1) // 1st col: arrival time // 2nd col: priority // 3rd col: treatmentTime
// 1. read one record containing all columns int arrivalTime = fileIn.nextInt(); int priority = fileIn.nextInt(); int treatmentTime = fileIn.nextInt();
// 2. create Patient object and add to ArrayList records records.add(new Patient(arrivalTime, priority, treatmentTime));
// end one record }// end while: reading all records
} catch (IOException ioe) { log("Error reading \"" + fileName+ "\" file: " + ioe); } finally // close file { if ( fileIn != null) { // close if was connected to a file fileIn.close(); } } // end file input
return records; } // end loadFromFile
/** * Runs the simulation of patients arriving, being treated, and exiting */ public void run() { if (rawRecords == null || rawRecords.size()==0) { log("Empty paitent list. End of simulation."); return; }
/* // help test the basic setup log("# of patient records loaded: " + rawRecords.size()); for (Object p : rawRecords) { log(((Patient) p).toString()); }*/
// ADD CODE
} // end run
/** * A helper method to display information on screen * * @param str Message to be displayed */ private void log(String str) { System.out.println(str); }
} // end class ER
// Patient.java (shell) // CS451 // Patient class for ER simulation. // Simulates arrival and treatment of ER patients with one single doctor.
public class Patient implements Comparable{ private static int nextID = 1; // id seed. Used to generate an unique id starting from 1 private int id; private int arrivalTime; private int priority; private int treatmentTime;
/** * Generate an unique id that is 1 larger than the last id generated. * The first id should be 1. * * @return The generated ID */ private static int getID() { return nextID++; }
public Patient(int arrivalTime, int priority, int treatmentTime) { this.id = getID(); this.arrivalTime = arrivalTime; this.priority = priority; this.treatmentTime = treatmentTime; }
// ADD
} // end class Patient
Step by Step Solution
There are 3 Steps involved in it
Step: 1
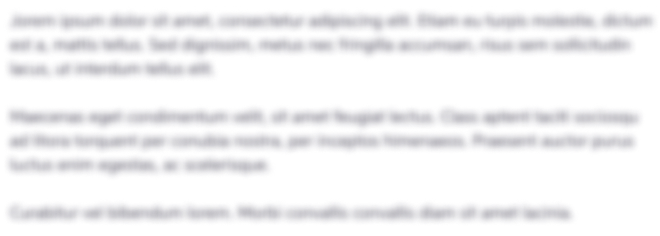
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started