Question
PROGRAMMING LANGUAGE: Python 3.8 For this assignment, you'll be reading grade data from a file, determining student letter grades, and writing the results to a
PROGRAMMING LANGUAGE: Python 3.8
For this assignment, you'll be reading grade data from a file, determining student letter grades, and writing the results to a new file.
Input Data. A sample input data file of the type that your program should process is available here: sample_grade_input.txt. This sample file contains fake grade data for 14 students. However, your program should be able to work with a file with an arbitrary number of students. For example, I may test your program with just 3 students, or with 300. Your program should work for any length file.
Each student record spans 3 lines in the data file. The first line contains the type of student: GRAD or UNDERGRAD. This is important because graduate and undergraduate students get graded differently at X. The second line contains the student's name. The third line contains the student's overall numerical grade. Your program will need to map these to letter grades according to the following rules:
For the sake of this assignment, GRAD student grades are defined as follows:
Number Range | Letter Grade |
---|---|
95-100 | H |
80-94 | P |
70-79 | L |
0-69 | F |
For the sake of this assignment, UNDERGRAD student grades are defined as follows:
Number Range | Letter Grade |
---|---|
90-100 | A |
80-89 | B |
70-79 | C |
60-69 | D |
0-59 | F |
Output Data. Your program should write the results of its computations to an output file. An example of the required output file can be found here: sample_grade_output.txt. This sample file contains fake grade data for 14 students. Each student record spans 2 lines in the data file. The first line contains the student name. The second line contains the student's letter grade.
Basic Requirements
Satisfying all basic requirements perfectly, with no points deducted for any reason, would earn a maximum score of 8 out of 10 for this assignment.
Your program should perform the following:
- Prompt the user to enter the name of the input file, making sure that the file exists and asking the user to re-enter a filename if needed.
- Prompt the user to enter the name of an output file. The output file should be erased/overwritten if an old one with the same name exists.
- Read the input file, assign grades as appropriate for the type of student (GRAD vs. UNDERGRAD), and write the output to file.
- Gracefully handle errors in the input file. In particular, your program should catch errors such as invalid numbers for grades, or student categories that are not "GRAD" or "UNDERGRAD". If found, the program should display an error message telling the user what the problem was. The program should then stop processing the rest of the data file and tell the user to fix the problems before retrying. During grading, your program will be tested with input files that have had errors intentionally introduced. It is suggested that you try this in your own testing to make sure that your program works as expected.
Two kinds of output should be produced by your program. First, an output data file should be created using the same format described in the "Output Data" paragraph of the Overview section above. Second, your program will produce output on the console in the form of status messages and prompts for user input. The output produced by your program should be nearly identical to the sample output provided below. While the specific data (names, grades, etc.) will vary based on the input provided, the data formats, prompts, and messages printed to the console when using your program should match those in the sample output files to receive full credit.
Please Note: Your program should use the flow control and conditional constructs that have been discussed in class to "stop processing the rest of the data file." In this way, the program should continue to run until the end, with your logic telling the program what to do (or what not to do). You should NOT use any special Python functions to "immediately stop" your program (e.g., using sys.exit() or a similar function is not allowed).
Advanced Requirements
Satisfying both the basic and advanced requirements perfectly, with no points deducted for any reason, will result in a full 10 out of 10 score for this assignment.
Expand on the basic requirements by extending your program as follows.
- Allow the user if they wish to subject the grades to a curve prior to grading.
- If the user declines to curve the grades, the program should proceed as outlined in the Basic Requirements.
- If the user chooses to curve the grades, the program should ask the user to enter the number grade that should be treated as a "100" for grading purposes.
- Once the should enters a number, the program should use this to curve the grades using linear interpolation. For example:
- If a user enters "50" as the score to use for the curve, then the following "curved grades" should be used when assigning letters:
- 25 becomes 50
- 50 becomes 100
- 100 becomes 200
- If a user enters "80" as the score to use for the curve, then the following "curved grades" should be used when assigning letters:
- 40 becomes 50
- 80 becomes 100
- 100 becomes 125
- If a user enters "50" as the score to use for the curve, then the following "curved grades" should be used when assigning letters:
- Pay attention to possible exceptions. Make sure that your code for this feature is robust.
Sample Output
An example of the output produced by my solution to this assignment can be found here. When the user elects to curve the grades, the output looks like this. When an error in the data file is detected, the output looks like this.
The above files all show what is shown to the user on the screen. There is also the output data file, of course, which contains the assigned letter grades if no error occurs. An example of the data output file is available here.
HERE ARE THE SAMPLE OUTPUTS:
Step by Step Solution
There are 3 Steps involved in it
Step: 1
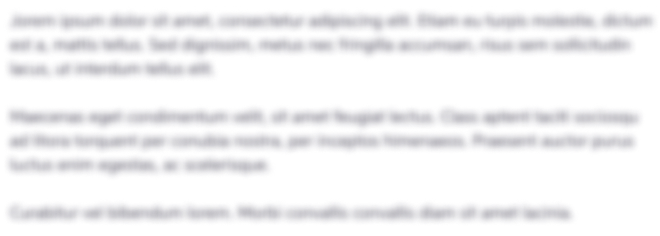
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started