Question
Project 3: Frozen Yogurt with Mix-ins Assignment For this project, you get to build off of the FrozenYogurt class from Project 2 and exercise using
Project 3: Frozen Yogurt with Mix-ins
Assignment
For this project, you get to build off of the FrozenYogurt class from Project 2 and exercise using loops in Java! A very common application of loops is input validation. You will get to do that in this project. Additionally, you get to use either a StringBuilder or StringBuffer object to store multiple toppings in a FrozenYogurt object.
FrozenYogurt class
For the FrozenYogurt class make the following changes to that class:
(Driver class will be separate)
1.Change the instance variable for the topping to be either a StringBuilder or StringBuffer object to appropriately support a comma-separated list of toppings (instead of just a single topping)
2.Change the setPrice method so that it returns a String. If the new price is negative, do not update the price and return a String with the following value: ALERT: Unable to set
3.Change setYogurtFlavor method so that it returns a String. If the new flavor is not "chocolate" nor "vanilla", do not update the base flavor and return a String with the following value: ALERT:
4.Add an addTopping method that take a String as a parameter. If the instance variable already has a topping, then separate the previous value and the new value with ", ". Otherwise, just use the new topping as the value (without a ","). For each additional topping added, add $0.49 to the price.
import java.util.Scanner;
public class FrozenYogurt{
//private instance variables
private String name;
private double price;
private String flavor;
private String topping;
//constructor with no parameters
public FrozenYogurt(){
this.name = "";
this.price = 0.0;
this.flavor = "";
this.topping = "";
}
// constructor with parameters
public FrozenYogurt(String name, double price, String flavor, String topping){
this.name = name;
this.price = price;
this.flavor = flavor;
this.topping = topping;
}
// mutator methods
public void setName(String name){
this.name = name;
}
public void setPrice(double price){
this.price = price;
}
public void setYogurtFlavor(String flavor){
this.flavor = flavor;
}
public void setTopping(String topping){
this.topping = topping;
}
//Accessor methods
public String getName(){
return name;
}
public double getPrice(){
return price;
}
public String getYogurtFlavor(){
return flavor;
}
public String getTopping(){
return topping;
}
//toString method
public String toString(){
return "Frozen Yogurt: " + name + " Price: $" + price + " Yogurt: " + flavor + " Toppping: " + topping + " ";
}
//User inputs
public String valueEntered(String s){
return "You entered: " + s + " ";
}
}
//driver class
import java.util.Scanner;
public class FrozenYogurtDriver
{
public static void main(String[] args) {
//Scanner class
Scanner sc = new Scanner(System.in);
//object creation from FrozenYogurt
FrozenYogurt froyo1 = new FrozenYogurt();
FrozenYogurt froyo2 = new FrozenYogurt();
System.out.println("Welcome to the Frozen Yogurt Selector");
//User inputs for object
System.out.print("Please enter the name of the first frozen yogurt: ");
froyo1.setName(sc.nextLine());
System.out.println(froyo1.valueEntered(froyo1.getName()));
System.out.print("Please enter the name of the second frozen yogurt: ");
froyo2.setName(sc.nextLine());
System.out.println(froyo2.valueEntered(froyo2.getName()));
//User inputs for prices
System.out.print("Please enter the price for the "+ froyo1.getName() + ": ");
froyo1.setPrice(Double.parseDouble(sc.nextLine()));
System.out.println(froyo1.valueEntered(String.valueOf(froyo1.getPrice())));
System.out.print("Please enter the price for the "+ froyo2.getName() + ": ");
froyo2.setPrice(Double.parseDouble(sc.nextLine()));
System.out.println(froyo2.valueEntered(String.valueOf(froyo2.getPrice())));
//User inputs for flavors
System.out.print("Please enter the base yogurt flavor for " + froyo1.getName() +": ");
froyo1.setYogurtFlavor(sc.nextLine());
System.out.println(froyo1.valueEntered(froyo1.getYogurtFlavor()));
System.out.print("Please enter the base yogurt flavor for " + froyo2.getName() +": ");
froyo2.setYogurtFlavor(sc.nextLine());
System.out.println(froyo2.valueEntered(froyo2.getYogurtFlavor()));
//User inputs for toppings
System.out.print("Please enter the topping to add to " + froyo1.getName() +": ");
froyo1.setTopping(sc.nextLine());
System.out.println(froyo1.valueEntered(froyo1.getTopping()));
System.out.print("Please enter topping to add to " + froyo2.getName() +": ");
froyo2.setTopping(sc.nextLine());
System.out.println(froyo2.valueEntered(froyo2.getTopping()));
//display toSting
System.out.println(froyo1);
System.out.println(froyo2);
//Final message
System.out.print("Thank you!");
}
}
Step by Step Solution
There are 3 Steps involved in it
Step: 1
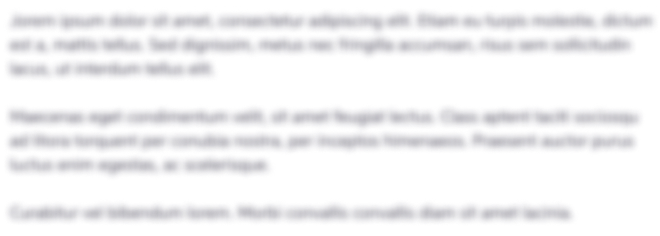
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started