Question
Project : Tower of Hanoi ---------------------------- In this assignment, you will implement tower of hanoi by using learned static stack and algorithms. ## Getting started
Project : Tower of Hanoi ----------------------------
In this assignment, you will implement tower of hanoi by using learned static stack and algorithms.
## Getting started
Investigate the contents of files. You should have the following files:
- `CMakeLists.txt` --- The build system for your homework. Read this file carefully. - `mystack.h` --- The definition of your static class template and its associated functions will go here. You need to finish all functions that required for the project. - `towers.cpp` and `towers.h` --- The definition of your tower of hanoi class and its associated functions will go here. You need to finish all functions that required for the project. - `towerofhanoi.cpp` --- main() function goes into this file. It starts the tower and run the tower. You don't need to modify this file. - `results.txt` --- The result after your program runs. Your output must match this file
Make a directory called towerofhanoi, and move all above files into the directory. You can try building the project, but it won't work.
## project requirements
1. Some instruction details are provided in files, you must read these instructions before writing your code. 2. You must finish all functions that classes require. 3. Your results must match to the results provided ## end of project requirements
## if you can give me your email id i can send you the txt, cpp, h files. thank you
THE FILES FOR THIS ASSIGNMENT:
##### mystack.h
#ifndef MYSTACK_H
#define MYSTACK_H
//***************************************//
//static stack template //
//finish all functions //
//***************************************//
#include
using namespace std;
template
class MyStack
{
private:
T *stackArray; // Pointer to the stack array
int stackSize; // The stack size
int numElem; //index of the top element in the stack array
public:
// Constructor
MyStack(int); //pass in an int: tells the maximum size of the stack
// Copy constructor
MyStack(const MyStack &);
//operator= overloading
MyStack& operator=(const MyStack &);
// Destructor
~MyStack();
// Stack operations
void push(T); //add an item to the stack by passing in a value
T pop(); //pop a value out by returning the value
T top(); //return the value at the top position in the stack
bool isFull() const; //tell if the statck is full
bool isEmpty() const; //tell if the stack is empty
int size() const; //tell how many items are in the stack
};
//YOUR CODE
//......
#endif
#### towerhanoi.cpp:
//do not modify this file
#include
#include "towers.h"
using namespace std;
int main()
{
int numDisk = 4;
Towers t(numDisk);
t.start();
return 0;
}
##### towers.cpp:
#include
#include "towers.h"
#include "mystack.h"
using namespace std;
//YOUR CODE
//......
//display disks on the three pegs in the console window (stdout)
//DO NOT MODIFY THIS FUNCTION
void Towers::plotPegs()
{
if (peg1==NULL || peg2==NULL || peg3==NULL) return;
int n1=peg1->size();
int n2=peg2->size();
int n3=peg3->size();
int numDisk = n1+n2+n3;
MyStack
MyStack
MyStack
//plot
for (int i=0; i //peg1 if (numDisk-n1-i>0) { for (int j=0; j cout << " "; } else { int m1 = tmp1.top(); tmp1.pop(); for (int j=0; j cout << "*"; for (int j=m1; j cout << " "; } cout <<" | "; //peg2 if (numDisk-n2-i>0) { for (int j=0; j cout << " "; } else { int m2 = tmp2.top(); tmp2.pop(); for (int j=0; j cout << "*"; for (int j=m2; j cout << " "; } cout <<" | "; //peg3 if (numDisk-n3-i>0) { for (int j=0; j cout << " "; } else { int m3 = tmp3.top(); tmp3.pop(); for (int j=0; j cout << "*"; for (int j=m3; j cout << " "; } cout< } cout << "_________________________________________ "; } ##### towers.h : #ifndef TOWERS_H #define TOWERS_H #include "mystack.h" //********************************// //prototype of class tower // //put all functions to towers.cpp // //DO