Question
Project2 Consider an abstract class Money with two subclasses: class Coin that represents a coin class Bill that represents a paper bill. A paper bill
Project2
Consider an abstract class Money with two subclasses:
- class Coin that represents a coin
- class Bill that represents a paper bill.
A paper bill can have one of the 7 denomination values: 1, 2, 5, 10, 20, 50, and 100 dollars with names: WASHINGTON, JEFFERSON, LINCOLN, HAMILTON, JACKSON, GRANT, and FRANKLIN respectively.
A coin can have one of the 4 denomination values: 1, 5, 10, and 25 cents with names: PENNY, NICKEL, DIME, and QUARTER respectively.
Denominations and denomination names are defined in enum types: CoinDenomination and BillDenomination.
Each coin and bill can either be placed HEADS or TAILS up.
Coin and Bill constructors generate the coinDenomination and billDenomination randomly based on the number of denominations defined in the respective enums.
The method getValue returns the value of a coin (for example 0.25 for a quarter) or the value of paper bill respectively.
The method toString returns the String representation of the object; for example: WASHINGTON landed TAILS or NICKEL landed TAILS.
Money default constructor sets the value of heads to false.
The method toss simulates a money toss in which the coin/paper bill lands either heads up or tails up.
The method isHeads returns true if a coin is heads up.
The class has only one constructor.
Now, you need to have a piggy bank with predefined capacity to hold your monies. The piggy bank holds the monies but gives them no other organization. And it can certainly contain duplicates. The piggy bank is an ADT that has only the following operations:
- check the capacity of the piggy bank,
- you can add money to the piggy bank one at a time,
- adding fails if there is no more room in the piggy bank (PiggyBankFullException will be thrown that must be handled by the client)
- remove one (randomly selected),
- remove fails if the piggy bank is empty (PiggyBankEmptyException will be thrown that must be handled by the client)
- see whether the piggy bank is empty,
- see whether the piggy bank is full,
- see how many monies can fit in the piggy bank,
- see how many monies are in the piggy bank,
- see the content of the piggy bank,
- shake the piggy bank to rearrange the monies,
- empty the piggy bank counting how many monies landed HEADS
This functionality should be implemented in a class PiggyBank where the piggy bank is represented as a ResizableArrayBag of Money objects (this.jar instance variable).
The PiggyBank constructor:
- takes two inputs: numberOfMoneys to put initially to the new created piggy bank and capacity of the piggy bank
- creates this.jar object and fills it with the given number of monies, randomly (by calling nextBoolean) choosing a Coin or a Bill object to create and add to this.jar.
PLEASE NOTE:
- that shake method must utilize toArray method to get the access to the objects:
Object[] toShake = this.bucket.toArray();
For each element in the toShake array select randomly another element to swap it with. Once the objects are shuffled in the toShake array they need to be added to this.bucket (which needs to be cleared first)
- PiggyBank toString method utilizes polymorphism:
- Calls tArray t get the elements (see above)
- Uses a fr loop over the resulted array to compute the total - must use casting: ttal += ((Money) content[i]).getValue();
- Calls Arrays.tString t get the content of the objects
emptyPiggyBankAndCountHeads method while removing money from the piggy bank counts how many coins/bills landed HEADS, displays the count and the dollar value, and returns the heads count.
Client called CountHeadsGame is fully implemented. It manages a game where the user and the computer take turns to create the piggy bank with the same capacity and the same initial number of monies (since the monies are randomly generated the denominations will be different for each player). Each player empties the piggy bank. The player with the larger number of monies landing HEADS wins. The tie is also possible.
Round constructor creates PiggyBank object and displays the content of the piggy bank. The Round object is created in the main.
addCoinAndBill method creates one Coin object and one Bill object and attempts to add them to the piggy bank. Since the piggy bank may be full, the addCoinAndBill method must try/catch PiggyBankFullException
play method calls addCoinAndBill method; shakes the piggy bank; prints its content; calls emptyPiggyBankAndCountHeads method and returns the number of heads returned by the method.
See the Sample Runs below.
Your Task:
- Open the provide project in IDEA
- Create all the remaining classes as defined in the UML diagram provided below:
- Define all the methods as skeletons first (see Round class)
- Declare all instance variables private
- Pay attention which methods should be defined as abstract
- Make sure to implement inheritance relationship
- Pay attention which variables should be defined as static
- Pay attention which variables should be defined as final
- Make sure that all constants have appropriate values assigned at the declaration level
- Do not start implementation until all classes compile as skeletons
- Use javadoc-style comments to describe the purpose of each method, its parameters and return values.
