Question
Provided is the source code for the SimpleTime class. It stores the time as three integers and does not override the equals method it inherits
Provided is the source code for the SimpleTime class. It stores the time as three integers and does not override the equals method it inherits from its superclass.
Subclass SimpleTime as GlobalTime by providing an integer zone field (named "zone") that allows for time zones values in the range of [-12, 12] (which doesn't comply with real-world conditions, but is ideal for this programming challenge).
Of course, make sure that every feature of the superclass remains functional in the subclass, along with any new features of the subclass that you are required to add (either by general Java conventions or the challenge requirements included here).
The toString of a GlobalTime should format as: H:MM:SS UTC[][Z]
That is: zero-pad the minute and second and leave no space between the literal "UTC" and the plus or minus sign and no space between the plus or minus sign and the zone. Note that if the zone is 0, then do not append either a plus or a minus sign nor the zone.
A GlobalTime object should be considered equal to a SimpleTime object if their hour, minute, and second match. Do not make any changes to the SimpleTime code. SimpleTime will not perform equals correctly but GlobalTime will.
/* * Source: SimpleTime.java * Author: Instructor * Detail: Superclass for use in the Advanced Java Design Lab */
public class SimpleTime { private int hour; // valid values 0 - 23 private int minute; // valid values 0 - 59 private int second; // valid values 0 - 59
public SimpleTime() { this(0, 0, 0); }
public SimpleTime(int h) { this(h, 0, 0); }
public SimpleTime(int h, int m) { this(h, m, 0); }
public SimpleTime(int h, int m, int s) { setSimpleTime(h, m, s); }
public SimpleTime(SimpleTime otherTime) { this(otherTime.hour, otherTime.minute, otherTime.second); }
public void setSimpleTime(int h, int m, int s) { setHour(h); setMinute(m); setSecond(s); }
public void setHour(int h) { hour = ( h >= 0 && h < 24 ) ? h : 0; }
public void setMinute(int m) { minute = ( m >= 0 && m < 60 ) ? m : 0; }
public void setSecond(int s) { second = ( s >= 0 && s < 60 ) ? s : 0; }
public int getHour() { return hour; }
public int getMinute() { return minute; }
public int getSecond() { return second; }
public String toString() { return String.format ( "%d:%02d:%02d %s", (hour == 0 || hour == 12) ? 12 : hour % 12, minute, second, hour < 12 ? "AM" : "PM" ); }
public static void main (String[] args) // SimpleTime Self-Test { SimpleTime t1 = new SimpleTime(20, 30, 40); SimpleTime t2 = new SimpleTime(20, 30, 40); SimpleTime t3 = new SimpleTime(20, 30, 50); System.out.println("Time 1 is: " + t1); System.out.println("Time 2 is: " + t2); System.out.println("Time 3 is: " + t3); System.out.println("Time 1 and 2 match: " + t1.equals(t2)); System.out.println("Time 2 and 3 match: " + t2.equals(t3)); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
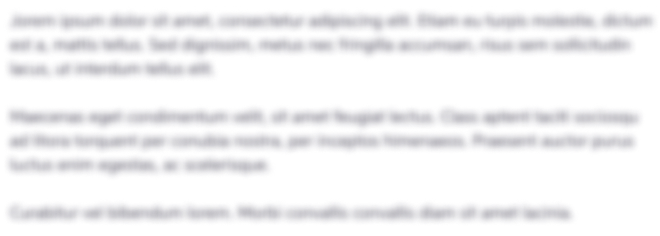
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started