NOT MODIFY THIS FILE // //********************************// class Towers { private: MyStack MyStack MyStack int numDisk; //number of discs to move public: //constructor, passing in an int to tell how many discs to move Towers(int); //destructor ~Towers(); //copy constructor Towers(const Towers &); //operator= overloading Towers& operator=(const Towers &); //start function, DO NOT MODIFY void start() { move(numDisk, peg1, peg2, peg3); } private: void setDisks(); //initialize start-tower by putting discs in, then print out the status of three towers after initialized void plotPegs(); //print out the status of three towers void move(int n, MyStack void moveOne(MyStack }; #endif results.txt: * | | ** | | *** | | **** | | _________________________________________ | | ** | | *** | | **** | * | _________________________________________ | | | | *** | | **** | * | ** _________________________________________ | | | | *** | | * **** | | ** _________________________________________ | | | | | | * **** | *** | ** _________________________________________ | | | | * | | **** | *** | ** _________________________________________ | | | | * | ** | **** | *** | _________________________________________ | | | * | | ** | **** | *** | _________________________________________ | | | * | | ** | | *** | **** _________________________________________ | | | | | ** | * | *** | **** _________________________________________ | | | | | | * ** | *** | **** _________________________________________ | | | | * | | ** | *** | **** _________________________________________ | | | | * | | *** ** | | **** _________________________________________ | | | | | | *** ** | * | **** _________________________________________ | | | | ** | | *** | * | **** _________________________________________ | | * | | ** | | *** | | **** _________________________________________ CMakeLists.txt: # Requires the `cmake` command to be at least version 2.8. cmake_minimum_required(VERSION 2.8) # Declares the project name and its implementation language. "CXX" # means "C++". project(towerofhanoi CXX) # Turn on the generation of test suites. When this command is present # in the CMakeListst.txt file, it will create a `test` target that # you can run as: # # make test # # from within the build directory. # enable_testing() # Update the C++ compiler flags so that we use C++11, not the older # version of the language, C++03. GCC-4.9, the default compiler in # our labs works with this setting. So does the default compiler on # an up-to-date Mac OS X system. set(CMAKE_CXX_FLAGS -std=c++11) # Declare that we want to build an executable program named "rc", which # stands for rational calculator). add_executable(twhn towerofhanoi.cpp towers.cpp) # Declare an executable test program and adds it to the test # suite. This program will be executed when you run # # make test # #add_executable(test_rational test_rational.cpp rational.cpp test.cpp) #add_test(test_rational test_rational) # DO NOT REMOVE OR MODIFY THIS MACRO. # # This creates a new target for the make program. After # including this, you can write: # # make printout # # And the build system will assemble a PDF containing the # formatted text in the files listed above. The PDF will # be created in the build directory and is named "printout.pdf". # # To use this, add the following to this file: # # add_printout(f1.hpp f1.cpp f2.hpp f2.cpp ...) # # Where f1 and f2 are the files that you want to include # in the printout. Note that header files should always # precede their source files. macro(add_printout) add_custom_target(printout WORKING_DIRECTORY ${CMAKE_SOURCE_DIR} COMMAND a2ps -Afill -o- ${ARGN} | ps2pdf - ${CMAKE_BINARY_DIR}/printout.pdf) endmacro() # Add files to the output. add_printout(towerofhanoi.cpp towers.cpp towers.h mystack.h)
Step by Step Solution
There are 3 Steps involved in it
Step: 1
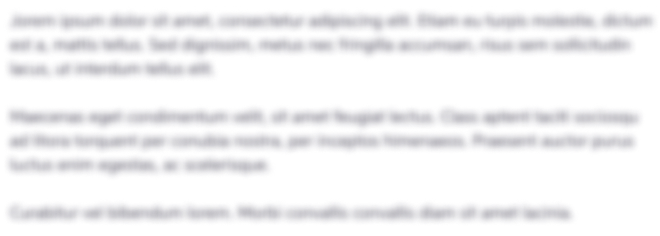
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started