Answered step by step
Verified Expert Solution
Question
1 Approved Answer
public class SortedLinkedList { /** * The 0th indexed Node in the list, the nth indexed Node in the list. */ Node head, tail; /**
public class SortedLinkedList { /** * The 0th indexed Node in the list, the nth indexed Node in the list. */ Node head, tail; /** * Creates a SortedLinkedList. */ public SortedLinkedList() { head = null; tail = null; } /** * Inserts the given data at a location that maintains sorted order (ascending). * @param data The value to be inserted into the list. */ public void insertSorted(int data) { } /** * Deletes the Node at the given index. * @param data Index of the int to be deleted. * @return If the data was deleted. */ public boolean delete(int data) { return false; } /** * Gets the int at the given index. Throws an IndexOutOfBoundException if index * is negative or too large. * @param idx Index of the int to be return. * @return The int at the given index */ public int get(int idx) { throw new IndexOutOfBoundsException(); } /** * Searches for the given int and returns its index if found. If the int is not * found, returns -1. * @param data The int to search for. * @return The data at the given index */ public int search(int data) { return -1; } @Override public String toString() { return printList(); } /** * A recursive helper for toString that generates a String representation of this. * @return A String representation of the this. */ private String printList() { String listStr = ""; String delimiter = ", "; Node cur = head; while (cur != null) { listStr += cur.data + delimiter; cur = cur.next; } return listStr.substring(0, listStr.length()-delimiter.length()); } }
different file----------------------------------------
public class Node { /** * The integer to be stored at the current Node. */ int data; /** * The next Node in the SortedLinkedList. */ Node next; /** * Creates a new Node and links it to null or the next Node in the SortedLinkedList. * @param data The integer to be stored at the current Node. * @param next The next Node in the SortedLinkedList. */ public Node(int data, Node next) { this.data = data; this.next = next; } }
---------------------------------------------
Please solve the bold texts
Step by Step Solution
There are 3 Steps involved in it
Step: 1
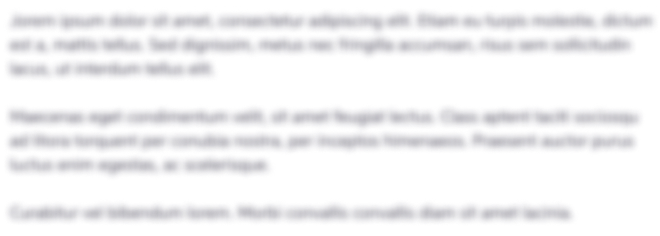
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started