Question
public class Square extends Shape{ double height; public Square(double l) { height = l; } public double getArea(){return height*height;} public String toString(){ return Square
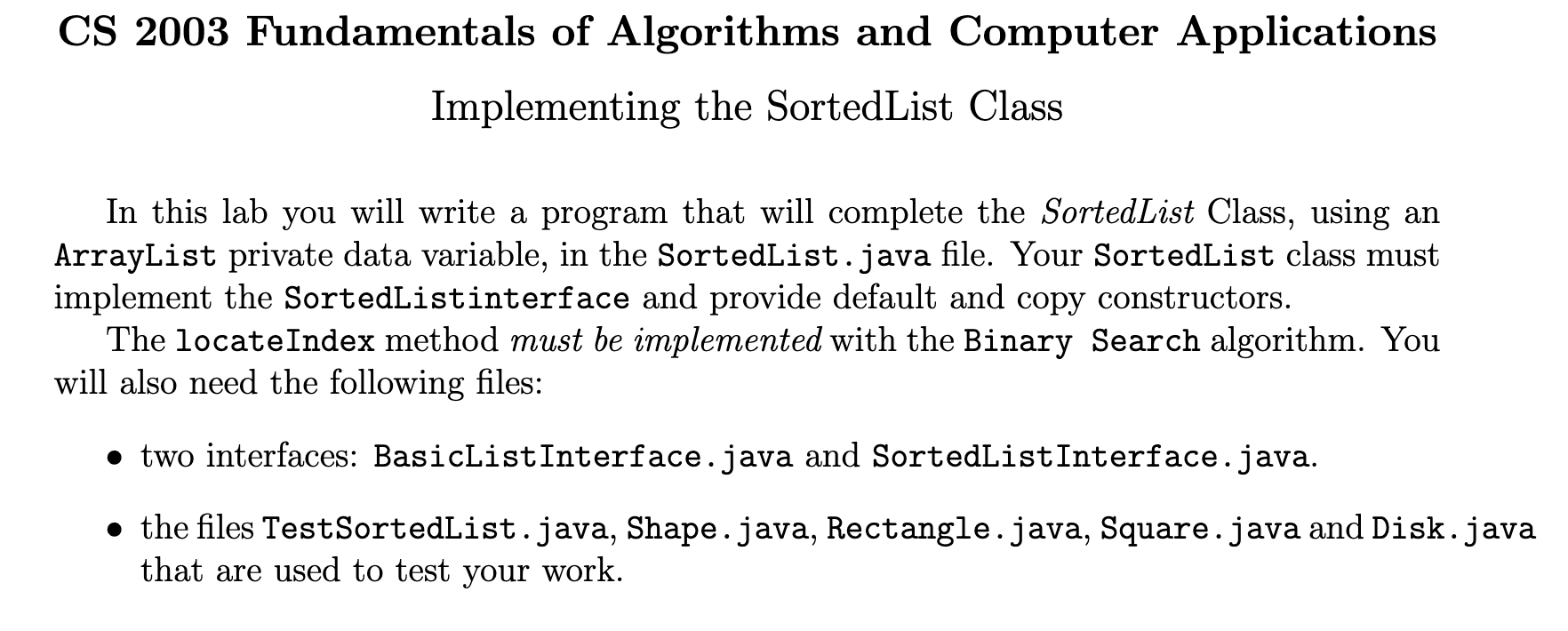
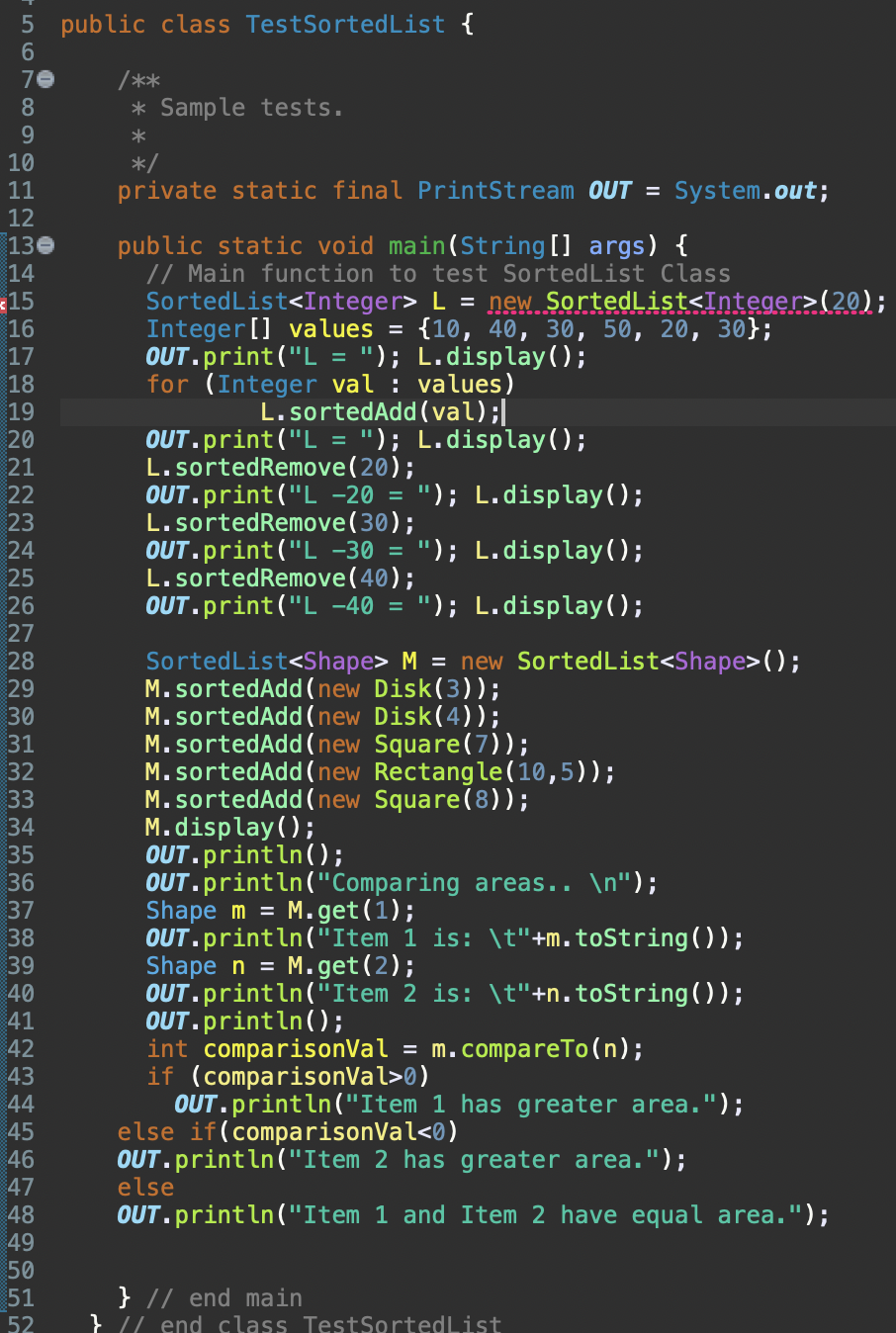
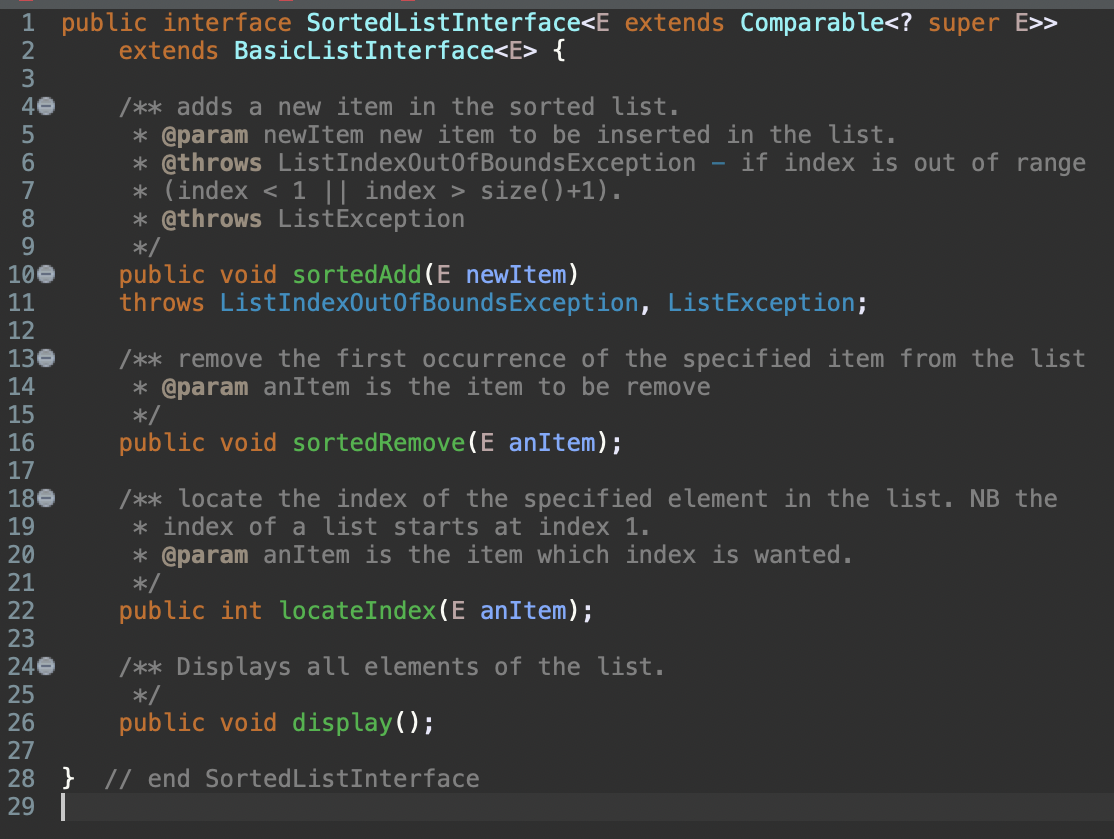
public class Square extends Shape{
double height;
public Square(double l) {
height = l;
}
public double getArea(){return height*height;}
public String toString(){
return "Square ("+height+")";
}
}
//new class
public abstract class Shape implements Comparable
public abstract double getArea();
public int compareTo(Shape s){
double areaS = s.getArea();
double areaThis = getArea();
if (areaThis < areaS)
return -1;
else if (areaThis == areaS)
return 0;
else
return +1;
}
public abstract String toString();
}
//new class
public class Rectangle extends Shape {
double length;
double height;
public Rectangle(double l, double h){
length = l;
height = h;
}
public double getArea(){
return length*height;
}
public String toString(){
return "Rectangle ("+length+"x"+height+")";
}
}
//new class
public class ListIndexOutOfBoundsException
