Question
public class BankAccountMainProgram { public static void main(String[] arguments) { // create three bank accounts BankAccount accountNum1 = new BankAccount(); BankAccount accountNum2 = new BankAccount(1233203,
public class BankAccountMainProgram
{
public static void main(String[] arguments)
{
// create three bank accounts
BankAccount accountNum1 = new BankAccount();
BankAccount accountNum2 = new BankAccount(1233203, 105.51, "Betty");
BankAccount accountNum3 = new BankAccount(6542345, 33.11, "Veronica");
// Test the two String Method
System.out.println("*** Does the toStringMethod work?");
System.out.println("First account : " + accountNum1);
System.out.println("Second account : " + accountNum2);
System.out.println("Third account : " + accountNum3);
System.out.println();
// Do the get() method work?
System.out.println("*** Does the get() methods work?");
System.out.println(accountNum2.getName() + " has $" +
accountNum2.getBalance() +
" in account number " + accountNum2.getID() + ".");
System.out.println();
// Can we change the account name
System.out.println("*** Does the set() methods work?");
System.out.println("Second account Before : " + accountNum2);
accountNum2.setName("Archie");
System.out.println("Second account After : " + accountNum2);
// Can we change the account name
System.out.println();
System.out.println("*** Does the changeBalance() methods work?");
System.out.println("Third account Before : " + accountNum3);
accountNum3.changeBalance(10.10);
System.out.println("Third account After : " + accountNum3);
accountNum3.changeBalance(-50);
System.out.println("Third account After : " + accountNum3);
}
}
Create a java BankAccount class that works with the given client code BankAccountMainProgram. Each BankAccount object represents one users account information including name, customer ID and balance of money. Write a BankAccount class with the following data fields and methods:
Fields (All data fields should be declared as private
- ID (int)
- Balance (double)
- Name (string)
Methods
Default constructor
- sets the ID to 0
- sets the balance to 0
- sets the name to "unknown"
A full constructor
- Passes all three values as parameters and set the values accordingly
toString() method
- returns a string in the format as follows:
- Name [ID] = $balance
- Example: Betty [1233203] = $105.5
getName()
- returns the Name of this BankAccount
getBalance()
- returns the Balance of this BankAccount
getID()
- returns the ID of this BankAccount
setName(String newName)
- change the name on the account with newName
changeBalance(double x)
- add the amount x to the balance.
- if x is negative you still add it, but the balance should go down.
- If the balance is negative, then set it to zero before finishing.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
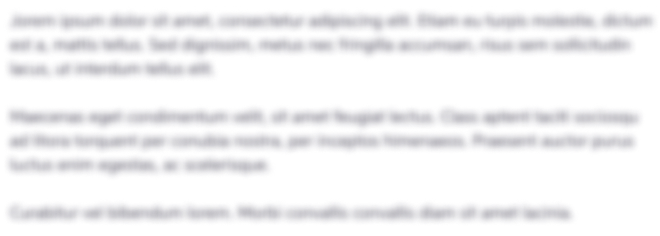
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started