Question
PYTHON PROGRAMMING . import uuid import random def make_class(attrs, base=None): def make_instance(cls): def bind(val, instance): if callable(val): def method(*args): return val(instance, *args) return method return
PYTHON PROGRAMMING .
import uuid import random
def make_class(attrs, base=None): def make_instance(cls): def bind(val, instance): if callable(val): def method(*args): return val(instance, *args)
return method return val
def get_v(attr_name): if attr_name in attrs: # Object attribute return attrs[attr_name]
# Class attribute val = cls['get'](attr_name) return bind(val, instance)
def set_v(attr_name, val): attrs[attr_name] = val def get_uuid(): return uuid.UUID(bytes=bytes(random.getrandbits(8) for _ in range(16)), version=4)
# FIXME: Edit `get_hash()` to return hash of object def get_hash(): return None
# FIXME: Edit `is_same(other)` to return whether this object is the # same as other def is_same(other): return None
# FIXME: Edit `clone_o()` to return the clone of this object def clone_o(): return None
# FIXME: Generate a hash for this function using the `get_uuid()` function hashcode = None
# FIXME: Edit the instance dictionary to add `hash`, `is_same` and `clone` # functions attrs = {} instance = { 'get': get_v, 'set': set_v, }
return instance
def init(cls, *args): obj = make_instance(cls) init_m = cls['get']('__init__')
if init_m: init_m(obj, *args)
return obj
def get_a(attr_name): if attr_name == 'super': return base elif attr_name in attrs: return attrs[attr_name] elif base is not None: return base['get'](attr_name)
def set_a(attr_name, val): attrs[attr_name] = val
def new_o(*args): return init(cls, *args) random.seed('EEE111')
cls = { 'get': get_a, 'set': set_a, 'new': new_o, }
return cls
# Don't edit anything below this to make your life easier. :) def main(): def DynamicClassFactoryMaker(fun_name, props_dict): def f(): def __init__(self): for prop_name, (prop_val, is_get, is_set) in props_dict.items(): self['set'](prop_name, prop_val) self['modify'](prop_name, is_get, is_set) return make_class(locals()) f.__name__ = fun_name return f n_testcases = int(input())
for t in range(n_testcases): obj_dict = {}
n_commands = int(input()) class_name = input() DynamicClassFactory = DynamicClassFactoryMaker(class_name, {}) DynamicClass = DynamicClassFactory()
print(f'Case #{t + 1}:') for _ in range(n_commands): cmd_line = input().split()
if cmd_line[0] == 'create': obj_name = cmd_line[1]
class_obj = DynamicClass['new']() obj_dict[obj_name] = class_obj elif cmd_line[0] == 'hash': obj_name = cmd_line[1]
print(obj_dict[obj_name]['hash']()) elif cmd_line[0] == 'clone': a, b = cmd_line[1:]
obj_dict[b] = obj_dict[a]['clone']() elif cmd_line[0] == 'is_equal': a, b = cmd_line[1:] print(obj_dict[a]['is_same'](obj_dict[b]))
if __name__ == '__main__': main()
Composing Programs has introduced us to a way to implement an OOP system in programming languages that don't have it. Together with the current lesson, the OOP system can do the following: Make a class Bind the self object to a class method Instantiate objects of a class Run an __init__0 routine during instantiation of an object Make a class inherit from a base class Get its superclass from a class method Now, we are interested in augmenting our system to be able to compare two objects. Recognizing that (at least initially) no two objects from the same class are created, we can assign an ID to each object instantiated. This hashcode is used by some languages, such as Java, to implement the equality operator between objects. In this challenge, we will be emulating this in Python. We will be assigning each object with a hash based on a pseudorandom universally unique identifier (UUID). This hash can be retrieved using the hash function. To check whether two objects are the same, we will be implementing an is same function to check whether their hashes are the same. Finally, we will be implementing a clone function to duplicate an object. There are several ways to "clone" an object, but we will only implement a very simple one that makes a new object with the same hash as itself. Input Format The first line of the input is an integer T denoting the number of classes (or testcases) that will follow. Each testcase starts with a line containing a single number denoting the number of commands ne to execute for this testcase. The next line contains the name of the class class_name to create. The next ne lines denote the commands to execute. Each line consists of an operation op followed by some operands. op can only be from this set: create, hash, is_equal, clone. The format of a line with each keyword is shown below, with a and bas snake-case variable names. create a - create an object a of class class_name hash a-get hash of object a is equal a b - compare two objects a and b clone a b-copy object a into a new object . a - Note that each line in a set of commands is executed in order. For example, the code below shows means that object a is created first, then its hash is printed, and then the object b is then created. create a hash a create b hash b Constraints Input Constraints 1Step by Step Solution
There are 3 Steps involved in it
Step: 1
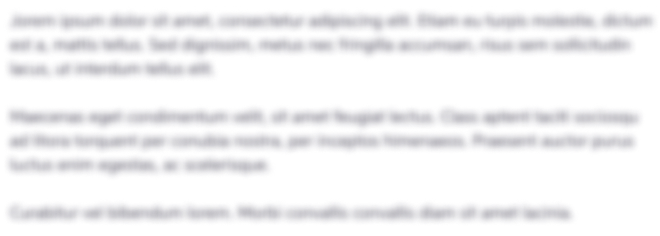
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started