Question
public class ScoreBoard { //number of games private int gameCount; //player records - you MUST use this for credit! //Do NOT change the name or
public class ScoreBoard { //number of games private int gameCount; //player records - you MUST use this for credit! //Do NOT change the name or type //NOTE: you cannot use any arrays or JCF instances in your implementation. private ThreeTenDynArray
return -1; //default return, remove or update as needed
} public int gameCount(){ //report number of games //O(1)
return -1; //default return, remove or update as needed }
public void addPlayer(PlayerRec player){ // append new player into record // if player is already present, updated the record // - assume each player has a unique name, i.e. matching names means same player // O(N) where N is the number of players present } public PlayerRec getPlayer(int index){ //return player record corresponding to index //return null for invalid indexes //O(1) return null; //default return, remove or update as needed }
public PlayerRec findPlayer(String name){ //find and return player record with the matching name //return null if not present // O(N) where N is the number of players present return null; //default return, remove or update as needed }
public boolean removePlayer(String name){ //remove player with the matching name //return true if a record is removed successfully; false otherwise // O(N) where N is the number of players present return false; //default return, remove or update as needed } public boolean changeScore(String name, int game, int newScore){ //set the score of the given game for the player with a matching name //return false if newScore cannot be set for any reason // (e.g player /game not present); return true otherwise // O(N) where N is the number of players present
return false; //default return, remove or update as needed } public int topTotalScore(){ //return largest total score among all players // return -1 if no player present // O(N) where N is the number of players present
return -3; //default return, remove or update as needed }
public ThreeTenDynArray
return null; //default return, remove or update as needed }
public int getGameTotal(int game){ // return the sum of all players' scores of the given game // - return -1 if no players present or game index is invalid // O(M) where M is the number of players return -10; //default return, remove or update as needed } public int getGameMax(int game){ // return the max score of the given game // - return -1 if no players present or game index is invalid // O(M) where M is the number of players return -10; //default return, remove or update as needed }
public int getGameMin(int game){ // return the min score of the given game // - return -1 if no players present or game index is invalid // O(M) where M is the number of players return -10; //default return, remove or update as needed
}
public boolean combine(ScoreBoard another, boolean append){ // add records from another scoreboard into the current one // - if a player is already present, update the record // - otherwise, // -if append is false, records from another should be added // to the start of the current records but keep their original order // -if append is true, add new records to the end of the list // Check main() below and description document for examples. // return false if two score boards cannot be combined (game count mismatch etc.); // return true otherwise // Although we do not have a big-O requirement for this method, you should // practice code reuse and utilize existing operations as much as possible. return false; //default return, remove or update as needed }
//****************************************************** //******* BELOW THIS LINE IS PROVIDED code ******* //******* Do NOT edit code! ******* //******* Remember to add JavaDoc ******* //******************************************************
public String gameToString(int game){ if (game<0 || game>gameCount()-1) return ("Game index " + game + " invalid!"); StringBuilder s = new StringBuilder("Game [" + game + "]: "); s.append(" "+ getGame(game).toString()); s.append(" Game Total: "+ getGameTotal(game) + " "); s.append(" Game Max: "+ getGameMax(game) + " "); s.append(" Game Min: "+ getGameMin(game) + " "); return s.toString().trim(); } @Override public String toString(){ StringBuilder s = new StringBuilder("%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%% "); s.append("Game Count: " + gameCount() + "\t"); s.append(" Player Count: " + playerCount() + " "); s.append("%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%%% "); for (int i=0; i
Step by Step Solution
There are 3 Steps involved in it
Step: 1
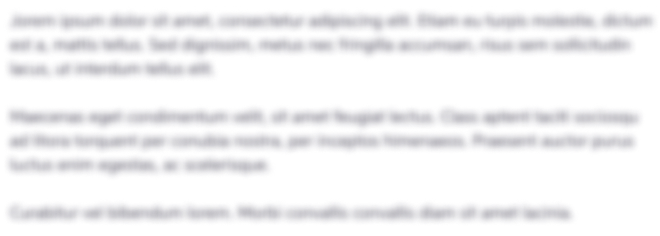
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started