Question
public class HospitalPatient { private static long admissionNumber; private String id; private String lastName; private String firstName; private SimpleDate admitDate; private char ward; private int
public class HospitalPatient { private static long admissionNumber; private String id; private String lastName; private String firstName; private SimpleDate admitDate; private char ward; private int roomNumber;
/* * Creates a new Hospital patient record. * @param admitDate The day the patient is officially admitted to hospital * @param lastname The patient's last name. * @param firstname The patient's first name. * @param ward Each hospital ward is designated as an uppercase letter. * @param roomNumber The 3-digit room number, first digit relates to the floor level. */ public HospitalPatient(SimpleDate admitDate, String lastName, String firstName, char ward, int roomNumber) { this.admitDate = admitDate; this.lastName = lastName; this.firstName = firstName; this.ward = ward; this.roomNumber = roomNumber; id = lastName+"_"+admissionNumber++; }
/** * @return A formatted string representing all the information about the patient. */ public String toString () { return getLocation()+"\tadmitted: "+admitDate+"\tid: "+id; }
/** * @return The unique id of the patient. */ public String getId() { return id; }
/** * @return The patient's last name. */ public String getLastName() { return lastName; }
/** * @return The patient's first name. */ public String getFirstName() { return firstName; } /** * @return a formatted string of the patient's full name and their current location in the hospital. */ public String getLocation() { return lastName+","+firstName+":\t"+ward+roomNumber; }
/** * Determines if two patients have identical id numbers, which in this case, means that they are the same. * @param other The patient to compare to this patient. * @return true if the id numbers are identical. */ public boolean equals(HospitalPatient other) { return id == other.id; }
/** * Determines the lexicographic order of the ids of two patients. * @param other The patient to compare to this patient. * @return if 0 then this patient is later in a sequence, * if = 0, then this patient and the other patient are equivalent. */ public int compareTo(HospitalPatient other) { return id.compareTo(other.id); }
/** * Updates the new location for a patient who has been moved into a new room. * @param ward The single letter representing the ward. * @param roomNumber The 3-digit room number. */ public void move(char ward, int roomNumber) { this.ward = ward; this.roomNumber = roomNumber; }
/** * Used for internal testing. * @param args Not used in this class. */ public static void main(String[] args) { HospitalPatient p1 = new HospitalPatient(new SimpleDate(2018,3,9),"Duck","Donald",'B',420); HospitalPatient p2 = new HospitalPatient(new SimpleDate(2018,3,7),"Mouse","Minnie",'D',333); System.out.println(p1); System.out.println(p2); System.out.println(p1.getId()); System.out.println(p1.getLastName()); System.out.println(p1.getFirstName()); System.out.println(p1.getLocation()); p1.move('F',104); System.out.println(p1); if (!p1.equals(p2)) { System.out.println("not the same patient"); } int cmp = p1.compareTo(p2); if (cmp 0){ System.out.println(p2.getId()+" comes before "+p1.getId()); } else { System.out.println("something is wrong"); } } }
public class SimpleDate { private int year = 2018; private int month = 1; private int day = 1;
private static final int[] daysInMonth = {31,29,31,30,31,30,31,31,30,31,30,31};
/** * Creates a SimpleDate. * @param year The year (from 1999 to the current year). * @param month The month (from 1 to 12). * @param day The day of the month. * @throws RuntimeException if any of the parameters are out of bounds. */ public SimpleDate(int year, int month, int day) { setYear(year); setMonth(month); setDay(day); }
/** * Creates a default date of January 1, 2018. */ public SimpleDate() { // keeps the defaults }
/** * Sets the year. * @param year The year (from 1999 to the current year). * @throws RuntimeException if the year is not valid. */ public void setYear(int year) { if (year 2018) { throw new RuntimeException("not a valid year"); } this.year = year; }
/** * Sets the month. * @param month The month (from 1 to 12). * @throws RuntimeException if the month is not valid. */ public void setMonth(int month) { if (month 12) { throw new RuntimeException("not a valid month"); } this.month = month; }
/** * Sets the day. * @param day The day of the month. * @throws RuntimeException if the day is not valid. */ public void setDay(int day) { if (day daysInMonth[month-1]) { throw new RuntimeException("not a valid day"); } }
