Question
Project 3: BitVector & Application Implementing BitVector of undetermined size implementing new concepts of copy constructor and assignment operators as well as destructors to determine
Project 3: BitVector & Application
Implementing BitVector of undetermined size implementing new concepts of copy constructor and assignment operators as well as destructors to determine the prime numbers up to a maximum range.
Educational Objectives. After successfully completing this assignment, the student should be able to accomplish the following:
Implement a class from a class definition
Implement class BitVector
You may use the bitset and iomanip library
Implement global operators for a class
Implement global operators class BitVector
Correctly separate class definition and implementation using files
Create executables of class client programs using makefiles and the Make utility
Test a class using specs and an existing test platform
Create client applications to determine the Prime Numbers up to a maximum range
Operational Objectives: Implement the class BitVector
Deliverables: bitvector.cpp, bitvector.h, makefile
bitvector.h: I have included a draft or start of the bitvector.h which you may use and/or modify. Submit your own copy. Your program must work with the supplied main routine
#ifndef BITVECTOR_H #define BITVECTOR_H #include
Sample Main Routine to test if all of your member functions are defined:
#include } Functional Requirements: Implement the bitvector.h as stated in this document exactly. You may add the Test the functionality of all of the methods even if they are not used in determining if a vector was set or not. Write your own driver and be sure to test all of your member functions to see if the work properly. For instance, the Flip() should reverse all the bits. I would print out a before and after. Your constructors should turn all bits initially to off. Test the Flip() method that reverses all the bits by performing the Flip() and print out the bitvector to ensure all were flipped. I would print out a before and after . The default constructor should allocate enough words to store 256 bits. void Set (const size_t index); - Passes in a constant integer zero or larger and turns that particular bit on. If the bit value is out of range then take no action. void Set (); - Sets all bits to ones. You can do this by setting each word to a -1. void Unset (const size_t index); - Unsets all bits to zeros. void Flip (const size_t index); - Passes in a constant integer zero or larger and flips that particular bit. If the bit value is out of range then take no action. void Flip (); - Reverse all of the bits. bool Test (size_t index) const; - Check the bit passed in as a positive integer value. Return a True if the bit is set to one, otherwise return zero. If the value is out of range then return zero. void Print (string S); - Print the status of each bit. You may elect to print only the bits turned on or whether a bit is turned on or off. Passes a string to print as a header. explicit BitVector (size_t); - Creates a new BitVector object of size passed in. BitVector (const BitVector&); - Creates a new BitVector object and copies the contents of the the BitVector object passed in as a parameter. ~BitVector (); - Destroy and deallocate the memory for the BitVector Object. BitVector& operator = (const BitVector& a); - Copy (over write) the contents of one BitVector into another. They must be of the same size. size_t Size () const; - Return the size in the number of bits that the object will hold. void Unsetmultiple (const size_t index); - A value is passed to this function and the function unsets all bit locations that are multiples of that value. This is part of the Sieve algorithm.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
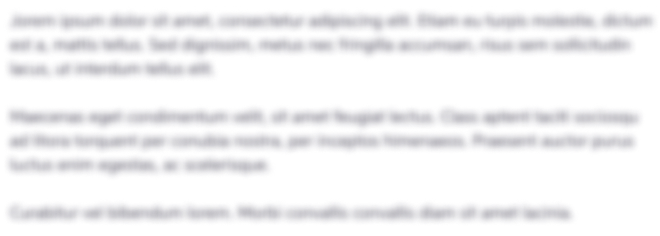
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started