Answered step by step
Verified Expert Solution
Question
1 Approved Answer
/ * * * Purpose: Simulates an ArrayList for Strings. MyStringArrayList * is an abstract data type ( ADT ) . * / public class
Purpose: Simulates an ArrayList for Strings. MyStringArrayList
is an abstract data type ADT
public class MyStringArrayList
Memory reference to the array that we use to store the data.
private String array;
The maximum amount the current array can hold. The default capacity is ten.
private static final int CAPACITY ;
Holds the current number of data in the array
private int size;
Sets the container capacity to be and size to
public MyStringArrayList
array new StringCAPACITY;
size ;
requires: e null
ensures : e added to the end of this array list.
Add a new element at the end of this list
@param e element to be added
public void addLastString e
ifisFull
increaseSize;
arraysize e; add element e to last empty location.
size; increment the size of this array list
This method is only used by this class.
@returns if this array list is full then it returns true, otherwise returns false.
private boolean isFull
return size array.length;
This method is only used by this class, and is thus detonated by private.
This method increases the size of the attribute 'array' by the CAPACITY.
Note: Java uses a garbage collector that will come through and remove the old reference we are overwriting in this method.
private void increaseSize
String temparray new Stringarraylength CAPACITY;
System.arraycopyarray temparray, array.length;
array temparray; make temparray the container
returns the current size # of elements of this list
requires: none
ensures: self.size #self.size
@return current size of this array list
public int getSize
return size;
Adds a String to the front of this Data Structure.
@param s any valid string
public void addFrontString s
TODO use add last as reference
if isFull increase capacity if array is full
String temp new Stringarraylength CAPACITY;
for int i ; i array.length; i
tempi arrayi;
array temp; make temp the container
for int i size; i ; i
arrayi arrayi ;
array s;
size;
Uses Linear search to see if param s is contained within the ArrayList.
@param s any valid string to search
@return boolean that tells the client if the param s was found.
public boolean containsString s
TODO
forint i;i size;i
ifarrayiequalss
return true;
return false;
Gets the element at the index i in the ArrayList. Index starts at
@param i integer specifies the index in the ArrayList that should be returned.
@return Returns null if out of bound or the String if it is found.
public String getElementint i
This should be implemented first
TODO
if i i size
return null;
return arrayi;
Removes the element at the index i
@param i Integer that specifies the index to be removed starting from
@return Returns the string being removed or null if it is out of bounds.
public String removeElementAtint i
TODO
if i i size
return null;
String e getElementi;
for int j i; j size ; j
arrayj arrayj ;
size;
return e;
This method converts the ArrayList to a string.
@return Passes a String representation of the list starting with ending with and each string separated by a comma.
public String toString
TODO
return ;
Step by Step Solution
There are 3 Steps involved in it
Step: 1
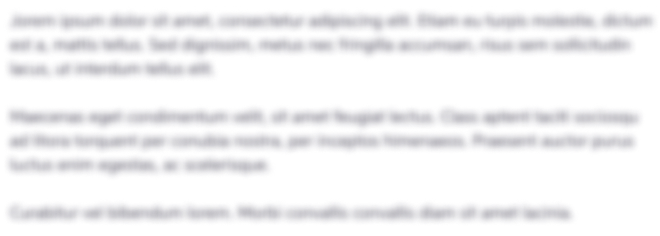
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started