Question
Python coding Assignment: Please make sure this compiles correctly.P In this assignment, you will work with lists. Begin with the useList.py starter file. This file
Python coding Assignment:
Please make sure this compiles correctly.P
In this assignment, you will work with lists.
Begin with the "useList.py" starter file. This file contains comment instructions that tell you where to add your code to do various tasks that use and manipulate lists.
Make sure you read the "Guidance" document.
Make sure your output matches the "Expected Output" document.
Guidance document:
Guidance ================================= General ------- Programmers must learn how to use frameworks. In this course, you are learning about the Textbook Collections Framework. You will not have the luxery to change the framework in any way. You must learn to do what you need to do with what is provided. Sometimes, this takes ingenuity and trial and error. Make sure you use the provided input.txt starter file to test your code. It must be located in the same folder as your Python file. Compare your results carefully to the "Programming Activity 5 - Expected Output" document. You have 6 parts to complete. Each part requires writing the code for the body of a function. Do not add, change, or delete any code outside of these function bodies. Each function already has all the parameters you might need. Make use of these parameters as needed within the function body. You do not need to use the parameter "words" in your code. As described in the function comments, "words" is a list of words. An iterator for this list, "wordsIter", is also passed to the functions. "wordsIter" would not make sense without describing what it iterates. Your code can make use of "wordsIter" to access the words list. However, you could access "words" directly in a "for" loop, if you want. Part 1 ------ Use Python string standard built-in functions to code this part. Keep in mind that strings are immutable. This means you can never actually change a string. However, you can get a changed copy. For example, suppose you want to convert a string named line to all uppercase. Using: line.upper() by itself is not enough. You need to use: line = line.upper() in order to get the changed copy. Part 2 ------ Call first() to prime the list iterator. Use a while loop with a hasNext() condition to traverse the words list. In the while block: Use next() to get the next word. Use "word" as the name of the variable to store this word. Determine if this word is in the removals list. To do this, use: if (word in removals): If the word is in the removals list: Call remove() on the list iterator to remove it from the words list. Part 3 ------ Introduce a variable called previousWord and initialize it to an empty string. Call first() to prime the list iterator. Use a while loop with a hasNext() condition to traverse the words list. In the while block: Use next() to get the next word. If the word is equal to "first" or word is equal to "last", then update the value of previousWord to be the current word and use continue to keep looping. If the word is equal to the previous word, call remove() on the list iterator. Update the value of previousWord to be the current word. Part 4 ------ This is similar to part 2. Instead of the removals list, use the misspellings list. Instead of using remove(): Call insert() on the list iterator to insert the flag text. Then call next() on the list iterator to move past the flag. Part 5 ------ The strategy here is to iterate through the list to: Count the number of "first" words. Remove each of the "first" words as they are encountered. Then use the count to control another loop to: Insert new "first" words at the beginning of the list. For each one: Call first() to prime the list iterator. If there is a next word, call next() to establish the insertion point. Call insert() to insert a "first" word. Since part 5 is one of the more difficult parts, here is its solution as a free gift: # Part 5: # Move all occurrences of "first" to the front of the words list. # Input: # words - an ArrayList of words, no uppercase, no punctuation # wordsIter - a list iterator for the words list def moveFirstLit(words, wordsIter): countFirst = 0 wordsIter.first() while (wordsIter.hasNext()): word = wordsIter.next() if (word == FIRST): wordsIter.remove() countFirst += 1 for count in range(countFirst): wordsIter.first() if (wordsIter.hasNext()): wordsIter.next() wordsIter.insert(FIRST) Make sure you study this code line by line. Make sure you understand everything about how it works. The FIRST constant (a convenience variable), is setup near the top of the starter code. Part 6 ------ The strategy here is to iterate through the list to: Count the number of "last" words. Remove each of the "last" words as they are encountered. Then use the count to control another loop to: Insert new "last" words at the end of the list. For each one: Call last() to establish the insertion point. Call insert() to insert a "last" word.
Expected output:
# articles first this is test case this is second test case first this is neither nor second test case abc this is articles test case last # certain prepositions first dog ran deer but second dog i will walk here there it is warm here and cold there rug is table and table is rug this is prepositions test case last # duplicate consecutive words (except "first" and "last") boat had blue hull boat had blue very blue hull first boat had blue blue hull it is best way go that thats all folks rolling river first first words and are intentionally not reduced last last my my my my my my this is consecutive words (except "first" and "last") test case last # certain misspelled words FLAG: foriegn concept that preceeds FLAG: thier duplicated FLAG: occurrance will FLAG: excede maximum allowed value FLAG: judgement of FLAG: thier souls music had moving FLAG: rythm this is misspelled words test case last # occurrences of "first" and "last" first shall be last first shall be last first first multiple place place and place place winners and losers last last first first first last last this is occurrences of "first" and "last" test case last
Starter files if needed:
File: useList.py
This program exercises lists.
Input: input.txt This file must be in the same folder.
Output: output.txt This file will be created in the same folder. All articles are removed. Certain prepositions are removed. Duplicate consecutive words are reduced to a single occurrence. Note: Duplicates of the words "first" and "last" are not reduced. Certain misspelled words are flagged. Occurrences of "first" are moved to the front of a line. Occurrences of "last" are moved to the end of a line.
