Question
python language. This program uses a few lists to store data. To make grading easier, data will be added to these lists at the beginning
python language.
This program uses a few lists to store data. To make grading easier, data will be added to these lists at the beginning of the main function.
student_list = [('1001', '111'), ('1002', '222'), ('1003', '333'), ('1004', '444')] course_list = ['CSC101', 'CSC102', 'CSC103']
max_size_list = [3, 2, 1] roster_list = [['1004', '1003'], ['1001'], ['1002']]
There are 4 students in this program. ID and PIN of students are stored as tuples in student_list. The first element of each tuple is student ID, while the second element is PIN.
Three courses are offered. The course codes are stored in course_list. These courses are CSC101, CSC102 and CSC103.
The maximum class size of the courses offered are stored in max_size_list. The max sizes of CSC101, CSC102 and CSC103 are 3, 2 and 1, respectively.
Rosters of the three classes offered are stored as three lists, which are three elements of roster_list, which is actually a list of lists. Students 1004 and 1003 are enrolled in CSC101. Student 1001 is enrolled in CSC102. Student 1002 is enrolled in CSC103.
The program should have a loop to create multiple student sessions. In each session, ask user to enter ID, then call the login function to verify the students identity. If login is successful, use a loop to allow the student to add courses, drop courses and list courses registered.
The following is an example.
Enter ID to log in, or 0 to quit: 1234
Enter PIN: 123
ID or PIN incorrect
Enter ID to log in, or 0 to quit: 1001
Enter PIN: 111
ID and PIN verified
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 1
Enter course you want to add: CSC121
Course not found
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 1
Enter course you want to add: CSC102
You are already enrolled in that course.
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 1
Enter course you want to add: CSC103
Course already full.
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 1
Enter course you want to add: CSC101
Course added
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 2
Enter course you want to drop: CSC121
Course not found
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 2
Enter course you want to drop: CSC103
You are not enrolled in that course.
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 2
Enter course you want to drop: CSC102
Course dropped
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 3
Courses registered:
CSC101
Total number: 1
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 1
Enter course you want to add: CSC102
Course added
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 3
Courses registered:
CSC101
CSC102
Total number: 2
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 0
Session ended.
Enter ID to log in, or 0 to quit: 1002
Enter PIN: 222
ID and PIN verified
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 3
Courses registered:
CSC103
Total number: 1
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 1
Enter course you want to add: CSC101
Course already full.
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 1
Enter course you want to add: CSC102
Course added
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 3
Courses registered:
CSC102
CSC103
Total number: 2
Enter 1 to add course, 2 to drop course, 3 to list courses, 0 to exit: 0
Session ended.
Enter ID to log in, or 0 to quit: 0
Function add...course(id, r_list, m list) list, Specification This function adds a student to a course. It has four parameters: id is the ID of the student to be added; c list is the list of courses offered; r list is the list of class rosters; m list is the list of maximum class sizes. This function asks user to enter the course he/she wants to add. If the course is not offered, display error message and stop. If the course is full, display error message and stop. If student already registered for the course, display error message and stop. Add student ID to the course's roster and display a message if there is no problem. This function has no return value. This function drops a student from a course. It has three parameters: id is the ID of the student to be dropped; c list is the list of courses offered; r list is the list of class rosters. This function asks user to enter the course he/she wants to drop. If the course is not offered, display error message and stop. If the student is not enrolled in that course, display error message and stop. Remove student ID from the course's roster and display a message if there is no problem. This function has no return value. list, drop...course(id, r_list) list courses(id, clist, r_list) This function displays and counts courses a student has registered for. It has three parameters: id is the ID of the student; c. list is the list of courses offered; r list is the list of class rosters. This function displays the number of courses the student has registered for and which courses they are. This function has no return value. tream You must define the following functions in the main module. Function login(id, sulist) Specification This function allows a student to log in. It has two parameters: id and s list, which is the student list. This function asks user to enter PIN. If the ID and PIN combination is in s list, display message of verification and return True. Otherwise, display error message and return false. main() This function manages the whole registration system. It has no parameter. It creates 4 lists to store data: student list, course list, maximum class size list and roster list. It uses a loop to serve multiple students. Inside the loop, ask user to enter ID, and call the login function to verify student's identity. If login is successful, use a loop to allow student choose to add courses, drop courses or list courses the student has registered for. This function has no return value. Function add...course(id, r_list, m list) list, Specification This function adds a student to a course. It has four parameters: id is the ID of the student to be added; c list is the list of courses offered; r list is the list of class rosters; m list is the list of maximum class sizes. This function asks user to enter the course he/she wants to add. If the course is not offered, display error message and stop. If the course is full, display error message and stop. If student already registered for the course, display error message and stop. Add student ID to the course's roster and display a message if there is no problem. This function has no return value. This function drops a student from a course. It has three parameters: id is the ID of the student to be dropped; c list is the list of courses offered; r list is the list of class rosters. This function asks user to enter the course he/she wants to drop. If the course is not offered, display error message and stop. If the student is not enrolled in that course, display error message and stop. Remove student ID from the course's roster and display a message if there is no problem. This function has no return value. list, drop...course(id, r_list) list courses(id, clist, r_list) This function displays and counts courses a student has registered for. It has three parameters: id is the ID of the student; c. list is the list of courses offered; r list is the list of class rosters. This function displays the number of courses the student has registered for and which courses they are. This function has no return value. tream You must define the following functions in the main module. Function login(id, sulist) Specification This function allows a student to log in. It has two parameters: id and s list, which is the student list. This function asks user to enter PIN. If the ID and PIN combination is in s list, display message of verification and return True. Otherwise, display error message and return false. main() This function manages the whole registration system. It has no parameter. It creates 4 lists to store data: student list, course list, maximum class size list and roster list. It uses a loop to serve multiple students. Inside the loop, ask user to enter ID, and call the login function to verify student's identity. If login is successful, use a loop to allow student choose to add courses, drop courses or list courses the student has registered for. This function has no return valueStep by Step Solution
There are 3 Steps involved in it
Step: 1
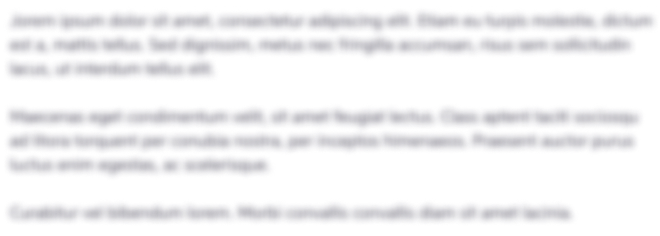
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started