Question
Python: Tower of hanoi must contain 4 py. Files (towers_of_hanoi.py)(tower.py)(disk.py)(toh_main.py) Please help This program plays the Towers of Hanoi game with different numbers of Disks
Python: Tower of hanoi must contain 4 py. Files (towers_of_hanoi.py)(tower.py)(disk.py)(toh_main.py) Please help
This program plays the Towers of Hanoi game with different numbers of Disks ranging from 3 to 24. Each time the game is played, the number of moves is recorded, and the state of Towers is displayed with every move. The mainline code is in the toh_main.py file. It plays each of the 22 TowersOfHanoi games.
This program must create three classes: Tower, Disk and TowersOfHanoi. The class definitions are placed in the files, towers_of_hanoi.py, tower.py, and disk.py, respectively. The mainline code is in the toh_main.py file.
Main: toh_main module:
The mainline code has a main function which uses a loop to play each of the 22 games with number of disks ranging from 3 to 24. For each game a TowersOfHanoi object is created and its play method is called. In addition, the number of moves for the game is displayed at the end of each game from this main function. Please follow the sample output which shows the program output. The Tower contents are displayed only when the game uses four disks.
Disk class UML:
Disk |
-size: int |
+__init__(size: int) +get_size(): int +__str__(): string |
A Disk is the object placed on a Tower. A Disk object has a size (private instance variable), which is initialized in the constructor when a Disk object is created. The Disk is an immutable class no setters. You may also code a getter for the size, if desired. Use the special __str__ method to display the size of the Disk, when the Tower containing the Disk is displayed.
Tower class UML:
Tower(list) |
-name: string |
+__init__(name: string) +get_name(): string +move(dest_tower: Tower) +__str__(): string |
You simulate a Tower in this game by making it a list. The Tower class must inherit from the list class. So, in OOP terms, we say that a Tower is a list. The Tower class is a subclass of the list class. Conversely, the list class is a super class for the Tower class. This means that you can call any list method on a Tower object. The Tower class constructor must call the list class constructor using the super method.
The Tower is a list of Disk objects. This implies that there is an aggregation association between Towers and Disks. The Disk objects are added to and removed from the three Tower objects as the game is played. The Tower only adds Disks to the end of itself and takes them off at the same end, simulating a stack. (More on stacks in Lesson 6). So, to initialize the beginning Tower, you need to add the Disks in order from largest to smallest. The Disk sizes are numbered 1, 2, 3, ., num_of_disks. Try using the following range function: range(num_of_disks, 0, -1)
The length of the Tower is the number of disks it has on it. This can be obtained with len(self). The individual Disks can be accessed with self[index], where index is its position on the Tower (self is a list).
A Tower object has a name (private instance variable), which is initialized in the constructor when a Tower object is created. The name is either A, B, or C, which is displayed when the Tower is displayed. A Tower must have a method that can be called to move a Disk from itself to a destination Tower.
You can code a getter for the name variable, if needed.
You are required to code a move method for the Tower class. The move method pops the top Disk from itself and appends the Disk to the end of the destination Tower. See its use in the move_disk method of the TowersOfHanoi class.
You must code the special __str__ method for the Tower class. It is needed to display the Tower. See the sample output. The __str__ method returns a string for displaying a Tower as follows:
For empty Towers: Tower A: []
For Towers with Disks: Tower A: [5, 4, 1]
You must follow the format of the output given above. There are many ways to create a string object from the Tower list for display. The simplest way to display the Tower list (which has Disk objects) is to use the join method of the string class. The Python string join method takes as an argument a list or another iterable. The string itself is the separator between each element of the list. You can also use the Python map function to create the list to pass in to the join method. The map function applies a passed in function object to each item of an iterable (a list). (Function objects will be covered in Lesson 4.) The returned value from the map function can then be passed to functions like list() to create a list.
