Question
PYTHON Use Lists and Dictionaries to enhance and improve your Cake Program; to achieve flexibility, efficiency and readability Specifications Use the template provided to assist
PYTHON
Use Lists and Dictionaries to enhance and improve your Cake Program; to achieve flexibility, efficiency and readability
Specifications
- Use the template provided to assist you in Lab 5 Part 2(Extra Credit); either download it from the Lab 5 assignment page in Canvas and use it as your base or use what you've developed for Part 2 if you've completed it.
- Modify the program to use (3)Dictionaries to store the (3)recipes
- Replace the 3 individual functions for calculating and outputting ingredients (calc_n_output_chocolate_ingrd, calc_n_output_redvelvet_ingrd, calc_n_output_lemon_ingrd) with one fully parameterized function with cake_wt and ingredient dictionary as its parameters and return a listwhich contains all the ingredients weights for the cake specified by the recipe dictionary. No more hard-code inside the function. And, also remove the call to print_ingrd(), because it will be called from the main program. The call statement and the function header should be like this:
-
choc_ingrd_list = calc_ingrd( choc_cake_wt, choc_recipe ) # e.g. for the chocolate cake
-
def calc_ingrd( cake_wt, recipe )
-
where choc_ingrd_lst is a List and choc_recipe is a Dictionary
-
- Modify the print_ingrd() function such that it can be called from the main program, after calling calc_ingrd, and you can simply use a loop inside the function to print the ingredients. The calling statement and function header should look like this:
-
print_ingrd ( choc_ingrd_lst, ingrd_names_list ) # make this call after calling calc_ingrd(), from the main program
- def print_ingrd ( ingrd_list, ingrd_names )
- where choc_ingrd_list is what was returned from calling calc_ingrd and ingrd_names_list is a list of string literals which are the names for all the possible ingredients, e.g. ["Flour", "Sugar", ", "Unsweetened Cocoa Powder", .... ]
-
Now you have a much cleaner, efficient and flexible program providing ease of maintenance and further modification - for more cake types, changing recipes, etc.
Checkpoints:
- Use dictionaries to define recipes
- Use lists as argument, parameter and return of a function
- Build up a list by using some list methods and functions. That is the most critical part in the functioncalc_ingrd(). There could be several different ways to achieve that.
- All the Documentation Requirements stated in previous lab assignments still apply.
def print_ingrd(flour_wt, sugar_wt, ...list of all possible ingredients for all cakes...): """ This general print function has a parameter list that includes all possible ingredients, for all cakes. Print only those ingredients which are in the current cake recipe - with non-zero values. """
if flour_wt != 0: print( ... ) if sugar_wt != 0: print( ... ) if unsweetened_cocoa_powder_wt != 0: print( ... ) . . .
if ... != 0 # last ingredient print( ... )
# Test code - back calcuation to get total weight # calculate total weight here print( ... total weight ... )
def calc_n_output_chocolate_ingrd(cake_wt): # Chocolate cake recipe; in percentage of total weight CHOC_FLOUR_PCT = CHOC_SUGAR_PCT = . . .
# create and initialize all variable to zero flour_wt = 0 sugar_wt = 0 . . .
""" *************************************************** CALCULATE *************************************************** """ # calc wt for each ingredient which is in the recipe sugar_wt = ... unsweetened_cocoa_powder_wt = ... baking_powder_wt = ... . . .
""" *************************************************** OUTPUT *************************************************** """ # output ingredient list by calling print_ingrd() print_ingrd( ... complete list of all possible ingredients, including even those with zero values ... )
def calc_n_output_red_velvet_ingrd(cake_wt): . . . SIMILAR TO calc_n_output__chocolate_ingrd() . . .
def calc_n_output__lemon_ingrd(cake_wt): . . . SIMILAR TO calc_n_output__chocolate_ingrd() . . .
""" ******************************************************************************* PROGRAM STARTS HERE ******************************************************************************* """
# CONSTANTS: Cake size weights in oz LARGE_CAKE_WT = ... REGULAR_CAKE_WT = ...
# define and initialize Cake Variables for the main program choc_cake_tot_wt = #... AND 2 MORE variables for the cake sizes ...
