Question
Project 3 Draft: ATM Introduction: Your task for this project is to create a very simple ATM. The project is broken into three sections: Collect
Project 3 Draft: ATM
Introduction:
Your task for this project is to create a very simple ATM. The project is broken into three sections:
Collect customer input
Calculate the ending balance
Display the results to the customer
Your final output should look like this:
Beginning Balance: 500.50 Deposit Amount: 200 Withdrawal Amount: 100
-----------------------------------------------
Collect Customer Input
The file is set up with a function to print the user options menu.
a. Create a prompt for the user to input the the account action. There are three actions:
Code | Action | Function |
---|---|---|
D | Deposit | deposit_amount |
W | Withdrawal | withdrawal_amount |
B | Account Balance | account_balance |
Create a function to print the account_balance, print the account actions and create the action for outputting the account balance outputting.
Input Information
The following data will be used as input in the test:
userchoice = input ("What would you like to do?") userchoice = 'B'
Output Information
Your current balance: 500.25
Collect Customer Input Check
Check It!
Request opening balance. Assign the opening balance to a variable called account_balance equal to $500.25. You will need to use the 'float' method in order to ensure that 'account_balance' is a float value.
Request the action to be taken on the account.
Create the action for Account Balance B, output to the console the account balance.
Test your code.
---------------------------------------------------------
Calculate the Balance - Deposit
If the action is Deposit 'D, use a deposit function to add funds to the account
You will need to write a function called deposit.
Request from the user the amount to be deposited. This value should be stored in a variable called deposit_amount. Use both the input and floatmethods in order to ensure the deposit_amount is a float value
Calculate a new account balance. Assign this calculation to a variable named balance.
The calculation for depositing funds into the account is balance = account_balance + deposit_amount
Print out the new account balance
Deposit Input
userchoice = input ("What would you like to do? ") userchoice = 'B' deposit_amount = 200
Deposit Output
What would you like to do? How much would you like to deposit today? Deposit was $200, current balance is $700.25
Customer Deposit
Check It!
------------------------------------------
Calculate the Balance - Withdrawal
If the action is Withdrawal W, use the withdrawal function to remove funds from the account
1. You will need to define a function called withdrawal.
2. Request from the user the amount to be withdrawn. This value should be stored in a variable called withdrawal_amount. Use both the input and floatmethods in order to ensure the withdrawal_amount is a float value
3. Ensure the withdrawal_amount is not greater than the account_balance.If the withdrawal_amount is greater than the `account_balance`, print the following message:
withdrawal_amount is greater that your account balance of account_balance
Ensure you display the withdrawal amount and the account balance with the '$' and two decimal points.
4. If the withdrawal amount is less than or equal to the account_balance then calculate a new account balance. Assign this calculation to a variable named balance
5.The calculation for withdrawing funds from the account is account_balance = account_balance -withdrawal_amount
6. Print print the following message:
Withdrawal amount was withdrawal_amount, your current balance is account_balance
Hint The formatter for a float value in Python is %f. With the formatter %.2f, you format the float to have 2 decimal places. For example:
print("Account balance: $%.2f" % account_balance)
Withdrawal Information
Withdraw Input
userchoice = input ("What would you like to do? ") userchoice = 'W' withdrawal_amount = 100
Withdraw Output
What would you like to do? How much would you like to withdraw? Withdrawal amount was $100, current balance is $600.25
Customer Withdrawal
Check It!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
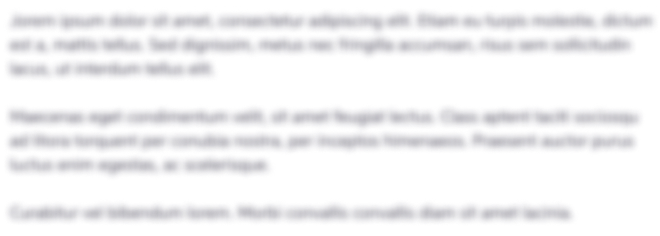
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started