/** * @return A formatted string of the day in yyyy/mm/dd format. */ public String toString() { return year+"/"+month+"/"+day; } }
class TreeNode { HospitalPatient item; TreeNode left; TreeNode right; // optional reference TreeNode parent;
/** * Creates a tree node. * @param item The element contained within the tree. * @param left The left child reference for this node. * @param right The right child reference for this node. * @param parent The reference for this node. */ TreeNode(HospitalPatient item, TreeNode left, TreeNode right, TreeNode parent) { this.item = item; this.left = left; this.right = right; this.parent = parent; }
/** * Creates a tree node that has no children. * @param item The element contained within the tree. * @param left The left child reference for this node. * @param right the right child reference for this node. */ TreeNode(HospitalPatient item, TreeNode left, TreeNode right) { this(item,left,right,null); }
/** * Creates a tree node. * @param item The element contained within the tree. */ TreeNode(HospitalPatient item) { this(item,null,null); }
/** * Creates a tree node with no elements and no children. */ TreeNode() {}
/* // for testing purposes only public String toString() { StringBuilder sb = new StringBuilder("TreeNode: item = "+item); if (left != null) { sb.append(" with left child"); } if (right != null) { sb.append(" with right child"); } if (parent != null) { sb.append(" with parent"); } return sb.toString(); } */ }
import java.awt.*; import java.util.*; import javax.swing.*;
public class ViewableTree extends JPanel{
private static final long serialVersionUID = 16L; /** * The approximate diameter, in pixels, of the initial node sizes. * Used when the tree has its own frame. */ public static final int NODE_START_SIZE = 30; /** * The approximate length, in pixels, of the initial edge sizes. * Used when the tree has its own frame. */ public static final int EDGE_START_SIZE = 20;
private AdmittedPatients tree;
private int size, height; private ViewableNode root; private LinkedList listing; //used for its iterator
/** * Does nothing really, except create an * empty JPanel with a preferred size. * Useful only in setting up an empty panel for another * GUI application. * @param canvasSize The preferred size of the initial canvas. */ public ViewableTree(Dimension canvasSize) { super(); this.setPreferredSize(canvasSize); repaint(); } /** * Generates a Drawn BinaryTree object in a Frame window. * The BinaryTree must have a protected data field called 'root' * @param tree The BinaryTree object that is to be drawn. * @param canvasSize The size of the canvas that will show the panel. */ public ViewableTree(AdmittedPatients tree, Dimension canvasSize) { super(); setTree(tree, canvasSize); repaint(); } /** * Generates a Drawn BinaryTree object in a Frame window. * The BinaryTree must have a protected data field called 'root'. * The panel size is determined by the number of nodes in the * tree and the height, which is calculated in this class. * @param tree The BinaryTree object that is to be drawn. */ public ViewableTree(AdmittedPatients tree) { super(); setTree(tree); int nodeWidth = power2(height-1); int canvasWidth = nodeWidth*(NODE_START_SIZE + EDGE_START_SIZE); int canvasHeight = height*(NODE_START_SIZE + EDGE_START_SIZE); this.setPreferredSize(new Dimension( canvasWidth, canvasHeight)); repaint(); } /** * This method is called by the super class whenever the window * is resized or moved. */ public void paintComponent(Graphics g) { super.paintComponent(g); drawTree(g); } /** * Creates a frame to house the ViewableTree panel * and renders it. */ public void showFrame() { JFrame frame = new JFrame("Binary Tree"); frame.setDefaultCloseOperation( JFrame.EXIT_ON_CLOSE ); frame.setContentPane(this); frame.pack(); frame.setVisible(true); } public void clearTree() { tree = null; repaint(); } public void setTree(AdmittedPatients tree, Dimension canvasSize) { setTree( tree ); this.setSize( canvasSize ); } public void setTree(AdmittedPatients tree) { this.tree = tree; TreeNode top = tree.root; if (top != null) { root = buildTree(top, 0); } else { throw new NullPointerException( "Cannot draw an empty tree"); } height = getHeight( root ); listing = new LinkedList(); inorderTraverse( root ); size = listing.size(); } /* * Private method * Calculates the height of the ViewableTree */ private int getHeight(ViewableNode subRoot) { if (subRoot == null) return 0; return 1 + Math.max( getHeight( subRoot.left ), getHeight( subRoot.right )); } /* * A private method that orders the nodes "in order". * into the