Question
public class LinkedListWithoutDummy { private class Node { //inner class private Object data; private Node next; private Node(Object data, Node next) { this.data = data;
public class LinkedListWithoutDummy {
private class Node { //inner class
private Object data;
private Node next;
private Node(Object data, Node next) {
this.data = data;
this.next = next;
}
//second constructor
private Node(Object data){
this(data, null); //correct way to call an existing constructor
}
}//end of Node class
private Node head;
private int size;
public LinkedListWithoutDummy() {//create an empty linked list
this.head = null;
this.size = 0;
}
public Object remove(int index) throws IllegalArgumentException {
//we always assume the worst
if(index < 0 || index >= this.size)
throw new IllegalArgumentException("wrong index value!");
if(index == 0) {
Object temp = this.head.data;
this.head = this.head.next;
this.size --;
return temp;
}
Node cur = this.head, prev= null;
int i = 0;
while(i < index) {
prev = cur;
cur = cur.next;
i++;
}//walk into the list and find the node at the index you passed in.
System.out.println("I am here.");
//cut out
prev.next = cur.next;
this.size --;
return cur.data;
}//end of method
}
Question: examine and trace the method call remove(3) above in a linked list without dummy head node, using an input linked list as shown below. Node A is indexed as 0(zero). After the while loop drops out and the method is printing out "I am here.", what is the value for i, cur and prev at that moment?
possible answers:
i is 3; cur points to null; prev points to Node D.
i is 3; cur points to Node C; prev points to Node D.
i is 3; cur points to Node D; prev points to Node C.
i is 3; cur points to Node C; prev points to null.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
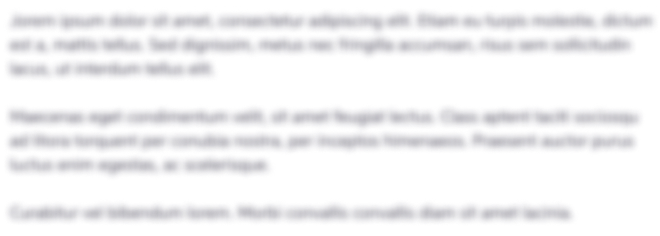
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started