- Implement the application iteratively using the bottom-up approach by implementing the classes in the following order:
- CoinDenomination
- BillDenomination
- Money
- Bill
- Coin
- PiggyBank
- Round
- Make sure that the output is properly formatted as shown in the Sample Runs below
Run#1:
How many coins/bills can fit in your piggy bank?
10
How many coins/bills should the computer put in your piggy bank?
60
How many rounds should we play?
4
How many coins/bills can fit in your piggy bank?
10
How many coins/bills should the computer put in your piggy bank?
6
How many rounds should we play?
4
******* Round #1 --> COMPUTER'S TURN *******
>> Adding 6 monies to your piggy bank <<
Added $100.00 to the piggy bank
Added $0.05 to the piggy bank
Added $0.10 to the piggy bank
Added $5.00 to the piggy bank
Added $0.05 to the piggy bank
Added $5.00 to the piggy bank
>> The content of your piggy bank <<
The piggy bank can hold 10 coins/bills;
There are 6 coins/bills in the piggy bank:[FRANKLIN landed TAILS, NICKEL landed TAILS, DIME landed TAILS, LINCOLN landed TAILS, NICKEL landed TAILS, LINCOLN landed TAILS]
The total of $110.20
--> Adding one more coin and one more bill:
Added $0.05 to the piggy bank
Added $20.00 to the piggy bank
>> Shaking your piggy bank <<
The piggy bank can hold 10 coins/bills;
There are 8 coins/bills in the piggy bank:[LINCOLN landed TAILS, NICKEL landed TAILS, DIME landed TAILS, LINCOLN landed TAILS, NICKEL landed TAILS, JACKSON landed TAILS, NICKEL landed TAILS, FRANKLIN landed TAILS]
The total of $130.25
>> Emptying your piggy bank <<
FRANKLIN landed TAILS
NICKEL landed HEADS
JACKSON landed HEADS
NICKEL landed HEADS
LINCOLN landed HEADS
DIME landed HEADS
NICKEL landed TAILS
LINCOLN landed HEADS
6 out of 8 coins/bills landed "HEADS"
The total value of "HEADS" is: $30.20
******* Round #2 --> USER'S TURN *******
>> Adding 6 monies to your piggy bank <<
Added $10.00 to the piggy bank
Added $50.00 to the piggy bank
Added $0.25 to the piggy bank
Added $1.00 to the piggy bank
Added $100.00 to the piggy bank
Added $0.01 to the piggy bank
>> The content of your piggy bank <<
The piggy bank can hold 10 coins/bills;
There are 6 coins/bills in the piggy bank:[HAMILTON landed TAILS, GRANT landed TAILS, QUARTER landed TAILS, WASHINGTON landed TAILS, FRANKLIN landed TAILS, PENNY landed TAILS]
The total of $161.26
--> Adding one more coin and one more bill:
Added $0.10 to the piggy bank
Added $50.00 to the piggy bank
>> Shaking your piggy bank <<
The piggy bank can hold 10 coins/bills;
There are 8 coins/bills in the piggy bank:[PENNY landed TAILS, WASHINGTON landed TAILS, FRANKLIN landed TAILS, DIME landed TAILS, QUARTER landed TAILS, GRANT landed TAILS, GRANT landed TAILS, HAMILTON landed TAILS]
The total of $211.36
>> Emptying your piggy bank <<
HAMILTON landed HEADS
GRANT landed TAILS
GRANT landed TAILS
QUARTER landed TAILS
DIME landed HEADS
FRANKLIN landed TAILS
WASHINGTON landed TAILS
PENNY landed TAILS
2 out of 8 coins/bills landed "HEADS"
The total value of "HEADS" is: $10.10
******* Round #3 --> COMPUTER'S TURN *******
>> Adding 6 monies to your piggy bank <<
Added $0.25 to the piggy bank
Added $20.00 to the piggy bank
Added $0.25 to the piggy bank
Added $2.00 to the piggy bank
Added $0.25 to the piggy bank
Added $10.00 to the piggy bank
