Question
Python programming question: Build a simple checkout system, the requirements are: (1) Build an Item class with the following specifications: Attributes (2 pts) item_name (string)
Python programming question:
Build a simple checkout system, the requirements are:
(1) Build an Item class with the following specifications:
- Attributes (2 pts)
- item_name (string)
- item_price (float)
- item_quantity (int)
(2) Add a default constructor to the Item class. The constructor should Initialize the item's name = "Empty", item's price = 0.0, item's quantity = 0. (1 pt)
(3) Add a method to Item called print_item_cost() that prints the item name, the item quantity, the unit price and total price for this item. The prices should have two decimal points. (1 pt)
Example of the print_item_cost() output:
Bottled Water 10.00 @ $1 = $10.00
(4) Build a Checkout class with the following data attributes and related methods. (2 pts)
- Constructor which takes the customer name and date as parameters
- Attributes
- customer_name (string) - Initialized in default constructor to "None"
- date (string) - Initialized in default constructor to "January 1, 1970"
- cart_items (list)
- Methods
- add_item()
- Adds an item to cart_items list. Takes Item as a parameter. Does not return anything.
- remove_item()
- Removes item from cart_items list. Takes a string (an item's name) as a parameter. Does not return anything.
- If item name cannot be found, output this message: Item not found. No changes.
- modify_item()
- Modifies an item's quantity. Has parameter Item. Does not return anything.
- If item can be found (by name) in the list, modify item in cart.
- If item cannot be found (by name) in cart, output this message: Item not found. No changes.
(5) In the main section of your program, prompt the user for a customer's name and today's date. Create an object of type Checkout. Create a function call print_cart() that rakes the Checkout instance as a parameter and prints the information in Checkout (it will have no items at this point). (2 pts) Example output:
Enter customer's name: Joe Doe Enter today's date: May 13, 2019 CART: Customer name: Joe Doe Today's date: May 13, 2019
(6) In the main section of your code, output a menu of options to manipulate the shopping cart. Each option is represented by a single character. Build and output the menu within the function.
If the an invalid character is entered, continue to prompt for a valid choice. Call print_menu() in the main() function. Continue to execute call print_menu() until the user enters q to Quit. (3 pts) Example output:
Enter customer's name: Joe Doe Enter today's date: May 13, 2019 CART: Customer name: Joe Doe Today's date: May 13, 2019 Total: $0.00 MENU a - Add item to cart r - Remove item from cart c - Change item quantity q - Quit Choose an option:
(7) Implement Add item to cart menu option. (3 pts) Example of output:
Enter customer's name: Joe Doe Enter today's date: May 13, 2019 CART: Customer name: Joe Doe Today's date: May 13, 2019 MENU a - Add item to cart r - Remove item from cart c - Change item quantity q - Quit Choose an option: a ADD ITEM TO CART Enter the item name: Running shoes Enter the price: 97 Enter the quantity: 2 CART: Customer name: Joe Doe Today's date: May 13, 2019 Running Shoes 2 @ $97.00 = $194.00 Total: $194.00
(8) Implement remove item menu option. (3 pts) Example of output:
Enter customer's name: Joe Doe Enter today's date: May 13, 2019 CART: Customer name: Joe Doe Today's date: May 13, 2019 Total: $0.00 MENU a - Add item to cart r - Remove item from cart c - Change item quantity q - Quit Choose an option: a ADD ITEM TO CART Enter the item name: Laptop Enter the price: 499 Enter the quantity: 1 CART: Customer name: Joe Doe Today's date: May 13, 2019 Laptop 1 @ $499.00 = $499.00 Total: $499.00 MENU a - Add item to cart r - Remove item from cart c - Change item quantity q - Quit Choose an option: r REMOVE ITEM FROM CART Enter name of item to remove: Laptop CART: Customer name: Joe Doe Today's date: May 13, 2019 Total: $0.00
(9) Implement Change item quantity menu option. (3 pts) Example of output:
Enter customer's name: Joe Doe Enter today's date: May 13, 2019 CART: Customer name: Joe Doe Today's date: May 13, 2019 Total: $0.00 MENU a - Add item to cart r - Remove item from cart c - Change item quantity q - Quit Choose an option: a ADD ITEM TO CART Enter the item name: Skateboard Enter the price: 109 Enter the quantity: 1 CART: Customer name: Joe Doe Today's date: May 13, 2019 Skateboard 1 @ $109.00 = $109.00 Total: $109.00 MENU a - Add item to cart r - Remove item from cart c - Change item quantity q - Quit Choose an option: c CHANGE ITEM QUANTITY Enter the item name: Skateboard Enter the new quantity: 3 CART: Customer name: Joe Doe Today's date: May 13, 2019 Skateboard 3 @ $109.00 = $327.00 Total: $327.00
Step by Step Solution
There are 3 Steps involved in it
Step: 1
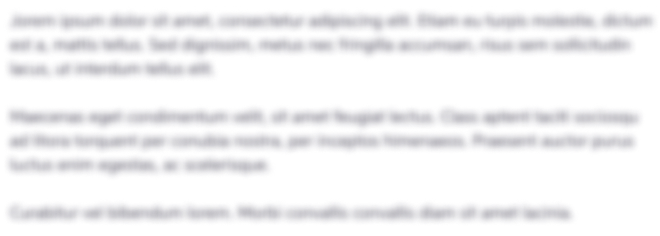
Get Instant Access with AI-Powered Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started