Question
Project Goals: To write a C++ program that: Builds upon and reinforces our work in Project #1 Utilizes selection (if, if..else) and iteration (while, for,
Project Goals:
To write a C++ program that:
Builds upon and reinforces our work in Project #1
Utilizes selection (if, if..else) and iteration (while, for, do..while) constructs, as
appropriate
Illustrates multi-way branches
Prep Readings:
Problem Solving with C++, Chapters 2 and 3.
Project Requirements:
1. Develop a program that calculates the cost of a mobile device service. The cost of the
service is determined by the base price and number of message units to be used. The
packages are structured so that the basic package, package "A," is the least expensive if the
user doesn't use many message units, however if the user uses more than the pre-paid
minimum, the charge is significantly more for each additional message unit. Users who
choose package "C" pay a flat rate for unlimited use. The cost of Package "B" is between
these extremes. Details of the packages structure are listed here:
Package Base Price Additional Fees
A $19.99 $0.25 per message unit after 20 units
B $39.99 $0.10 per message unit after 100 units
C $59.99 Unlimited message units
2. This software is a "what if" price quote producer, so after getting the first quote, the user
can continue to ask for quotes and see results. After each quote, the system will prompt the
user to keep going (y or n), until the user enters the letter "n" (not case sensitive) after a
successful quote. You should assume that the software will run at least ONCE!
3. The program will require some validity checking:
The user is required to enter a character to represent the package chosen. If a
character other than ('A', 'a', 'B', 'b', 'C', 'c') is entered, the program provides a
message and re-prompts them for a new package selection. The program will
continue to prompt the user until a valid package is chosen. Assume the user will
only enter a single character; there is no need to error check for entries of multiple
characters.
The user is required to enter the number of message units used; it must be between
1 and 672 (the number of hours in 4 weeks) inclusive. If a value outside that range is
entered the program provides an error response and prompts the user to try again.
Assume the user will only enter integers, there is no need to error check for letters
or decimal value
2. Add the calculation to the program to show the user what it will cost depending
on which package is selected and the number of hours. No error checking, or
package comparison needed at this point.
3. Add code that will test which package is selected and compare cost of an
upgrade, then display a message informing the user how much the upgrade will
save them.
4. Add the error handling. (looping constructs)
5. Put the entire program in the loop that keeps getting new package/message unit
combinations.
Submission:
1. Compile and run your program one last time before submitting it. Your program must run in
either Dev C++ (recommended) or the version of JGrasp available through ArgoApps.
2. The name of your program file should be: lastname_firstname_project#.cpp, where # is the
number of the project. For example, if your name is Ricky Landry and you are submitting
project 1, your source code file should be named landry_ricky_project1.cpp
3. Login to UWF's eLearning system at http://elearning.uwf.edu/. Select our course.
4. Select the Assignment and click on the Submit Assignment button on the top.
5. Upload your file and check to make sure it finished.
6. Be sure to review the university policy on academic dishonesty. This is an individual project
and you should not work with anyone else in completing it. Do not hesitate to ask me questions
if you need help!
Important Notes:
Projects will be graded on whether students correctly solve the problem, and whether they
adhere to good programming practices including use of comments as specified in the materials,
using meaningful variable names, and proper indentations. Projects must be submitted by the
time specified as the due date. Projects submitted after that time will get a grade of zero.
Please review UWF's academic conduct policy. Note that viewing another student's solution,
whether in whole or in part, is considered academic misconduct. Also note that submitting code
obtained through the Internet or other sources, whether in whole or in part, is considered
academic misconduct. All programs submitted will be reviewed for evidence of academic
misconduct, and all violations will be handled accordingly.
SEE SAMPLE RUN ON NEXT PAGE
Sample Run:
Which package are you shopping for? (enter A, B, C)? A
How many message units(1 - 672)? 5
The charges are $9.95.
Do you want to go again (y/n)? y
Which package are you shopping for? (enter A, B, C)? a
How many message units(1 - 672)? 350
The charges are $92.45
By switching to Package B you would save $47.50
By switching to Package C you would save $52.50
Do you want to go again (y/n)? y
Which package are you shopping for? (enter A, B, C)? B
How many message units(1 - 672)? 350
The charges are $44.95
By switching to Package C you would save $5.00
Do you want to go again (y/n)? y
Which package are you shopping for? (enter A, B, C)? f
Enter only A, B, or C.
Which package are you shopping for? (enter A, B, C)? 5
Enter only A, B, or C.
Which package are you shopping for? (enter A, B, C)? a
How many message units(1 - 672)? -12
Please enter a number between 1 and 672 inclusive
How many message units(1 - 672)? 7
The charges are $9.95.
Do you want to go again (y/n)? y
Which package are you shopping for? (enter A, B, C)? a
How many message units(1 - 672)? 90000
Please enter a number between 1 and 672 inclusive
How many message units (1 - 672)? 7
The charges are $9.95
Do you want to go again (y/n)? N
Thank you for using this program. Goodbye.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
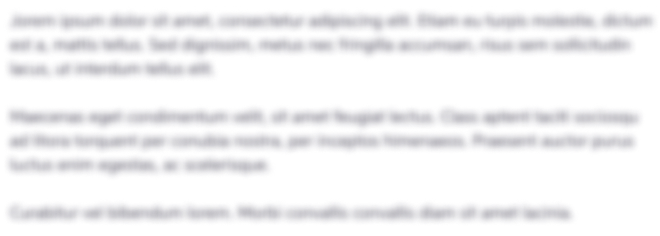
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started