Question
public class LinkedList { private class Node { private T data; private Node next, thread; private Node(T data) { this.data = data; next = thread
public class LinkedList
private class Node {
private T data;
private Node next, thread;
private Node(T data) {
this.data = data;
next = thread = null;
}
}
private Node head, tail;
public LinkedList() {
head = tail = null;
}
public void setThread(boolean pointToPrev) { /* YOU MUST IMPLEMENT */ }
Provide a RECURSIVE implementation for the setThread method. For this problem:
If pointToPrev is true, the method initializes the thread node reference of each node to the node that precedes it in the list. If the node is the first one, thread will be set to null. After the thread reference has been set, we will have a doubly-linked list.
If pointToPrev is false, the method initializes the thread node reference of every node (except the first one) to point to the first node in the list. The thread reference of the first node will be set to null.
No processing will take place if the list is empty.
Notice that thread has nothing to do with Java threads.
You will lose significant credit if you use any iteration statement (while, do while, for). Your solution must be recursive.
Notice the list is not a sorted list.
Driver
LinkedList
list.add("F").add("A").add("M").add("C").add("M").add("W");
System.out.println("list: " + list);
list.setThread(true);
System.out.print("After threading: ");
/* prints list starting at last element, following thread */
list.printListThread();
Output:
list: W M C M A F
After threading: F A M C M W
Step by Step Solution
There are 3 Steps involved in it
Step: 1
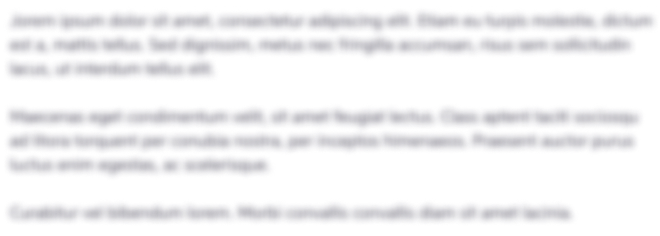
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started