Question
Python In this assignment, you will implement an online banking system. Users can sign-up with the system, log in to the system, change their password,
Python
In this assignment, you will implement an online banking system. Users can sign-up with the system, log in to the system, change their password, and delete their account. They can also update their bank account balance and transfer money to another users bank account.
Youll implement functions related to File I/O and dictionaries. The first two functions require you to import files and create dictionaries. User information will be imported from the users.txt file and account information will be imported from the bank.txt file. Take a look at the content in the different files. The remaining functions require you to use or modify the two dictionaries created from the files.
Each function has been defined for you, but without the code. See the docstring in each function for instructions on what the function is supposed to do and how to write the code. It should be clear enough. In some cases, we have provided hints to help you get started.
def signup(user_accounts, log_in, username, password): ''' This function allows users to sign up. If both username and password meet the requirements, updates the username and the corresponding password in the user_accounts, and returns True. If the username and password fail to meet any one of the following requirements, returns False. - The username already exists in the user_accounts. - The password must be at least 8 characters. - The password must contain at least one lowercase character. - The password must contain at least one uppercase character. - The password must contain at least one number. - The username & password cannot be the same. For example: - Calling signup(user_accounts, log_in, "Brandon", "123abcABCD") will return False - Calling signup(user_accounts, log_in, "BrandonK", "123ABCD") will return False - Calling signup(user_accounts, log_in, "BrandonK","abcdABCD") will return False - Calling signup(user_accounts, log_in, "BrandonK", "123aABCD") will return True. Then calling signup(user_accounts, log_in, "BrandonK", "123aABCD") again will return False. Hint: Think about defining and using a separate valid(password) function that checks the validity of a given password. This will also come in handy when writing the change_password() function. ''' # your code here
########################## ### TEST YOUR SOLUTION ### ########################## user_accounts, log_in = import_and_create_accounts("user.txt")
tools.assert_false(len(user_accounts) == 0) tools.assert_false(len(log_in) == 0) tools.assert_equal(user_accounts.get("Brandon"),"brandon123ABC") tools.assert_equal(user_accounts.get("Jack"),"jack123POU") tools.assert_is_none(user_accounts.get("Jennie")) tools.assert_false(log_in["Sarah"])
#user.txt
Brandon - brandon123ABC Jack Jack - jac123 Jack - jack123POU Patrick - patrick5678 Brandon - brandon123ABCD James - 100jamesABD Sarah - sd896ssfJJH Jennie - sadsaca
#bank.txt
Brandon: 5 Patrick: 18.9 Brandon: xyz Jack:
Sarah: 825 Jack : 45 Brandon: 10 James: 3.25 James: 125.62 Sarah: 2.43
Brandon: 100.5
Step by Step Solution
There are 3 Steps involved in it
Step: 1
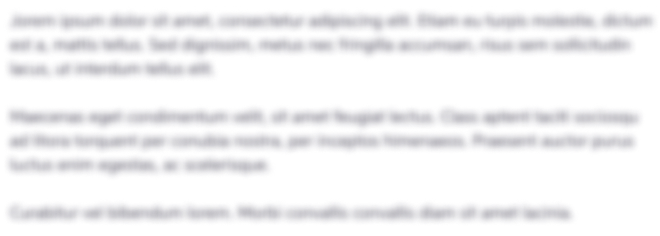
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started