# ... Similar variables for red velvet ...
# ... Similar variables for lemon ...
""" *********************************************************** INPUT *********************************************************** """ cake_type = input( ... ) if not( cake_type == 'q' or cake_type == 'Q' ): cake_size = input( ... )
""" ************************************************* ... sentinel while loop here ... * * . . ... One set of Cake Variables (defined above) will be updated for each round . . * * ************************************************* """
""" *********************************************************** CALCULATE & OUTPUT *********************************************************** """ # Figure out the correct words for print-out
# the printing of header could be done insider the function # too, but this is simpler implmentation print(... header for Chocolate Cake orders ... ) calc_n_output_chocolate_ingrd( choc_cake_tot_wt )
print(... header for Red Velet Cake orders...) calc_n_output_red_velet_ingrd(redv_cake_tot_wt)
print(... header for Lemon Cake orders...) calc_n_output_lemon_ingrd(lemo_cake_tot_wt)
Chocolate Cake Recipe: Flour - 15.8% Sugar - 24.5% Unsweetened Cocoa Powder - 5.6% Baking Powder - 0.4% Baking Soda - 0.6% Salt - 0.4% Egg - 9% Buttermilk - 18.0% Canola Oil - 8.1% Vanilla Extract - 0.6% Boiling Water - 17.0%
Red Velvet Cake Recipe: Flour - 22.4% Sugar - 22.4% Baking Soda - 0.7% Salt - 0.4% Unsweetened Cocoa Powder - 0.4% Canola Oil - 24.0% Buttermilk - 17.9% Egg - 9.0% Red Food Coloring - 2.1% Vanilla Extract - 0.3% Distilled Vinegar - 0.3%
Lemon Cake Recipe: Butter - 8.5% Sugar - 15.0% Egg - 9.0% Sifted Self-Rising Flour - 15.6% Buttermilk - 9.0% Vanilla Extract - 0.2% Filling: Egg Yolk - 17.9% Sugar - 11.3% Butter - 2.1% Lemon Juice and Zest - 11.4%
These are what the Cake Program will do :
Take an order for the type and size of the cake from the keyboard(console) interface. These are the types that can be ordered, only 3 types such that the programming scope is within manageable limits, as the lab assignment for this week: Chocolate Red Velvet Lemon For each type, the customer can choose from two sizes: Regular (4 Lb) Large (7 Lb) From the inputs gotten from above, using the recipes given here, calculate the amount of each ingredient required. You must use the recipe here! For the cake just selected, print the list of ingredients and each accompanied with the amount in Oz
OUTPUT:
Please Select Cake Type; Enter 1 for Chocolate, 2 for Red Velvet, 3 for Lemon, q to quit: 1 Please Select Cake Size; Enter L for large, R for regular: R
Regular Chocolate Ingredient List: Flour: 10.1 Oz sugar: 15.7 Oz Unsweetened Cocoa Powder: 3.6 Oz Baking Powder: 0.3 Oz Baking Soda: 0.4 Oz Salt: 0.3 Oz Egg: 5.8 Oz buttermilk: 11.5 Oz Canola Oil: 5.2 Oz Vanilla Extract: 0.4 Oz Boiling Water: 10.9 Oz
Step by Step Solution
There are 3 Steps involved in it
Step: 1
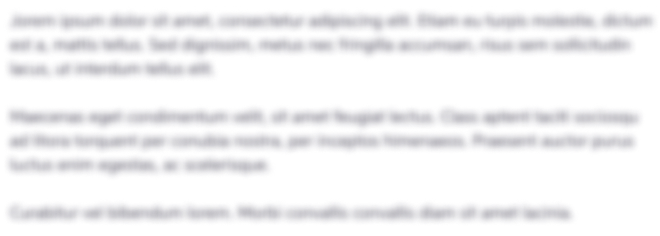
Get Instant Access to Expert-Tailored Solutions
See step-by-step solutions with expert insights and AI powered tools for academic success
Step: 2

Step: 3

Ace Your Homework with AI
Get the answers you need in no time with our AI-driven, step-by-step assistance
Get Started