Question
PYTHON!!! Implement a class intlist which a list that stores only integers. You MUST subclass list. Please note the following: constructor can be passed a
PYTHON!!! Implement a class intlist which a list that stores only integers. You MUST subclass list. Please note the following:
constructor can be passed a list of ints, or, by default constructs an empty intlist.
append, insert,extend can also be used to add ints to an intlist. If you dont know how extend works for a list, look it up. All should raise errors if a non-int is added.
__setitem__ - can be used for item assignment using an index. Raises error if non-int is used.
odds() write a method odds() which returns an intlist consisting of the odd ints. They should not be removed from the original.
evens() same as odds(), but for even ints
NotIntError also write an Exception class NotIntError that subclasses Exception.
NotIntError a NonIntError should be raised when client code attempts to place something other than an int in an intlist. This can happen in three ways (all shown in code below):
append
insert
The constructor when passed a list that contains something other an int
Note: you can check whether item is an int by evaluating the expression type(item)==int
Your goal is to get the following behavior:
>>> il = intlist()
>>> il
intlist([])
>>> il = intlist([1,2,3])
>>> il
intlist([1, 2, 3])
>>> il.append( 5 )
>>> il
intlist([1, 2, 3, 5])
>>> il.insert(1,99)
>>> il
intlist([1, 99, 2, 3, 5])
>>> il.extend( [22,44,66] )
>>> il
intlist([1, 99, 2, 3, 5, 22, 44, 66])
>>> odds = il.odds()
>>> odds
intlist([1, 99, 3, 5])
>>> evens = il.evens()
>>> evens
intlist([2, 22, 44, 66])
>>> il
intlist([1, 99, 2, 3, 5, 22, 44, 66])
>>> il[2] = -12 # calls __setitem__
>>> il
intlist([1, 99, -12, 3, 5, 22, 44, 66])
>>> il[4] # calls __getitem__
5
Trying to put anything except for an int into an intlist will always raise an NotIntError. Note that there 5 different ways this could be attempted:
>>> il.append(33.4)
Traceback (most recent call last):
...
NotIntError: 33.4 not an int
>>> il.insert(2,True)
Traceback (most recent call last):
...
NotIntError: True not an int
>>> il = intlist([2,3,4,"apple"])
Traceback (most recent call last):
...
NotIntError: apple not an int
>>> il.extend( [2,3,'hello'])
Traceback (most recent call last):
...
NotIntError: hello not an int
>>> il[2] = 22.3
Traceback (most recent call last):
...
NotIntError: 22.3 not an int
Step by Step Solution
There are 3 Steps involved in it
Step: 1
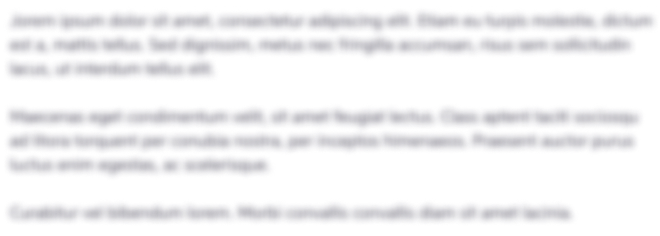
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started