Question
public class ArrayClass extends java.lang.Object Description: Class wrapper for a Java array, with additional management operations Note: Maintains a capacity value for maximum number of
public class ArrayClass
extends java.lang.Object
Description: Class wrapper for a Java array, with additional management operations
Note: Maintains a capacity value for maximum number of items that can be stored, and a size value for the number of valid or viable data items in the array
Implement the followings in ArrayClass:
-
Field Detail
-
DEFAULT_CAPACITY
private static final int DEFAULT_CAPACITY
See Also:
Constant Field Values
-
FAILED_ACCESS
public static final int FAILED_ACCESS
See Also:
Constant Field Values
-
localArray
private int[] localArray
-
arraySize
private int arraySize
-
arrayCapacity
private int arrayCapacity
-
-
Constructor Detail
-
ArrayClass
public ArrayClass()
Default constructor, initializes array to default capacity (10)
-
ArrayClass
public ArrayClass(int capacity)
Initializing constructor, initializes array to specified capacity
Parameters:
capacity - integer maximum capacity specification for the array
-
ArrayClass
public ArrayClass(int capacity, int size, int fillValue)
Initializing constructor, initializes array to specified capacity, size to specified value, then fills all elements with specified size value
Parameters:
capacity - maximum capacity specification for the array
size - sets the number of items to be filled in array, and sets the size of the ArrayClass object
fillValue - value to be placed in all elements of initialized array up to the size
-
ArrayClass
public ArrayClass(ArrayClass copied)
Copy constructor, initializes array to size and capacity of copied array, then copies only the elements up to the given size
Parameters:
copied - ArrayClass object to be copied
-
-
Method Detail
-
accessItemAt
public int accessItemAt(int accessIndex)
Accesses item in array at specified index if index within array size bounds
Parameters:
accessIndex - index of requested element value
Returns:
accessed value if successful, FAILED_ACCESS (-999999) if not
-
appendItem
public boolean appendItem(int newValue)
Appends item to end of array, if array is not full, e.g., no more values can be added
Parameters:
newValue - value to be appended to array
Returns:
Boolean success if appended, or failure if array was full
-
clear
public void clear()
Clears array of all valid values by setting array size to zero, values remain in array but are not accessible
-
getCurrentCapacity
public int getCurrentCapacity()
Description: Gets current capacity of arrayNote: capacity of array indicates number of values the array can hold
Returns:
capacity of array
-
getCurrentSize
public int getCurrentSize()
Description: Gets current size of arrayNote: size of array indicates number of valid or viable values in the array
Returns:
size of array
-
insertItemAt
public boolean insertItemAt(int insertIndex, int newValue)
Description: Inserts item to array at specified index if array is not full, e.g., no more values can be addedNote: Value is inserted at given index, all data from that index to the end of the array is shifted up by one
Note: Value can be inserted after the last valid element but not at any index past that point
Parameters:
insertIndex - index of element into which value is to be inserted
newValue - value to be inserted into array
Returns:
Boolean success if inserted, or failure if array was full
-
isFull
public boolean isFull()
Tests for size of array equal to capacity, no more values can be added
Returns:
Boolean result of test for full
-
isEmpty
public boolean isEmpty()
Tests for size of array equal to zero, no valid values stored in array
Returns:
Boolean result of test for empty
-
removeItemAt
public int removeItemAt(int removeIndex)
Description: Removes item from array at specified index if index within array size boundsNote: Each data item from the element immediately above the remove index to the end of the array is moved down by one element
Parameters:
removeIndex - index of element value to be removed
Returns:
removed value if successful, FAILED_ACCESS (-999999) if not
-
resize
public boolean resize(int newCapacity)
Description: Resets array capacity, copies current size and current size number of elementsException: Method will not resize capacity below current array capacity, returns false if this is attempted, true otherwise
Parameters:
newCapacity - new capacity to be set; must be larger than current capacity
Returns:
Boolean condition of resize success or failure
-
Step by Step Solution
There are 3 Steps involved in it
Step: 1
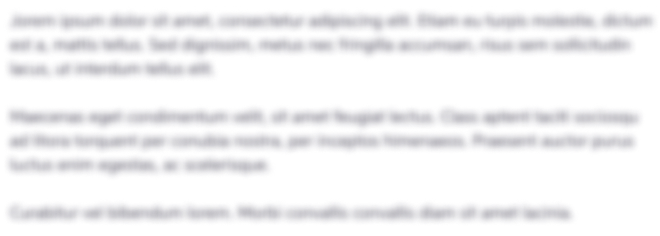
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started