Question
Project 1a: Amazon Customer Order History System Congratulations! After a months-long, rigorous, and very competitive screening process, Amazon,com has selected you as a new Engineer
Project 1a: Amazon Customer Order History System
Congratulations! After a months-long, rigorous, and very competitive screening process, Amazon,com has selected you as a new Engineer in their Prime Divison to help update their Customer Order History and Invoice tracking system (COHI). Your job is to come up with a clean object-oriented design using reusable Java classes for printing order detail reports and customer invoices. Here are real examples of each. (As it turns out, Amazon is using a recent summer reading book order for my kids, for your training support):
Sample Actual Amazon Order-Detail History
AmazonOrderDetails.docx
Sample Actual Amazon Invoice
AmazonInvoice.docx
Your new Amazon supervisor wants you to prototype an abbreviated, but re`alistic object-oriented design to support a list of products, orders, order details, shipping records, invoices, and customers. Your system should contain at least one customer, one order, five products, five order details, one shipping record, and one payment transaction to print an order details list and invoice as shown above. The product names need not be the same as above.
Below is a class diagram that models the class attributes that will allow you to support this system.
Sales Order System.pdf
You should create classes to implement this design.
Guidelines:
- Use Data Encapsulation. All class attributes should be accessed through either constructors or getters and setters. Most attributes should be set using constructors. All attributes that need to be read from your class objects should be accessed with getters. IntelliJ IDEA supports code generation for this purpose. After you implement classes with the class attributes defined, press Alt-Insert in your class file, and IntelliJ IDEA will present you with an options dialog to generate constructors, toString() methods, getters, and setters for your classes. This is a powerful feature. I suggest generating only getters.
- Java class files can contain multiple classes, or you can segregate your classes into separate files. If you choose to use multiple classes per java file, that is inner classes, only the main class can be designated public, while the others must be default or private.
- Your implementations generally do not need for, and should not include any global variables, with the possible exception of constants.
- Your system, as in real-life, will generate an order, and only later ship the order, and generate a payment. See the sample main method provided below for the order of operations.
- Your Order Details print-out should obtain its data only through the object model. No hard coding is permitted in the print-outs.
- Note that Order and OrderDetails are separate objects but an order contains a list (such as an ArrayList) of OrderDetails. Lab 2 shows you how to create ArrayLists of Java Objects.
Simplifications:
- For simplicity, you can assume that all order items are shipped together in one shipment and paid for in one transaction, though in real-life this is not the case.
- Similarly, orders are paid for in a single transaction, while in real-life Amazon permits multiple payments and shipments per order.
- Again, since we assume only one payment per order, we will generate only one invoice per order.
- Finally, your system will not need to contain many millions of products, rather only five. Lucky you, that you don't have to type in all these millions of product information records.
Class Diagram Model for System (Critical! SEE THE DIAGRAM BELOW!):
Sales Order System-1.pdf
Sample Simplified Order Details Output:
************* Order Details ************* Ordered on May 21, 2020 Order # 114-4625135-4373821 Shipping Address
Michael Whitehead 1298 Hares Hill Road Kimberton, PA 19442 United States
Payment Method
Amazon.com Visa **** 7744
Order Summary
Item(s) Subtotal: $34.85 Shipping and Handling $7.25 Total before tax: $42.10 Estimated tax to be collected: $2.53 Grand Total: $44.63