LinkedList "listing". */ private void inorderTraverse(ViewableNode dbtn) { if (dbtn == null) { return; } inorderTraverse(dbtn.left); listing.addLast(dbtn); inorderTraverse(dbtn.right); } /* * A private recursive method that builds the * viewable BinaryTree. */ private ViewableNode buildTree(TreeNode top, int level) { if (top == null) return null; return new ViewableNode(top.item, buildTree(top.left, level+1), buildTree(top.right, level+1), level); } /* * A private method that actually draws the tree. */ public void drawTree(Graphics g) { g.setColor(Color.green); if (root == null) { g.drawString("NOTHING TO DRAW!!!", 20, 20); } else { Rectangle drawingArea = this.getBounds(); setPositions(drawingArea); Iterator it = listing.iterator(); while (it.hasNext()) { (it.next()).drawNode(g, true); } } } /* * A private method that determines the actual pixel * position of each of the nodes in the tree. * Must be called everytime the window changes or * moves. */ private void setPositions(Rectangle drawingArea) { int cornerX = drawingArea.x; int cornerY = drawingArea.y; int wGrid = drawingArea.width / size; int hGrid = drawingArea.height / (height); int nodeSize = Math.min( wGrid, hGrid ) / 2; int rX, rY;
Iterator it = listing.iterator(); ViewableNode current; rX = 0; while( it.hasNext() ) { current = it.next(); rY = current.yPos; current.setPosition( wGrid*rX+cornerX, hGrid*rY+cornerY, nodeSize ); rX++; } } /* * A very handy math/computer method to calculate power of 2 * results. */ private static int power2(int power) { if (power
/* * A private inner class. * The ViewableNode are the nodes specific to this * Viewable BinaryTree. * The Rectangle it extends is used as its bounding box. */ private class ViewableNode extends Rectangle { private static final long serialVersionUID = 5L; private ViewableNode left; private ViewableNode right; HospitalPatient element; Point midPoint; int yPos; /* * Creates the tree-position properties * of the Node. */ ViewableNode(HospitalPatient element, ViewableNode left, ViewableNode right, int yPos) { super(); this.element = element; this.left = left; this.right = right; this.yPos = yPos; } /* * Sets the actual Rectangular coordinates * of the super class (The Rectangle object). */ void setPosition(int xCoord, int yCoord, int size) { x = xCoord; y = yCoord; height = size; width = size; midPoint = new Point( (x+x+size)/2, (y+y+size)/2 ); } /* * Draws the node and the edges going to its children. */ void drawNode(Graphics g, boolean includeObj) { g.fillOval(x, y, width, height); if (left != null) { g.drawLine(midPoint.x, midPoint.y, left.midPoint.x, left.midPoint.y); } if (right != null) { g.drawLine(midPoint.x, midPoint.y, right.midPoint.x, right.midPoint.y); } if (includeObj) { int stringLength = element.toString().length(); int stringX = x; int stringY = y + width/3 + width/3; int fontsize; Color tmp = g.getColor(); g.setColor( Color.black ); if (stringLength > 3) { stringX = x; stringY = y + width/3 + width/3; fontsize = (width-stringLength)/3; } else { stringX = x+width/3; fontsize = width/3; } stringY = y + width/3 + width/3; if (fontsize > 0) { g.setFont( new Font( "TimesRoman", Font.BOLD, fontsize )); g.drawString( element.getId(), stringX, stringY ); } g.setColor( tmp ); } } }
Hospital Admission Information System Description. The local hospital Admissions Department needs to keep a record of current patients and room numbers. During the day, the Admission staff update, insert, delete and retrieve patient information from one of the workstations in the Admissions Department. Visitors also ask for information about the location of their loved ones. In the evening, when the Admissions Desk staff go home, two volunteers take over the job of locating patients for the evening and night visitors. However, the volunteers to do not have access to a workstation and therefore work from a printout of ordered patient names and room numbers. This list is printed daily for them by one of the Admission staff just before she goes home. We are asked to design a system for the Admissions Department. The hospital generally deals with thousands of admitted patient information and patient enquiries need to be handled quickly. We elect to use a BinarySearchTree data structure for relatively quick and easy access to the patient records, and because the inorder traversal easily processes records in their natural order, the Admissions Desk can produce an ordered printout for the volunteers at nightStep by Step Solution
There are 3 Steps involved in it
Step: 1
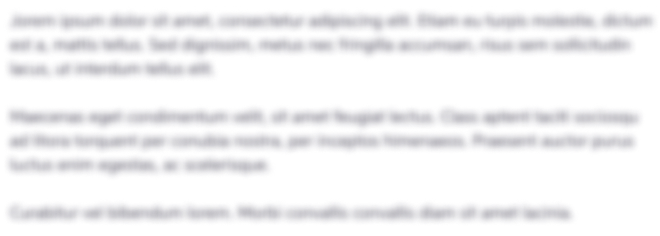
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started