Question
Project Description Complete the provided partial C++ program that will implement a Priority Queue ADT (abstract data type) in which the internal representation of the
Project Description
Complete the provided partial C++ program that will implement a Priority Queue ADT
(abstract data type) in which the internal representation of the priority queue is a doublelinked
series of dynamically allocated nodes.
The Priority Queue will be used to store text messages read from an input file. Each
message has a priority (H for High, M for Medium, L for Low). When input from the file by
the main() function, the message and priority information are stored within an object of
the type Message. The text message is stored as a string attribute, and the message
priority is represented by an attribute with type Priorities, an enumerated type provided.
*******************************************Main.cpp**********************************************
#include
#include
#include
#include
#include "priorityq.h"
using namespace std;
int main(int argc, char* argv[])
{
ifstream inputs; // Input file for commands
char op; // Hold operation
int n; // Integer input from file
PriorityQ* ptr = NULL; // Will point to priority queue object
Message msg; // Assembled message
Priorities p; // Message priority
char c; // Message priority input from file
string s; // Message string from file
// Output usage message if one input file name is not provided
if (argc != 2)
{
cout << "Usage: project03
return 1;
}
// Attempt to open input file -- terminate if file does not open
inputs.open(argv[1]);
if (!inputs)
{
cout << "Error - unable to open input file" << endl;
return 1;
}
// Process commands from input file
getline(inputs, s); // Input comment line
cout << s << endl; // Echo comment line
inputs >> op; // Attempt to input first command
while (inputs)
{
switch (op) // Identify and perform operation input from file
{
case '#': // Echo Comment
getline(inputs, s);
cout << "-------- " << op << s << endl;
break;
case 'c': // Constructor
cout << endl << "Constructor()";
try
{
ptr = new PriorityQ;
cout << endl;
}
catch ( std::bad_alloc )
{
cout << "Failed : Terminating now..." << endl;
return 1;
}
break;
case '+': // Enqueue
inputs >> c; // Input message priority from file
inputs.ignore(100, ' '); // Skip one space
getline(inputs,s); // Input rest of line as the message
cout << "Enqueue(" << c << " '" << s << "'" << ")";
try
{
switch (c)
{
case 'H': msg.SetPriority(HIGH); break;
case 'M': msg.SetPriority(MEDIUM); break;
case 'L': msg.SetPriority(LOW); break;
}
msg.SetMessage(s);
ptr->Enqueue(msg);
}
catch (FullPQ)
{
cout << " -- Failed Full PriorityQ";
}
cout << endl;
break;
case '-': // Dequeue
cout << "Dequeue() -- ";
try
{
ptr->Dequeue();
cout << "Successful";
}
catch (EmptyPQ)
{
cout << "Failed Empty PriorityQ";
}
cout << endl;
break;
case 'x': // Purge
inputs >> c; // Input message priority from file
cout << "Purge(" << c << ")";
try
{
switch (c)
{
case 'H': ptr->Purge(HIGH); break;
case 'M': ptr->Purge(MEDIUM); break;
case 'L': ptr->Purge(LOW); break;
}
}
catch (EmptyPQ)
{
cout << " -- Failed Empty PriorityQ";
}
cout << endl;
break;
case '?': // Peek
inputs >> n;
cout << "Peek(" << n << ") -- ";
try
{
ptr->Peek(n).Print();
}
catch (InvalidPeekPQ)
{
cout << "Failed Invalid Peek PriorityQ";
}
cout << endl;
break;
case 'f': // Front
cout << "Front() -- ";
try
{
ptr->Front().Print();
}
catch (EmptyPQ)
{
cout << "Failed Empty PriorityQ";
}
cout << endl;
break;
case 'r': // Rear
cout << "Rear() -- ";
try
{
ptr->Rear().Print();
}
catch (EmptyPQ)
{
cout << "Failed Empty PriorityQ";
}
cout << endl;
break;
case 'p': // Print PriorityQ
cout << "Print() -- ";
ptr->Print();
cout << endl;
break;
case 's': // Size of PriorityQ
cout << "Size() -- " << ptr->Size() << endl;
break;
case 'm': // Make PriorityQ Empty but ready for use
cout << "MakeEmpty()" << endl;
ptr->MakeEmpty();
break;
case 'd': // Destructor
delete ptr;
ptr = NULL;
cout << "Destructor()" << endl << endl;
break;
default: // Error
cout << "Error - unrecognized operation '" << op << "'" << endl;
cout << "Terminating now..." << endl;
return 1;
break;
}
inputs >> op; // Attempt to input next command
}
return 0;
} // End main()
*********************************************priorityq.h******************************************
#ifndef PRIORITYQ_H
#define PRIORITYQ_H
#include
#include "message.h"
using namespace std;
//
// Exception classes for PriorityQ
//
class EmptyPQ { /* No additional code */ }; // Exception class for empty PriorityQ condition
class FullPQ { /* No additional code */ }; // Exception class for full PriorityQ condition
class InvalidPeekPQ { /* No additional code */ }; // Exception class for invalid PriorityQ peek condition
//
// Priority Queue Node Structure
//
struct Node // Linked priority queue node structure
{
Message data; // Field for storing data in the priority queue node
Node* nextPtr; // Points to next priority queue node
Node* previousPtr; // Points to previous priority queue node
};
//
// PriorityQ class declaration
//
class PriorityQ // Double linked queue of messages sorted by priority
{
private:
Node* frontPtr; // Points to front node of priority queue
Node* rearPtr; // Points to rear node of priority queue
int count; // Number of values stored in priority queue
public:
/********** Start of functions you must implement for PriorityQ **************/
// Implement the following nine public functions in the file named priorityq.cpp
PriorityQ();
// PriorityQ()
// Initializes all private variables to indicate an empty priority queue
~PriorityQ();
//~PriorityQ()
// Deallocates all priority queue nodes
// No memory leak allowed
void MakeEmpty();
// MakeEmpty()
// Deallocates all priority queue nodes and returns priority queue to empty ready-to-use state
// No memory leak allowed
void Enqueue(Message msg);
// Enqueue()
// Adds value to priority queue in correct position and increments count.
