Question
public class Inventory { private Item[] itemList = new Item[0]; private int goldCoins=0; double maximumCarryingCapacity = 0; int maximumNumberOfItems = 0; String name = null
public class Inventory {
private Item[] itemList = new Item[0];
private int goldCoins=0;
double maximumCarryingCapacity = 0;
int maximumNumberOfItems = 0;
String name = null;
/**
* Increase the array by one. You will need to create a new array one element
* bigger than the old array.
* No External Classes Permitted to Be Used in This Method
*
*/
private void increaseArray() {
}
/**
* shrink the array by one. You will need to create a new array one smaller
* than the current one then copy the old one into the new one.
* This method should fail if the list is less than 1 in size, also if the last
* element is not null.
* No External Classes Permitted to Be Used in This Method
* @return true if it was successful false if unsuccessful
*/
private boolean decreaseArray() {
return false;
}
/**
* Return the item at the specific location in the list
* If the item doesn't exist return null.
* This does not modify the item list. It will return
* No External Classes Permitted to Be Used in This Method
* @param index the position to return.
* @return The item at index or null if no item
*/
//ALL METHODS FROM HERE ON ARE REMOVED YOU NEED TO ADD THEM IN.
public Item itemAt(int index) {
}
/**
* Add gold to the inventory.
* @param amountToAdd add the gold to the inventory
* @return
*/
public void addGold (int amountToAdd){
goldCoins = goldCoins + amountToAdd;
}
/**
* remove gold from inventory.
* If you ask for more than you got in the inventory
* return -1 to flag that its not possible to remove gold
* else remove the gold and return the amount you removed
* from the inventory.
* @param amountToRemove The amount of gold to remove from the inventory
* @return the amount removed unless it would have gone negative then -1
*/
public int removeGold(int amountToRemove) {
if (getGold()>= amountToRemove)
this.goldCoins -= amountToRemove;
else
this.goldCoins = -1;
return this.goldCoins;
}
/**
* Returns the current gold coins in the inventory
* Not tested in Junit
* @return amount of gold in kitty
*/
public int getGold() {
return this.goldCoins;
}
/**
* Adds the provided item to the array. You will need to increase
* the array size and add the new item to the end of the list. If the
* name already exists (case does not matter) don't add the item.
* No External Classes Permitted to Be Used in This Method Apart From
* String.toUpperClass and String.Equals
* If the item status is broken do not add it to the list
* @param newItem the item to be added
* @return the index of the new item -1 if newItem was null, null or its broken
*/
public int addItem(Item newItem) {
}
/**
* remove the item at the index.
* Pack the array and shrink the array when finished.
* No External Classes Permitted to Be Used in This Method
* @param index of the item to remove
* @return the item removed. If no item at the index return null.
*
*/
public Item removeItem(int index) {
}
/**
* remove the item of name from the array.
* Pack the array and shrink the array when finished.
* case shouldn't matter.
* (Note that you should do this method by using other methods)
* No External Classes Permitted to Be Used in This Method
* @param nameOfItem The name of the item to be remove
* @return the item removed. If no item at the index return null.
*/
public Item removeItem(java.lang.String nameOfItem) {
}
/**
* returns a list of broken items.
* if there are no broken items return an empty list.
* No External Classes Permitted to Be Used in This Method.
* @return a list of items that are broken. If there is nothing in the list return empty item array.
*/
/**
* Damage the particular item at the index. If the item is destroyed (eg damage greater
* than the durability of the item. The item should be left in the list with status updated to broken.
* No External Classes Permitted to Be Used in This Method Apart from String.equals.
* @param index the item to damage
* @param damage the amount of damage
* @return true if the item was broken false if not found or not broken
*/
/**
* find the item with the particular name.
* Leave the item in the list and get a copy.
* No External Classes Permitted to Be Used in This Method Apart from String.equals
* @param nameToBeFound the name of the item to be found
* @return the item with the name or null if not found
*/
/**
* Repair the item of the name.
*
* The item will be repaired only if there is enough gold in the inventory
* to pay for it.
*
* The cost of the repair is 25% of the lost value of the durability
* For example
* If you had a widget that was worth 1000 gold coins
* and it was at 60% durability then its current value is 600 gold coins.
* It has lost 400 gold coins in value due to wear.