>> The content of your piggy bank <<
The piggy bank can hold 10 coins/bills;
There are 6 coins/bills in the piggy bank:[QUARTER landed TAILS, JACKSON landed TAILS, QUARTER landed TAILS, JEFFERSON landed TAILS, QUARTER landed TAILS, HAMILTON landed TAILS]
The total of $32.75
--> Adding one more coin and one more bill:
Added $0.01 to the piggy bank
Added $20.00 to the piggy bank
>> Shaking your piggy bank <<
The piggy bank can hold 10 coins/bills;
There are 8 coins/bills in the piggy bank:[QUARTER landed TAILS, HAMILTON landed TAILS, QUARTER landed TAILS, JACKSON landed TAILS, JEFFERSON landed TAILS, PENNY landed TAILS, QUARTER landed TAILS, JACKSON landed TAILS]
The total of $52.76
>> Emptying your piggy bank <<
JACKSON landed TAILS
QUARTER landed HEADS
PENNY landed TAILS
JEFFERSON landed HEADS
JACKSON landed TAILS
QUARTER landed HEADS
HAMILTON landed TAILS
QUARTER landed TAILS
3 out of 8 coins/bills landed "HEADS"
The total value of "HEADS" is: $2.50
******* Round #4 --> USER'S TURN *******
>> Adding 6 monies to your piggy bank <<
Added $1.00 to the piggy bank
Added $5.00 to the piggy bank
Added $50.00 to the piggy bank
Added $0.25 to the piggy bank
Added $20.00 to the piggy bank
Added $0.25 to the piggy bank
>> The content of your piggy bank <<
The piggy bank can hold 10 coins/bills;
There are 6 coins/bills in the piggy bank:[WASHINGTON landed TAILS, LINCOLN landed TAILS, GRANT landed TAILS, QUARTER landed TAILS, JACKSON landed TAILS, QUARTER landed TAILS]
The total of $76.50
--> Adding one more coin and one more bill:
Added $0.10 to the piggy bank
Added $100.00 to the piggy bank
>> Shaking your piggy bank <<
The piggy bank can hold 10 coins/bills;
There are 8 coins/bills in the piggy bank:[QUARTER landed TAILS, QUARTER landed TAILS, GRANT landed TAILS, DIME landed TAILS, LINCOLN landed TAILS, JACKSON landed TAILS, FRANKLIN landed TAILS, WASHINGTON landed TAILS]
The total of $176.60
>> Emptying your piggy bank <<
WASHINGTON landed TAILS
FRANKLIN landed TAILS
JACKSON landed TAILS
LINCOLN landed TAILS
DIME landed TAILS
GRANT landed HEADS
QUARTER landed HEADS
QUARTER landed TAILS
2 out of 8 coins/bills landed "HEADS"
The total value of "HEADS" is: $50.25
***GAME OVER***
computerHeadsCount = 9
userHeadsCount = 4
Computer wins!!!
Process finished with exit code 0
Run#2:
How many coins/bills can fit in your piggy bank?
10
How many coins/bills should the computer put in your piggy bank?
9
How many rounds should we play?
2
******* Round #1 --> USER'S TURN *******
>> Adding 9 monies to your piggy bank <<
Added $50.00 to the piggy bank
Added $0.10 to the piggy bank
Added $0.05 to the piggy bank
Added $50.00 to the piggy bank
Added $0.10 to the piggy bank
Added $1.00 to the piggy bank
Added $0.25 to the piggy bank
Added $0.10 to the piggy bank
Added $2.00 to the piggy bank
>> The content of your piggy bank <<
The piggy bank can hold 10 coins/bills;
There are 9 coins/bills in the piggy bank:[GRANT landed TAILS, DIME landed TAILS, NICKEL landed TAILS, GRANT landed TAILS, DIME landed TAILS, WASHINGTON landed TAILS, QUARTER landed TAILS, DIME landed TAILS, JEFFERSON landed TAILS]
The total of $103.60
Step by Step Solution
There are 3 Steps involved in it
Step: 1
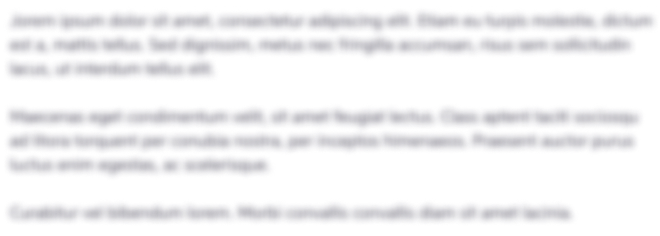
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started