Answered step by step
Verified Expert Solution
Question
1 Approved Answer
Project: Particle Filter - Based Robot Localization November 1 2 , 2 0 2 3 Abstract Based on the information from the Particle Filter slides,
Project: Particle FilterBased Robot Localization
November
Abstract
Based on the information from the Particle Filter slides, here is a project idea for students
involving the implementation of a Particle Filter for localization and navigation using Python. The
project is designed to be straightforward enough for students with some programming experience,
yet challenging enough to provide a comprehensive understanding of Particle Filters in a practical
scenario.
Project Description
In this project, students will implement a Particle Filter to estimate the position of a robot moving in
a twodimensional space. The robots environment will be represented as a grid, where each cell can
be either an obstacle or free space. The robot will have access to a simple sensor that provides noisy
measurements of its distance to the nearest obstacle in its front, left, right, and back directions.
Objectives
Implement a Particle Filter: Students will develop a Particle Filter to estimate the robots
location based on sensor readings and a map of the environment.
Simulate Robot Movement: Create a simulation where the robot moves a certain number of
steps in the environment, making random turns and moves.
Sensor Data Simulation: Generate simulated sensor data based on the robots actual position
and the map.
Visualization: Implement realtime visualization of the particle cloud and the estimated position of the robot in comparison to its actual position.
Implementation Approaches
Basic Python Implementation: Use standard Python libraries numpymatplotlib for visualization Represent the map as a D array, the robots position as coordinates, and particles as
objects with position and weight attributes. Implement particle resampling, motion update, and
measurement update functions.
ObjectOriented Approach: Define classes for the Robot, Particle, and Map. Implement
methods for movement, sensing and updating in each class. Use inheritance to showcase different
types of particles or robots, if desired.
Advanced Visualization with Pygame: Utilize the pygame library for more interactive
and sophisticated visualization. Allow realtime interaction, eg manually controlling the robots
movement or altering the environment.
Example Template
Import Necessary Libraries
import numpy as np
import matplotlib pyplot as plt
from matplotlib animation import FuncAnimation
Define the Robot and Particle Classes
class Robot :
def init self x y orientation :
self x x
self y y
self orientation orientation # in degrees
def move self deltax deltay deltaorientation :
self x deltax
self y deltay
self orientation self orientation deltaorientation
# Simulate sensor reading based on robot s position
def sense self environmentmap :
# Implement sensor reading logic here
pass
class Particle :
def init self x y orientation weight :
self x x
self y y
self orientation orientation
self weight weight
def move self deltax deltay deltaorientation :
# Add noise to movement
self x deltax np random normal
self y deltay np random normal
self orientation self orientation deltaorientation np random
normal
# Update weight based on measurement
def updateweight self measurement robotmeasurement :
# Implement weight updating logic here
pass
Initialize Robot and Particles
robot Robot
particles Particle np random randint np random randint np random
randint for in range
Particle Filter Algorithm
def particlefilter particles robot environmentmap movecommand :
# Move the robot and particles
robot move movecommand
for particle in particles :
particle move movecommand
# Update particles weights based on sensor reading
robotmeasurement robot sense environmentmap
for particle in particles :
particlemeasurement particle sense environmentmap # Particle s sense
method not shown
particle updateweight particlemeasurement robotmeasurement
# Resampling
weights np array particle weight for particle in particles
weights np sum weights # Normalize weights
indices np random choice range len particles size len particles p weights
resampledparticles particles i for i in indices
return resampledparticles
Visualization using Matplotlib
def update framenumber :
global particles robot
movecommand # Example move command
particles particlefilter particles robot environment
Step by Step Solution
There are 3 Steps involved in it
Step: 1
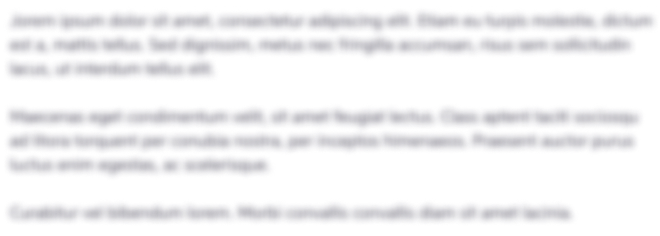
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started