Delivered May 22, 2020
The Pirate's Coin: A Sixty-Eight Rooms Adventure (The Sixty-Eight Rooms Adventures), Malone, Marianne Sold by: Amazon.com Services LLC Sold by: 7.99 Condition: New Pax, Pennypacker, Sara Sold by: Amazon.com Services LLC Sold by: 5.89 Condition: New The River (A Hatchet Adventure) Paulsen, Gary Sold by: Amazon.com Services LLC Sold by: 8.49 Condition: New Brian's Return (A Hatchet Adventure) Sold by: Amazon.com Services LLC Sold by: 7.19 Condition: New A Long Walk to Water: Based on a True Story, Park, Linda Sue Sold by: Amazon.com Services LLC Sold by: 5.29 Condition: New
Sample Simplified Invoice Output:
*********************************************************** Final Details for Order #114-4625135-4373821 ***********************************************************
Order Placed: May 21, 2020 Amazon.com order number: 114-4625135-4373821 Order Total: $34.85
Shipped on May 22, 2020
Items Ordered/Price The Pirate's Coin: A Sixty-Eight Rooms Adventure (The Sixty-Eight Rooms Adventures), Malone, Marianne Sold by: Amazon.com Services LLC Sold by: 7.99 Condition: New
Pax, Pennypacker, Sara Sold by: Amazon.com Services LLC Sold by: 5.89 Condition: New
The River (A Hatchet Adventure) Paulsen, Gary Sold by: Amazon.com Services LLC Sold by: 8.49 Condition: New
Brian's Return (A Hatchet Adventure) Sold by: Amazon.com Services LLC Sold by: 7.19 Condition: New
A Long Walk to Water: Based on a True Story, Park, Linda Sue Sold by: Amazon.com Services LLC Sold by: 5.29 Condition: New
Shipping Address
Michael Whitehead 1298 Hares Hill Road Kimberton, PA 19442 United States
Shipping Speed: OneDay Payment Method: Signature | **** 7744 Rewards Points
Item(s) Subtotal: $34.85 Shipping and Handling: $7.25
Total before tax: $42.10 Estimated tax to be collected: $2.53
Grand Total: $44.63
Billing Address
Michael Whitehead 1298 Hares Hill Road Kimberton, PA 19442 United States
Credit Card Transactions: Amazon.com Visa ending in **** 7744: May 22, 2020 : $44.63
Miscellaneous Formatting Tricks you will Need:
import java.text.NumberFormat; import java.text.ParseException; import java.text.SimpleDateFormat; import java.util.Date; public class FormattingAndEnumDemo { enum ShipmentStatus {InProcess, Shipped, Delivered} public static void main(String[] args) { double price = 99.99; String creditCardNumber = "132-444-2347-7744"; SimpleDateFormat dateFormat = new SimpleDateFormat("dd/MM/yyyy"); SimpleDateFormat dateFormat2 = new SimpleDateFormat("MMMM dd, yyyy "); NumberFormat formatter = NumberFormat.getCurrencyInstance(); try { Date date = dateFormat.parse("22/5/2020"); // Convert String to date System.out.println(dateFormat2.format(date)); // Convert Date to String System.out.println(formatter.format(price)); // Formatting Currency System.out.println(ShipmentStatus.Delivered); // How to print enum values System.out.println(creditCardNumber.substring(creditCardNumber.length() -4 )); // Last 4 character of a credit card number }catch (ParseException e) { System.out.println("Problem parsing date..."); } } } Output: May 22, 2020 $99.99 Delivered 7744
Sample Main Method Test Driver:
Your job is to implement the methods called here:
public static void main(String[] args) { AmazonOrderDetails od = new AmazonOrderDetails(); // Name of main class driver ArrayListproducts = new ArrayList<>(); od.addProducts(products); // Create your product data here Order order = od.createOrder(products); // Create your order od.createShipment(order); // Ship your order od.createPayment(order); // Create a payment record od.printOrderDetails(order); // Print od.printInvoice(order); }
Summary:
Your prototype should load your object model with sufficient data to print out the order with product-order line items, and an invoice with customer and shipping information. As mentioned above, use constructors where possible to set your attribute values.
Remember, your new career at Amazon depends on a good job on this assignment!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
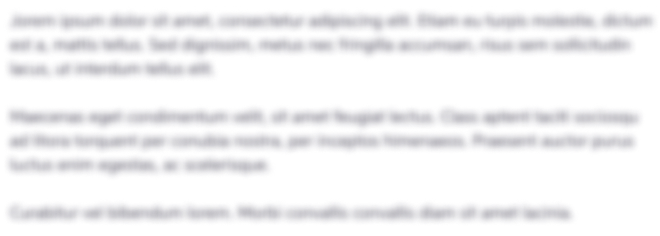
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started