Question
Pseudocode & Python In this portion of the project you will analyze a problem and create a Python program to solve it. In recent years,
Pseudocode & Python
In this portion of the project you will analyze a problem and create a Python program to solve it.
In recent years, there has been more attention to Water Quality. Specifically the amount of lead that is found in our daily water supply. Lead and copper residue start to show up in our water supply due to aging pipes. This residue can be found in older homes that have not replaced their pipes and in the municipal water supply. What this means is even if you have a new home or newer pipes, you can still have higher amounts of lead or copper in your water due to the age of the pipes that carry your water to your house.
According to the EPA, it is important to monitor your drinking supply on a regular basis. If lead concentrations exceed 15 ppb (parts per billion) and copper exceed 1.3 ppm (parts per million), corrective action may be necessary.
A group of environmental scientists have embarked upon a study of your areas water supply. They have divided the area into 4 sections: north, south, east and west. Within each section they have gathered water samples from 4 buildings. They have given you the data and are now asking you to create a program that will analyze the data.
Requirements
Create a Python program that will do each of the following:
List the names of each zone along with the measurement of lead and copper.
List the average lead and copper measurements.
List the average lead and copper measurements by zone.
List those buildings that have lead and copper values equal to or greater than the allowable values listed above.
List those buildings that have lead and copper values below the allowable values listed above.
They would like the program to offer a menu of options so that they can run whatever portion of the program they want at any given time. The menu should look like the following:
Select one of the options listed below:
P == Print Data
A == Get Averages
AZ == Average Per Zone
AL == Above Levels by Zone
BL == Below Levels
Q == Quit
Your program must use functions defined by you.
Some Help With the Logic
As with all programming projects it is important to plan out what your program needs to do. Begin by decomposing the problem keeping in mind that each part of the decomposition will represent a function/module. For each item listed in the decomposition, detail what that function/module of the code needs as far as data. Does the module need data? Does the data need to change while the module is working and does that change need to be reflected in the rest of the code? Does your module return data? And finally, what does each module do or try to accomplish.
Once you have completed your planning document, open up the file in week 13 of Canvas that reads: Water Quality Template.py. Begin by composing the loop that will allow the program to keep running in main(). Then start coding your functions. After you write each function, place your function call in main() and test your program. Expected output is listed towards the end of this guide sheet.
Program Requirement & Grading
Your program NEEDS to follow the rules listed below:
All code must reside in functions. Each item listed in the menu has to have its own function. Code outside of a function or main() will not be considered for grading.
Do not use GLOBAL or attempt to declare global variables.
Do not alter or change the lists in any way. As you need to move them throughout your program, how they appear in main() is how they need to be used. Do not attempt to splice the lists into segments.
Declare and use local variables and practice good naming conventions.
Do not declare any more variables in main(). What you see in the template is all you will need.
Contain your print statements to the individual functions.
Include comments in your code.
Make sure you consult with the rubric prior to turning in your assignment to make sure you have not missed anything.
Expected Output
What follows below is a series of outputs when the user selects the various items in the menu. Try to get your output to look as similar as possible to what is listed below.
When entering P:
Lead and Copper Water Study
Zone Lead Copper
****************************************
North 1 10 0.03
North 2 13 0.25
North 3 5 1.4
North 4 16 0.15
East 1 17 0.37
East 2 8 0.85
East 3 3 1.5
East 4 2 0.99
West 1 6 0.55
West 2 9 1.1
West 3 11 0.97
West 4 8 0.77
South 1 4 0.82
South 2 7 0.74
South 3 6 0.96
South 4 5 1.1
When entering A:
Average Levels of Lead and Copper
*****************************************
Lead Average: 8.12
Copper Average: 0.78
When entering AZ:
Average Values by Zone
********************************
LEAD Averages by Zone
********************************
North 11.00
East 7.50
West 8.50
South 5.50
COPPER Averages by Zone
********************************
North 0.46
East 0.93
West 0.85
South 0.91
When entering AL:
Zones Above Safe Levels
**********************************************
Lead Zones Above Acceptable Levels
**********************************************
North 4 16
East 1 17
Copper Zones Above Acceptable Levels
**********************************************
North 3 1.4
East 3 1.5
When entering BL:
Zones Below Safe Levels
**********************************************
Lead Zones Below Acceptable Levels
**********************************************
North 1 10
North 2 13
North 3 5
East 2 8
East 3 3
East 4 2
West 1 6
West 2 9
West 3 11
West 4 8
South 1 4
South 2 7
South 3 6
South 4 5
Copper Zones Below Acceptable Levels
**********************************************
North 1 0.03
North 2 0.25
North 4 0.15
East 1 0.37
East 2 0.85
East 4 0.99
West 1 0.55
West 2 1.1
West 3 0.97
West 4 0.77
South 1 0.82
South 2 0.74
South 3 0.96
South 4 1.1
Step by Step Solution
There are 3 Steps involved in it
Step: 1
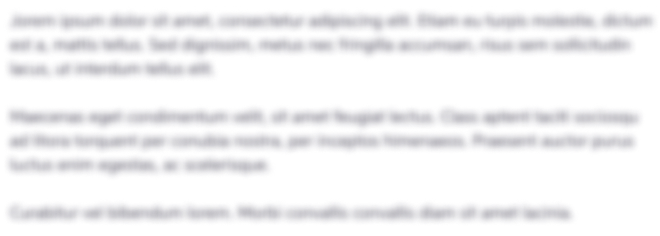
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started