Question
Python ''' The goal of this program is to maintain a student roster. The only data stored for each student is a single int variable
Python
''' The goal of this program is to maintain a student roster. The only "data" stored for each student is a single int variable representing the number of absences the student has. student_absences is the name + absence count stored as a dictionary. I'm starting you out with a small class. The rest of the functionality is in the functions below.
Note: As always, don't overthink this and take it one step at a time. I added less than 15 lines of code to accomplish all three functions. Your solution shouldn't be much bigger. ''' student_absences = { "The Batman": 0, "Robin": 0, "The Joker": 0, "The Catwoman": 0, "The Penguin": 0 }
''' For all of these functions, your goals is to replace the "pass" statement with meaningful logic. '''
def display_roster(): ''' This function should simply display each student in the class followed by at least one space followed by the number of absences that student has accumulated. One student + absence count per line.
As a challenge, you might try to look ahead at how to add string formmating codes to f-strings and see if you can align the names and spaces neatly (e.g., left align the names in 30 spaces followed by the number of absences) ''' global student_absences pass
def add_to_roster(name): ''' This function must add a new student to the roster and set the initial absences for that new student to 0. It should then simply print "{name} has been added to the roster". BUT, be careful. If the name already exists, do NOT attempt to add it. Instead print "{name} is already enrolled".
Though not required, you might want to "normalize" the names by title-casing them prior to adding them. ''' global student_absences pass
def mark_absent(name): ''' This function should simply increment a student's current number of absences. It should then print "{name} now has {absence count} absences" (noting that the absence count is now the NEW, updated one).
If the name does NOT exist in the roster, you should simply print "{name} does not exist".
Though not required (and only if you did it in add_to_roster), you might also here want to title-case the name parameter prior to checking to see if it is in the roster. ''' global student_absences pass
while True: print("1) Display Roster") print("2) Add To Roster") print("3) Mark Absent") print("T) Quick Test") print("X) Exit")
choice = input('CHOICE: ').strip().upper()
if choice == "1": display_roster() elif choice == "2": name = input("ENTER NAME: ").strip() add_to_roster(name) elif choice == "3": name = input("ENTER NAME: ").strip() mark_absent(name) elif choice == "T": print("QUICK TEST...SEE THE COMMENTS TO MAKE SURE ALL IS WORKING PROPERLY") display_roster() # SHOULD ADD THE FIRST TIME, ERROR MESSAGE ALL SUBSEQUENT TIMES add_to_roster("The Riddler") # SHOULD ALWAYS GIVE AN ERROR MESSAGE add_to_roster("The Batman")
# SHOULD INCREMENT THE ABSENCE COUNT BY ONE AND DISPLAY IT mark_absent("The Batman")
# INSURE THAT THE ABSENT COUNT FOR "The Batman" IS "REMEMBERED" # (i.e., it's the same as was just displayed...NOT 0) display_roster()
# SHOULD ALWAYS GIVE AN ERROR MESSAGE mark_absent("Superman")
elif choice == "X": break else: print("BAD CHOICE...TRY AGAIN")
print("See you soon. Same bat-time. Same bat-channel.")
''' JUST FYI:
My first (T)est run: QUICK TEST...SEE THE COMMENTS TO MAKE SURE ALL IS WORKING PROPERLY The Batman 0 Robin 0 The Joker 0 The Catwoman 0 The Penguin 0 The Riddler has been added to the roster The Batman is already enrolled The Batman now has 1 absences The Batman 1 Robin 0 The Joker 0 The Catwoman 0 The Penguin 0 The Riddler 0 Superman does not exist
My second (T)est run: QUICK TEST...SEE THE COMMENTS TO MAKE SURE ALL IS WORKING PROPERLY The Batman 1 Robin 0 The Joker 0 The Catwoman 0 The Penguin 0 The Riddler 0 The Riddler is already enrolled The Batman is already enrolled The Batman now has 2 absences The Batman 2 Robin 0 The Joker 0 The Catwoman 0 The Penguin 0 The Riddler 0 Superman does not exist '''
Step by Step Solution
There are 3 Steps involved in it
Step: 1
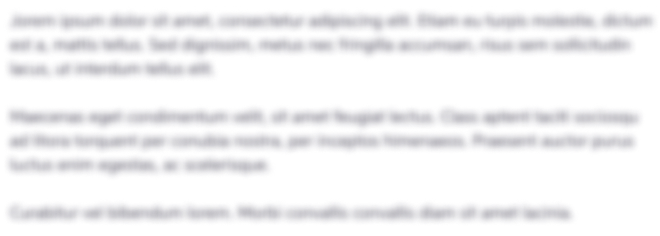
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started