extends IndexOutOfBoundsException {
public ListIndexOutOfBoundsException(String s) {
super(s);
} // end constructor
} // end ListIndexOutOfBoundsException
//new class
public class ListException extends RuntimeException {
public ListException(String s) {
super(s);
} // end constructor
} // end ListException
//new class
public class Disk extends Shape {
double radius;
public Disk(double r) {
radius = r;
}
public double getArea(){
return Math.PI*radius*radius;
}
public String toString(){
return "Disk ("+radius+")";
}
}
//new class
public interface BasicListInterface
/** Tests if this list has no elements.
* @return true
if this list has no elements;
* false
otherwise.
*/
public boolean isEmpty();
/**
* Returns the number of elements in this list.
* @return the number of elements in this list.
*/
public int size();
/** Removes all of the elements from this list.
*/
public void removeAll();
/**
* Returns the element at the specified position in this list.
* @param index index of element to return.
* @return the element at the specified position in this list.
* @throws IndexOutOfBoundsException - if index is out of range
* (index < 1 || index > size())
.
*/
public E get(int index)
throws ListIndexOutOfBoundsException;
} // end BasicListInterface
//new class, one that needs changed
import java.util.ArrayList;
public class SortedList
implements SortedListInterface
private ArrayList
} // end class
CS 2003 Fundamentals of Algorithms and Computer Applications Implementing the SortedList Class In this lab you will write a program that will complete the SortedList Class, using an ArrayList private data variable, in the SortedList.java file. Your SortedList class must implement the SortedListinterface and provide default and copy constructors. The locateIndex method must be implemented with the Binary Search algorithm. You will also need the following files: two interfaces: BasicListInterface.java and SortedList Interface.java. the files TestSortedList. java, Shape. java, Rectangle. java, Square. java and Disk.java that are used to test your work.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
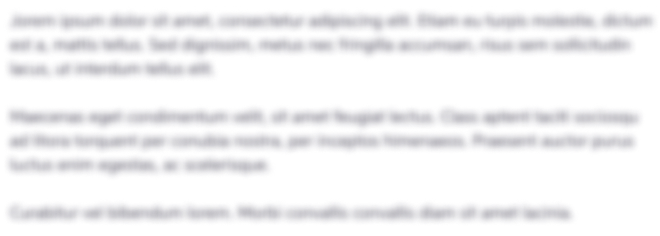
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started