// Duplicates are allowed.
// Highest priority messages must always be at front of queue
// Lowest priority messages must always be at rear of queue
// Add AFTER messages of similar priority
// If queue is already full, throws FullPQ exception.
void Dequeue();
// Dequeue()
// Removes highest priority message from front of priority queue and decrements count.
// If queue is empty, throws EmptyPQ exception
// No memory leak allowed
void Purge(Priorities p);
// Purge()
// Removes all messages of priority p from queue leaving all other messages in priority queue
// If queue is empty, throws EmptyPQ exception
// No memory leak allowed
Message Front() const;
// Front()
// Returns message at front of priority queue
// If queue is empty, throws EmptyPQ
Message Rear() const;
// Rear()
// Returns message at rear of priority queue
// If queue is empty, throws EmptyPQ
Message Peek(int n) const;
// Peek()
// Returns message n positions from front of priority queue
// If position n does not exist, throws InvalidPeekPQ
bool IsFull() const;
// IsFull()
// Returns true if queue is full. Returns false otherwise. DOES NOT MODIFY THE PRIORITY QUEUE
bool IsEmpty() const;
// IsEmpty()
// Returns true if queue is empty. Returns false otherwise. DOES NOT MODIFY THE PRIORITY QUEUE
int Size() const;
// Size()
// Returns number of items stored in priority queue. DOES NOT MODIFY THE PRIORITY QUEUE
/*********** End of functions you must implement for PriorityQ ***************/
void Print() const
// Print() -- DO NOT MODIFY OR RELOCATE THIS FUNCTION
// Prints contents of priority queue without modifying its contents
{
Node* tempPtr = frontPtr;
// Prints queue nodes Front-to-Rear order
cout << "Front { ";
while (tempPtr != NULL)
{
//cout << '(' << tempPtr->data.GetMessage() << ')' << ' ';
tempPtr->data.Print();
cout << ' ';
tempPtr = tempPtr->nextPtr;
}
cout << "} Rear Rear { ";
// Prints queue nodes Rear-to-Front order
tempPtr = rearPtr;
while (tempPtr != NULL)
{
//cout << '(' << tempPtr->data.GetMessage() << ')' << ' ';
tempPtr->data.Print();
cout << ' ';
tempPtr = tempPtr->previousPtr;
}
cout << "} Front";
} // End Print()
};
#endif
******************************************message.h*********************************************
#ifndef MESSAGE_H
#define MESSAGE_H
#include
using namespace std;
//
// Define enumerated Priorities type
//
enum Priorities {UNKNOWN, LOW, MEDIUM, HIGH};
//
// Message class declaration
//
class Message // Models a single message with priority
{
private:
Priorities priority; // Stores message priority
string message; // Stores message contents
public:
/********** Start of functions you must implement for Message **************/
// Implement the following five public functions in the file named message.cpp
Message(); // Initializes message to empty string with UNKNOWN priority
void SetPriority(Priorities p); // Sets priority equal to p
void SetMessage(string msg); // Sets message equal to msg
Priorities GetPriority() const; // Returns priority value without modification
string GetMessage() const; // Returns message contents without modification
/*********** End of functions you must implement for Message ***************/
void Print() const // DO NOT MODIFY OR RELOCATE THIS FUNCTION
{
cout << "[";
if (priority == HIGH)
cout << "H";
else if (priority == MEDIUM)
cout << "M";
else if (priority == LOW)
cout << "L";
else
cout << "U";
cout << ", " << message << "]";
} // End Print() const
};
#endif
Step by Step Solution
There are 3 Steps involved in it
Step: 1
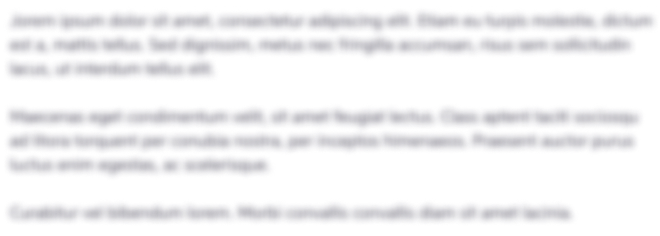
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started