Question
public abstract class Card implements Comparable { /* handy arrays for ranks and suits */ public final static String[] RANKS = { None, Joker, 2,
public abstract class Card implements Comparable
/* handy arrays for ranks and suits */ public final static String[] RANKS = { "None", "Joker", "2", "3", "4", "5", "6", "7", "8", "9", "10", "Jack", "Queen", "King", "Ace"}; public final static String[] SUITS = { "Diamonds", "Clubs", "Hearts", "Spades", "NONE"}; protected String suit; protected String rank;
// creates a card with specified suit and rank
public Card(String suit, int rank){ this.suit = suit; this.rank = RANKS[rank]; }
public Card(String suit, String rank){ // add code here if needed this.suit = suit; this.rank = rank; }
public abstract int getRank();
// the string representation of the rank of the current card
public abstract String getRankString();
// the suit of the current card
public abstract String getSuit();
@Override public final String toString(){ // outputs a string representation of a card object int r = getRank(); if( r >= 2 && r
}
import java.util.ArrayList; import java.util.List;
public class Deck{ protected List
public Deck(int num_jokers){ deck = new ArrayList // testing for (int i=0; i } public List // testing for (int i=0; i public Card getCard(){ Card removedCard=deck.get(0); deck.remove(0 ); System.out.println(removedCard.getRank() + removedCard.getSuit()); for (int i=0; i public void addCard(Card c){ deck.add((StandardCard) c); for (int i=0; i } import java.util.List; public class Hand{ protected List public Hand(List public int numberOfCards(){ if( this.cards == null ){ return -1; }else{ return this.cards.size(); } } public List public Card remove(Card card){ Card cardtoReturn = null; for (int i=0;i /* add the specified card to the hand */ public void add(Card card){ this.cards.add(card); } } public abstract class Player{ protected Hand hand; public Player(Hand hand){ this.hand = hand; } public abstract Card play(Card top_of_discard_pile, Deck deck); public final int cardsLeft(){ return this.hand.numberOfCards(); } } public class StandardCard extends Card implements Comparable public StandardCard(int rank, String suit) { super(suit,rank); if (rank != 1) { for (int i = 0; i public int getSuitInt(String suit) { int suitInt = 0; for (int i = 0; i @Override public String getRankString() { return this.rank; } @Override public String getSuit() { return this.suit; } @Override public int compareTo(Card o) { // int suitofFirstCard = 0; // int suitofSecondCard = 0; int rankofFirstCard = this.getRank(); int rankofSecondCard = o.getRank(); int value = 0; int suitofFirstCard = getSuitInt(this.getSuit()); int suitofSecondCard = getSuitInt(o.getSuit()); // for (int i = 0; i if (suitofFirstCard > suitofSecondCard){ value = 1; } else if (suitofFirstCard rankofSecondCard){ value = 1; } else { value = -1; } } return value; } public static void main (String[] args) { // int counter = 0; // for (int i = 1; i hand.add(c); System.out.println(hand.getCards()); hand.remove(c); System.out.println(hand.getCards()); } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
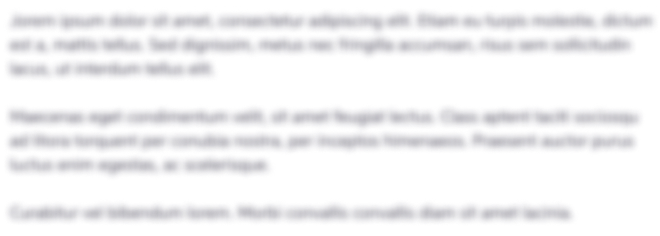
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started