Question
Project 1: Implementing the Set Class using a List class Implement the functionalities of the Set Class, as given below, in Java by using a
Project 1: Implementing the Set Class using a List class Implement the functionalities of the Set Class, as given below, in Java by using a private data member of type ListReferenceBased
Constructors: a default constructor, a constructor that takes a single argument of type E and initializing the set to contain that object, and a copy constructor,
setSize: a method that returns the number of elements in the set as an int value,
print: a method that prints out the contents of the set,
insert: a method that takes an element of type E and inserts it into the set,
arrayInsert: a method that takes an array of elements of type E and inserts them into the set,
union: a method that takes a Set as an argument and returns a new Set that is the union of the current set and the argument,
intersection: a method that takes a Set as an argument and returns a new Set that is the intersection of the current set and the argument,
dierence: a method that takes a Set as an argument and returns a new Set that is the dierence of the current set and the argument,
in: a method that takes an element of type E and returns a boolean value depending on whether the given E is contained in the current set or not.
You are required to provide comments in javadoc format for each method and the class variables.
public class SetTest { // Simple main function to test ADT Set public static void main(String[] args) { Integer[] M ={2,3,5,7,11,13,17,19,23}; Integer[] N = {1,3,6,8,9,11,12,17,19,23,24,25,3}; Set
Set
Set
Set
/** class Node for the implementation of simple linked list. The node * contains only an item and a reference to the following Node*/
public class Node
private E item; private Node
public Node(E newItem) { item = newItem; next = null; } // end constructor
public Node(E newItem, Node
public void setItem(E newItem) { item = newItem; } // end setItem
public E getItem() { return item; } // end getItem public void setNext(Node
public Node
} // end class Node
* Reference-based implementation of ADT list. * Modify the code to implement a doubly linked list.
import java.util.Iterator;
public class ListReferenceBased
private Node
public ListReferenceBased(ListReferenceBased
/** Tests if this list has no elements. * @return true
if this list has no elements; * false
otherwise. */public boolean isEmpty() {
return numItems == 0; } // end isEmpty
public int size() { return numItems; } // end size
private Node
public E get(int index) throws ListIndexOutOfBoundsException { if (index >= 1 && index <= numItems) { // get reference to node, then data in node Node
public void append(E item) { add(numItems+1, item); }//end append
public void remove(int index) throws ListIndexOutOfBoundsException { if (index >= 1 && index <= numItems) { if (index == 1) { // delete the first node from the list head = head.getNext(); } else{ Node
public void delete(E item){ int index = contains(item); if (index == -1) System.out.println("Try to delete " + item + " but it is not in the list"); else remove(index); } public int contains(E elt){ Node
public ListReferenceBasedIterator
public class ListReferenceBasedIterator
public ListReferenceBasedIterator(Node
public E next(){ E elt = current.getItem(); current = current.getNext(); return elt;}
public void remove(){} }
} // end ListReferenceBased
public interface BasicListInterface
public boolean isEmpty(); public int size(); public void removeAll();
public E get(int index) throws ListIndexOutOfBoundsException;
} // end BasicListInterface
----------------------------------------------------------------------------
/** * Interface ListInterface for the ADT list. */
public interface ListInterface
public void append(E elt);
public void remove(int index) throws ListIndexOutOfBoundsException; public void delete(E elt);
public int contains(E elt);
public void display();
} // end ListInterface
------------------------------------------------------------------------------------------
public class ListException extends RuntimeException { public ListException(String s) { super(s); } // end constructor } // end ListException
----------------------------------------------------------------------------------------------
public class ListIndexOutOfBoundsException extends IndexOutOfBoundsException { public ListIndexOutOfBoundsException(String s) { super(s); } // end constructor } // end ListIndexOutOfBoundsException
Step by Step Solution
There are 3 Steps involved in it
Step: 1
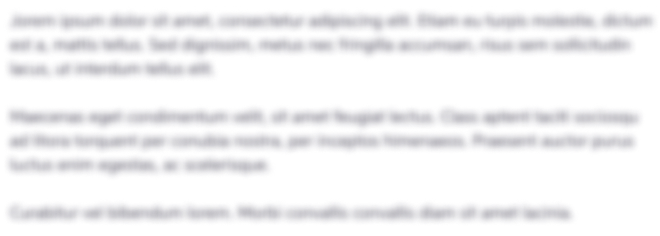
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started