For your program, you can do the following or something like this. However, since I gave you this code, you are required to comment in your code what the lines in red are doing, if you use this code.
tower_name = Tower + self.__name + :
separator = ,
disk_list = [str(d) for d in self]
OR use
disk_list = list(map(str, self))
disk_str = separator.join(disk_list)
tower_list_str = '[' + disk_str + ]
tower_str = tower_name + tower_list_str
TowersOfHanoi class UML:
TowersOfHanoi |
-num_of_disks: int -num_of_moves: int -towers: list |
+__init__(num_disks: int) +get_num_of_moves(): int +move_disks(num_disks_to_move: int, source: Tower, helper: Tower, target: Tower) +play() +display_towers() |
The TowersOfHanoi object is the one which plays the Towers of Hanoi game. This object must have a list containing three Towers objects. The Towers only exist within this TowersOfHanoi class object. This implies that there is a composition association between the TowersOfHanoi and Tower objects. So, in OOP terms, we say that the TowersOfHanoi has a list of Towers.
The constructor for the TowersOfHanoi class must accept the number of Disks being used for the game. The constructor initializes the num_of_disks (private) instance variable with the passed in number of Disks. The num_of_moves (private) instance variable is initialized to 0 in the constructor and is updated with every move. There is a getter for this variable that must be called after every game to display the number of moves.
The towers (private) instance variable is the list of the three Tower objects. It is created in the constructor. Each of the three Tower objects are also instantiated and added to the towers list. The first Tower (A) is initialized with the complete set of Disk objects for the game.
This TowersOfHanoi class must have a recursive method to move the Disks from Tower to Tower. There must be a helper method to start the game which calls the recursive method the first time. There must be a display method to display the three Towers after every move. When playing the game with 4 disks, you must display the towers to see the movement between them. Do this only when playing with 4 disks.
The move_disks method is the recursive method. Most of the code for this method is given to you in the background information provided ealier in this document. See the toh function. The play method is the helper method that calls the recursive move_disks method to start the game. The display_towers method must display each of the three towers and produce the sample output shown below.
You may code up these classes and the main function, as you wish, but you
must follow these specifications. You must also display the Towers, when the number of disks is 4, exactly as shown below in the Required Output.
Required Output
Towers of Hanoi
===============
Moving 3 disks completed in 7 moves!
Towers:
Tower A: [4, 3, 2, 1]
Tower B: []
Tower C: []
Towers:
Tower A: [4, 3, 2]
Tower B: [1]
Tower C: []
Towers:
Tower A: [4, 3]
Tower B: [1]
Tower C: [2]
Towers:
Tower A: [4, 3]
Tower B: []
Tower C: [2, 1]
Towers:
Tower A: [4]
Tower B: [3]
Tower C: [2, 1]
Towers:
Tower A: [4, 1]
Tower B: [3]
Tower C: [2]
Towers:
Tower A: [4, 1]
Tower B: [3, 2]
Tower C: []
Towers:
Tower A: [4]
Tower B: [3, 2, 1]
Tower C: []
Towers:
Tower A: []
Tower B: [3, 2, 1]
Tower C: [4]
Towers:
Tower A: []
Tower B: [3, 2]
Tower C: [4, 1]
Towers:
Tower A: [2]
Tower B: [3]
Tower C: [4, 1]
Towers:
Tower A: [2, 1]
Tower B: [3]
Tower C: [4]
Towers:
Tower A: [2, 1]
Tower B: []
Tower C: [4, 3]
Towers:
Tower A: [2]
Tower B: [1]
Tower C: [4, 3]
Towers:
Tower A: []
Tower B: [1]
Tower C: [4, 3, 2]
Towers:
Tower A: []
Tower B: []
Tower C: [4, 3, 2, 1]
Moving 4 disks completed in 15 moves!
Moving 5 disks completed in 31 moves!
Moving 6 disks completed in 63 moves!
Moving 23 disks completed in 8388607 moves!
Moving 24 disks completed in 16777215 moves!
Step by Step Solution
There are 3 Steps involved in it
Step: 1
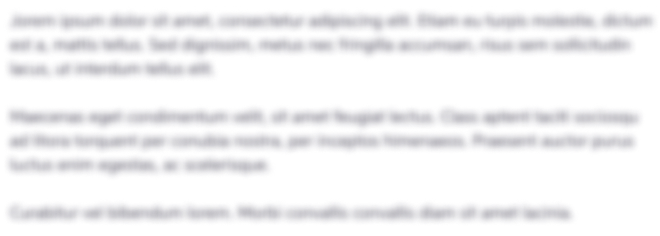
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started