Question
Convert the Baseball Team Manager program from procedural toobject-oriented. This shouldn?t change the functionality of thecode much, but it should make the code more modular,
Convert the Baseball Team Manager program from procedural toobject-oriented. This shouldn?t change the functionality of thecode much, but it should make the code more modular, reusable, andeasier to maintain.
Sample Console Output
- Use a Player class that provides attributes that store thefirst name, last name, position, at bats, and hits for a player.The class constructor should use these five attributes asparameters. This class should also provide a method that returnsthe full name of a player and a method that returns the battingaverage for a player.
- Use a Lineup class to store the lineup for the team as aprivate list. This class should include methods with appropriateparameters that allow youto add, remove, move,and get a player. The add method willadd a new player to the end of the list. The remove and get methodsrequire the lineup location of the targeted player. In addition, itmust include an iterator so that thelineup object can be used in a for loop.
- Use a file named ui to store thecode for the user interface.
- Use a file named objects to storethe code for the Player and Lineup classes.
- Use a file named db to store thefunctions that work with the file that stores the data. Use alineup object to hold the player objects that are created when thedata is read from the players.txt file.
- current code is to import a csv file I need it toimport a txt file
- sample txt info:
Dominick, Gilbert,1B,537,170Craig, Mitchell,CF,396,125Jack, Quinn,RF,345,99Simon, Harris,C,450,135Darryl, Moss,3B,501,120Grady, Guzman,SS,463,114Wallace, Cruz,LF,443,131Cedric, Cooper,2B,165,54Alberto, Gomez,P,72,19
def load_from_file(files, FILE_NAME):try:database = []inp = open(FILE_NAME , "r")line = inp.readline()except FileNotFoundError:print ("ERROR - Unable to open {}".format(FILE_NAME))quit()while(line):player = line.strip().split(",") if(len(player) == 4):valid = files.valid_POS(player[1])allow = Trueif(not valid):if(allow):print("Error(s) Occured in Player with details:",line)print("Position is Invalid")allow = Falsevalid = files.valid_at_bats(player[2])if(not valid):if(allow):print("Error(s) Occured in Player with details:",line)print("At bat is Invalid")allow = Falsevalid = files.valid_H(player[3])if(not valid):if(allow):print("Error(s) Occured in Player with details:",line)print("Invalid Hits")allow = Falsevalid = files.valid_AVG(player[3])if(allow):database.append(player)else:print("Invalid text:",line)line = inp.readline()inp.close()return database
def save_to_file(files, FILE_NAME, database):try:output = open(FILE_NAME , "w", encoding = "utf-8")except FileNotFoundError:print ("ERROR - Unable to open {}".format(FILE_NAME))quit()for data in database:data = ','.join(list(map(str, data)))print(data, file = output)
output.close()
Python project:
CURRENT DATE: GAME DATE: DAYS UNTIL GAME: 7 MENU OPTIONS 1 Display lineup 2 Add player 3 Remove player 4 Move player 5 6 7 2020-07-12 2020-07-19 Baseball Team Manager Edit player position Edit player stats Exit program Menu option: 1 Player POSITIONS C, 1B, 2B, 3B, SS, LF, CF, RF, P Menu option: 1 Dominick Gilbert Craig Mitchell 2 3 Jack Quinn 4 Simon Harris 5 Darryl Moss 6 Grady Guzman 7 Wallace Cruz 8 Cedric Cooper 9 Alberto Gomez POS 1B CF RF 3B SS LF 2B P AB H 545 174 533 127 477 475 215 103 0.319 0.238 176 0.329 174 0.359 0.235 535 485 532 125 AVG 122 138 58 21 0.256 0.291 0.270 0.204
Step by Step Solution
3.43 Rating (153 Votes )
There are 3 Steps involved in it
Step: 1
The objectspy file is written as follows The dbpy file is written as follows The ...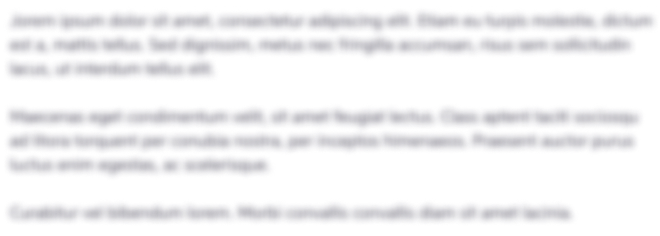
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started