* If you repair the item it will cost 100 gold coins (25% of 400 gold coins).
*
* If there is not enough gold to repair the item it should not repair the item
* at all and return false. Otherwise it should remove the gold coins from the
* inventory and return true.
* @param nameToBeRepaired the name of the item to be repaired
* @return true if successfully repaired false otherwise
*/
/**
* Repair the item at the index.
*
* The item will be repaired only if there is enough gold in the inventory
* to pay for it.
*
* The cost of the repair is 25% of the lost value of the durability
* For example
* If you had a widget that was worth 1000 gold coins
* and it was at 60% durability then its current value is 600 gold coins.
* It has lost 400 gold coins in value due to wear.
* If you repair the item it will cost 100 gold coins (25% of 400 gold coins).
*
* If there is not enough gold to repair the item it should not repair the item
* at all and return false. Otherwise it should remove the gold coins from the
* inventory and return true.
* @param index of the item to be repaired
* @return true if successfully repaired false otherwise
*/
/**
* Add an item to the inventory if there is enough gold coins to add it
* if there isn't. don't add the item and return false.
* The cost is based on the current Value of the item.
* @param newItem the item to be bought and added to inventory
* @return true if successfully added false otherwise
*/
public boolean buyItem(Item newItem) {
}
/**
* Give the relative difference in value between two items to allow
* the caller to figure out if a trade is worth it.
* (note this method is static).
* Note that the first item is assumed to be yours so the value returned
* is how much you would be ahead if you do the trade
* @param myItem first item to compare
* @param theirItem second item to compare
* @return the difference in current values between item 1 and item 2 -1 if either were nulls
*/
/**
* Find the item on the inventory with the closest cost to the item
* being passed in. Remove the item being returned
* This is to simulate the person pulling out the item and looking at it.
* If they decide against the trade it they should re-add to the inventory
*
* Note the closest item should be the closest item under the price of the
* item to be traded. Eg the new value of itemToBeTraded is greater than the
* value of the item you return.
*
* if itemToBeTraded has a lower value to that to all items in the list return
* null.
* @param itemToBeTraded the item to be compared to.
* @return the most expensive item in the inventory under the new value of itemToBeTraded. Null if none found
*/
/**
* Return the number of items in the list.
* No External Classes Permitted to Be Used in This Method
* @return the number of items
*/
public int numberOfItems() {
}
/**
* return the index of the item with the given name. Case does not matter.
* No External Classes Permitted to Be Used in This Method Apart from
* String.Equals and String.ToUpperCase
* @param nameToBeFound the name of the item to be found
* @return the position of the searched for item. -1 if none found
*/
public int indexOf(java.lang.String nameToBeFound) {
}
/**
* ADVANCED STUDENTS ONLY
*
* Sort the array by item name. Note that if you are not attempting this method
* you must still implement the method signature so that the test program will run.
* No External Classes Permitted to Be Used in This Method Apart From String.CompareToIgnoreCase
* My Solution Length in Lines (note yours can be longer or shorter): 12
*
*/
public void sort() {
}
/**
* Creates a list of item with their hit points in the following form
*
* Item List
* 1. output of item toStringMethod
* 2. output of item toStringMethod
* 3. output of item toStringMethod
* 4. output of item toStringMethod
*
* Note the formatting is done so that the output lines up in the columns
* Please use the most appropriate classes to help you do this method.
*/
public String toString() {
String value = "";
for (int i=0;i value += itemList[i].toString()+" "; } return value; } /** * Debug function for testing that things are working ok. * Dumps a printout of the current state of the item array */ private void dumpAll() { for (int i=0;i System.out.println(itemList[i]); } } }
Step by Step Solution
There are 3 Steps involved in it
Step: 1
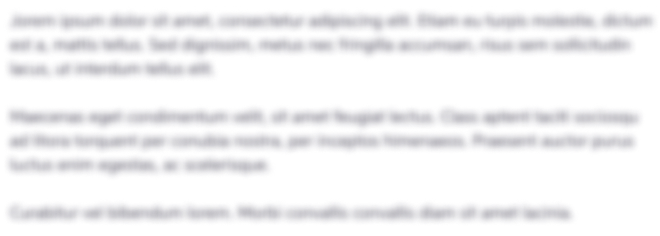
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started