The following files must be in the same folder: abstractcollection.py abstractlist.py arraylist.py arraylistiterator.py arrays.py linkedlist.py node.py input.txt - the input text file. """
#
# Replace
from arraylist import ArrayList from arraylistiterator import ArrayListIterator from linkedlist import LinkedList
# Data:
articles = ArrayList(["a", "the"]) prepositions = LinkedList(["after", "before", "from", "in", "off", "on", "under", "out", "over", "to"]) misspellings = ["foriegn", "excede", "judgement", "occurrance", "preceed", "rythm", "thier", ]
inputFile = open("input.txt", "r") outputFile = open("output.txt", "w")
# You should make use of these constant variables: FIRST = "first" FLAG = "FLAG:" LAST = "last"
# Processing:
# Part 1: # Simplifies a line by doing the following: # 1. Convert it to lowercase. # 2. Remove the following punctuation: # periods (.), commas (,), colons (:), semicolons (;), # exclamation marks (!), and question marks (?). # Input: # line - a line of text # Output: # returns the simplified line def simplifyLine(line): punctuation = ".,:;!?" # Nothing to do if the line is blank (whitespace): if (line.isspace()): return line #
# Part 2: # Removes all items from the words list that are found in the removals list. # Input: # words - an ArrayList of words # wordsIter - a list iterator for the words list # removals - the list of words to remove def removeItems(words, wordsIter, removals): #
# Part 3: # Removes extra occurrences of consecutive duplicate words from the words list. # Note: It does not reduce duplicates of the words "first" and "last". # Input: # words - an ArrayList of words # wordsIter - a list iterator for the words list def reduceDuplicates(words, wordsIter): #
# Part 4: # Flags certain misspelled words in the words list by inserting "FLAG:" before them. # Input: # words - an ArrayList of words # wordsIter - a list iterator for the words list # misspellings - the list of misspelled words to flag def flagMisspelled(words, wordsIter, misspellings): #
# Part 5: # Move all occurrences of "first" to the front of the words list. # Input: # words - an ArrayList of words # wordsIter - a list iterator for the words list def moveFirstLit(words, wordsIter): #
# Part 6: # Move all occurrences of "last" to the end of the words list. # Input: # words - an ArrayList of words, no uppercase, no punctuation # wordsIter - a list iterator for the words list def moveLastLit(words, wordsIter): #
def writeOutputLine(words): outputLine = " ".join(words) outputLine = outputLine + " " print(outputLine, end="") outputFile.write(outputLine)
# Main processing loop: for line in inputFile: line = simplifyLine(line) words = ArrayList(line.split()) wordsIter = words.listIterator()
# Make no changes to blank lines: if (len(words) == 0): writeOutputLine(words) continue
# Make no changes to comment lines: if (words[0] == "#"): writeOutputLine(words) continue
# Remove articles: removeItems(words, wordsIter, articles) # Remove prepositions: removeItems(words, wordsIter, prepositions)
# Reduce duplicate consecutive words to a single occurrence: reduceDuplicates(words, wordsIter)
# Insert "FLAG:" before certain misspelled words: flagMisspelled(words, wordsIter, misspellings) # Move all occurrences of "first" to the front of the line: moveFirstLit(words, wordsIter) # Move all occurrences of "last" to the end of the line: moveLastLit(words, wordsIter)
# Write output line: writeOutputLine(words)
# Wrap-up inputFile.close() outputFile.close() Input.text(don't think this one is needed it was provided though):
# Articles: this is the first test case this is a second test case this is neither the first nor a second test case the a the a the a abc the a the a the a This is the last articles test case.
# Certain prepositions: the first dog ran after the deer but before the second dog i will walk from here to over there it is warm in here and cold out there the rug is under the table and the table is over the rug on off under over This is the last prepositions test case.
# Duplicate consecutive words (except "first" and "last"): the boat had a blue blue hull the boat had a blue very blue hull the boat had a blue first blue hull it is is the best way to go go that that thats all folks rolling rolling rolling on the river the words first first and last last are intentionally not reduced my my my my my my my, my my my, my! my my. my, my: my; my! my? my My my My This is the last consecutive words (except "first" and "last") test case.
# Certain misspelled words: a foriegn concept that preceeds thier a duplicated occurrance will excede the maximum allowed value the judgement of thier souls the music had a moving rythm This is the last misspelled words test case.
# Occurrences of "first" and "last": the first shall be last the last shall be first multiple first place first place and last place last place winners and losers first first first last last This is the Last occurrences of "first" and "last" test case.
Step by Step Solution
There are 3 Steps involved in it
Step: 1
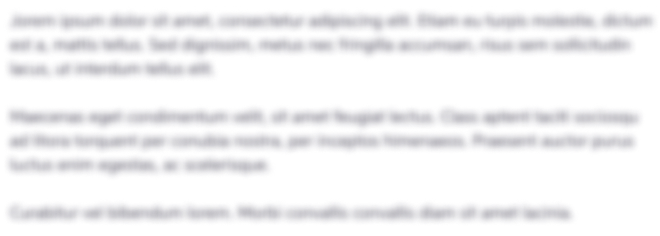
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started